Java Reference
In-Depth Information
An exception handler must cater for all checked exceptions thrown from its protected state-
ments, so a try statement may contain multiple catch blocks, as shown in Code 12.11. Note that
the same variable name can be used for the exception object in each case.
Code 12.11
Multiple catch blocks
in a try statement
try
{
...
ref.process();
...
}
catch
(EOFException e) {
// Take action appropriate to an end-of-file exception.
...
}
catch
(FileNotFoundException e) {
// Take action appropriate to a file-not-found exception.
...
}
When an exception is thrown by a method call in a try block, the catch blocks are checked
in the order in which they are written until a match is found for the exception type. So, if
an
EOFException
is thrown, then control will transfer to the first catch block, and if a
FileNotFoundException
is thrown, then control will transfer to the second. Once the end of
a single catch block is reached, execution continues following the last catch block.
Polymorphism can be used to avoid writing multiple catch blocks, if desired. However, this
could be at the expense of being able to take type-specific recovery actions. In Code 12.12,
the single catch block will handle
every
exception thrown by the protected statements. This
is because the exception-matching process that looks for an appropriate catch block simply
checks that the exception object is an instance of the type named in the block. As all exceptions
are subtypes of the
Exception
class, the single block will catch everything, whether checked
or unchecked. From the nature of the matching process, it follows that the order of catch blocks
in a single try statement matters and that a catch block for one exception type cannot follow a
block for one of its supertypes (because the earlier supertype block will always match before
the subtype block is checked). The compiler will report this as an error.
Code 12.12
Catching all exceptions
in a single catch block
try
{
...
ref.process();
...
}
catch
(Exception e) {
// Take action appropriate to all exceptions.
...
}
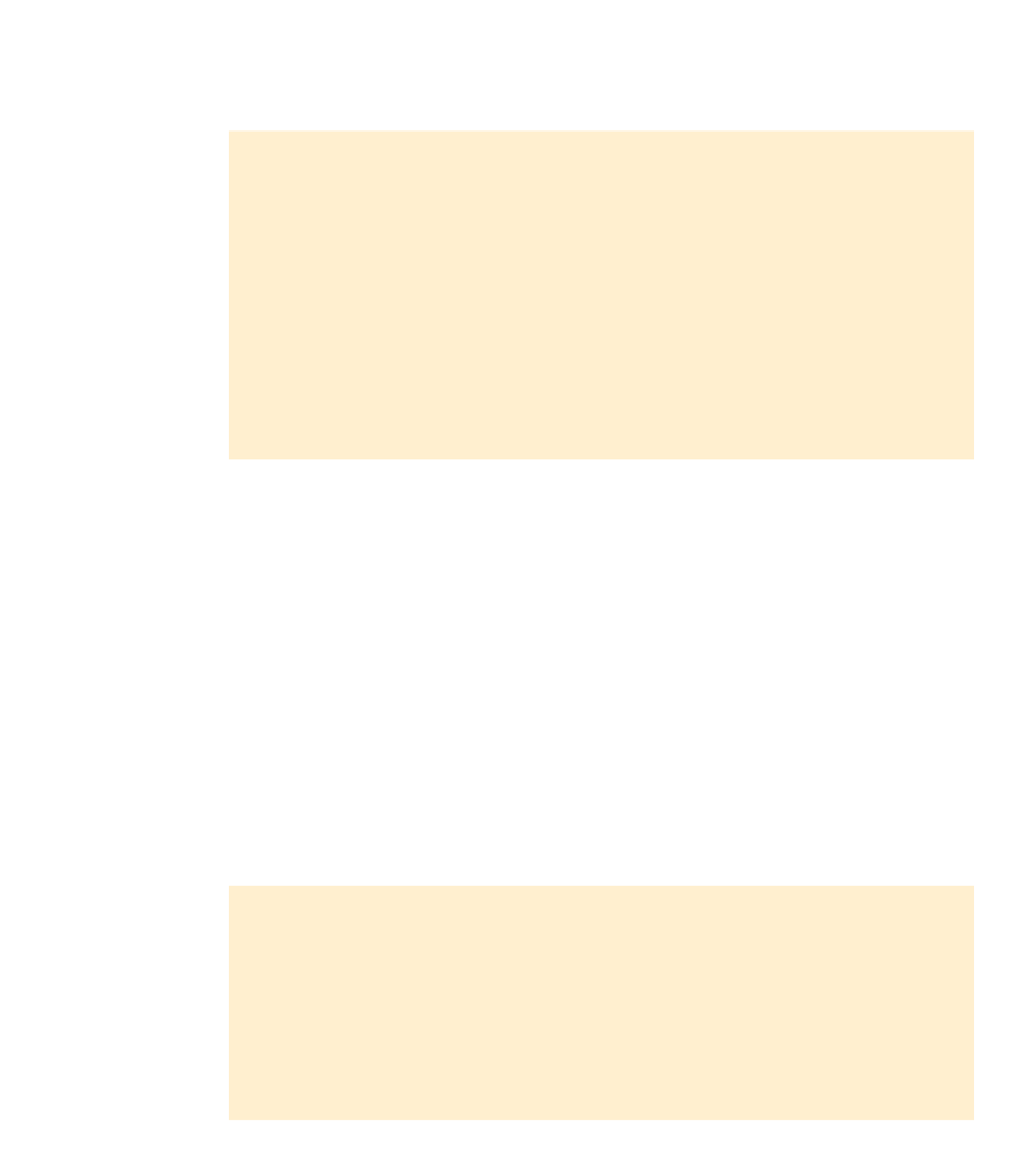
Search WWH ::

Custom Search