Java Reference
In-Depth Information
Code 12.23
continued
Reading integer data
with
Scanner
public
int
[] readInts(String filename)
{
int
[] data;
try
{
List<Integer> values =
new
ArrayList<Integer>();
Scanner scanner =
new
Scanner(
new
File(filename));
while
(scanner.hasNextInt()) {
values.add(scanner.nextInt());
}
// Copy them to an array of the exact size.
data =
new
int
[values.size()];
Iterator<Integer> it = values.iterator();
int
i = 0;
while
(it.hasNext()) {
data[i] = it.next();
i++;
}
}
catch
(FileNotFoundException e) {
System.out.println(
"Cannot find file: "
+ filename);
data =
new
int
[0];
}
return
data;
}
Note that this method does not guarantee to read the whole file. The
Scanner
's
hasNextInt
method that controls the loop will return
false
if it encounters text in the file that does not
appear to be part of an integer. At that point, the data gathering will be terminated. In fact, it
is perfectly possible to mix calls to the different
next
methods when parsing a complete file,
where the data it contains is to be interpreted as consisting of mixed types.
Another common use of
Scanner
is to read input from the “terminal” connected to a program.
We have regularly used calls to the
print
and
println
methods of
System.out
to write
text to the BlueJ terminal window.
System.out
is of type
java.io.PrintStream
and maps
to what is often called the
standard output
destination. Similarly, there is a corresponding
standard input
source available as
System.in
, which is of type
java.io.InputStream
. An
InputStream
is not normally used directly when it is necessary to read user input from the ter-
minal, because it delivers input one character at a time. Instead,
System.in
is usually passed
to the constructor of a
Scanner
. The
InputReader
class in the
tech-support-complete
project
of Chapter 5 uses this approach to read questions from the user:
Scanner reader = new Scanner(System.in);
. . . intervening code omitted . . .
String inputLine = reader.nextLine();
The
nextLine
method of
Scanner
returns the next complete line of input from standard input
(without including the final newline character).
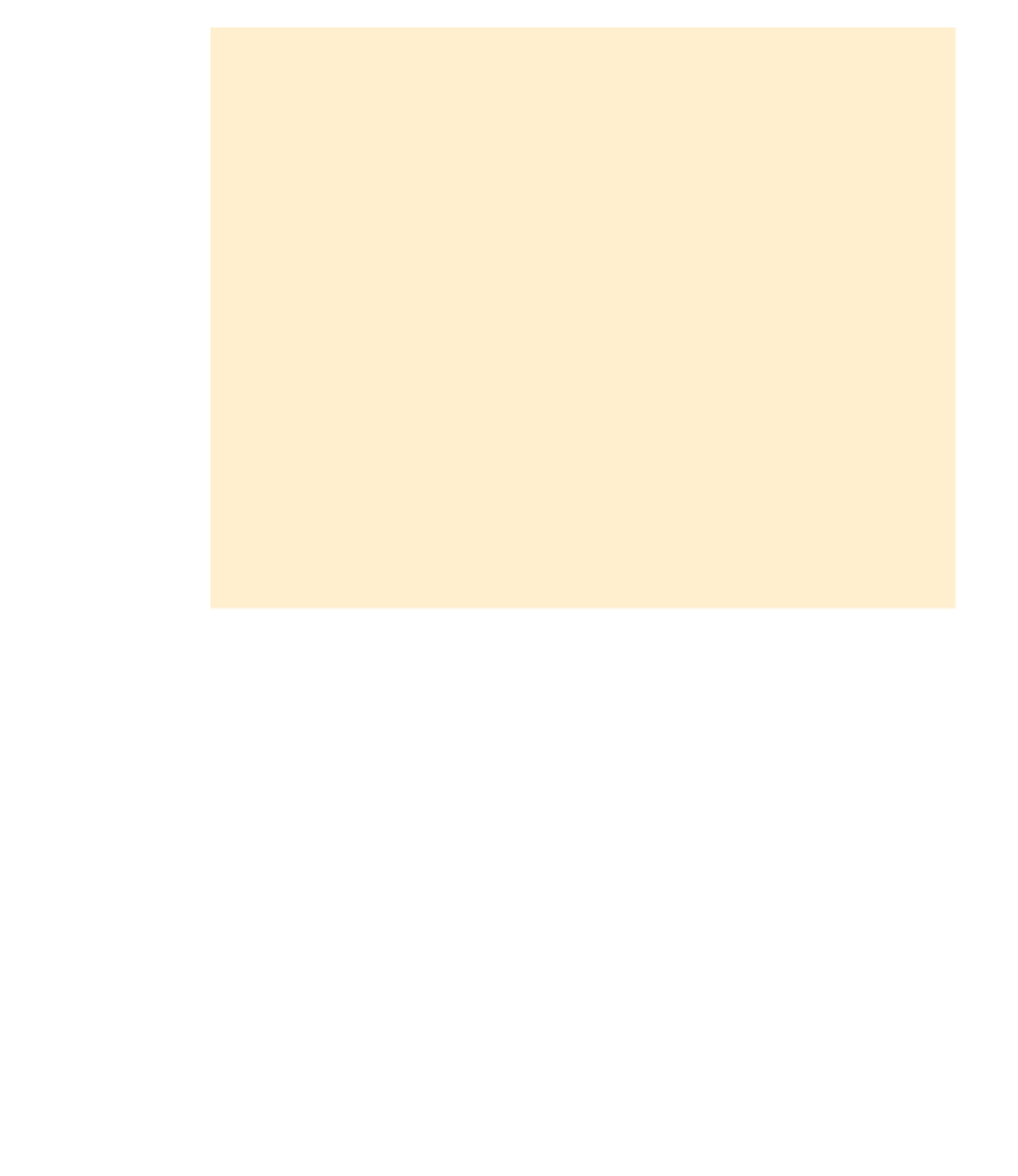
Search WWH ::

Custom Search