Java Reference
In-Depth Information
Dialogs can be implemented in a similar way to our main
JFrame
. They often use the class
JDialog
to display the frame.
For modal dialogs with a standard structure, however, there are some convenience methods in
class
JOptionPane
that make it very easy to show such dialogs.
JOptionPane
has, among
other things, static methods to show three types of standard dialog. They are:
■
Message dialog:
This is a dialog that displays a message and has an
OK
button to close the
dialog.
■
Confirm dialog:
This dialog usually asks a question and has buttons for the user to make a
selection—for example,
Ye s
,
No
, and
Cancel
.
■
Input dialog:
This dialog includes a prompt and a text field for the user to enter some text.
Our “About” box is a simple message dialog. Looking through the
JOptionPane
documenta-
tion, we find that there are static methods named
showMessageDialog
to do this.
Exercise 11.32
Find the documentation for
showMessageDialog
. How many methods
with this name are there? What are the differences between them? Which one should we use
for the “About” box? Why?
Exercise 11.33
Implement the
showAbout
method in your
ImageViewer
class, using a
call to a
showMessageDialog
method.
Exercise 11.34
The
showInputDialog
methods of
JOptionPane
allow a user to be
prompted for input via a dialog when required. On the other hand, the
JTextField
compo-
nent allows a permanent text input area to be displayed within a GUI. Find the documentation
for this class. What input causes an
ActionListener
associated with a
JTextField
to be
notified? Can a user be prevented from editing the text in the field? Is it possible for a listener
to be notified of arbitrary changes to the text in the field? (
Hint
: What use does a
JTextField
make of a
Document
object?)
You can find an example of a
JTextField
in the
calculator
project in Chapter 7.
After studying the documentation, we can now implement our “About” box by making a call to
the
showMessageDialog
method. The code is shown in Code 11.9. Note that we have intro-
duced a string constant named
VERSION
to hold the current version number.
Code 11.9
Displaying a modal
dialog
private
void
showAbout()
{
JOptionPane.showMessageDialog(frame,
"ImageViewer\n"
+ VERSION,
"About ImageViewer"
,
JOptionPane.INFORMATION_MESSAGE);
}
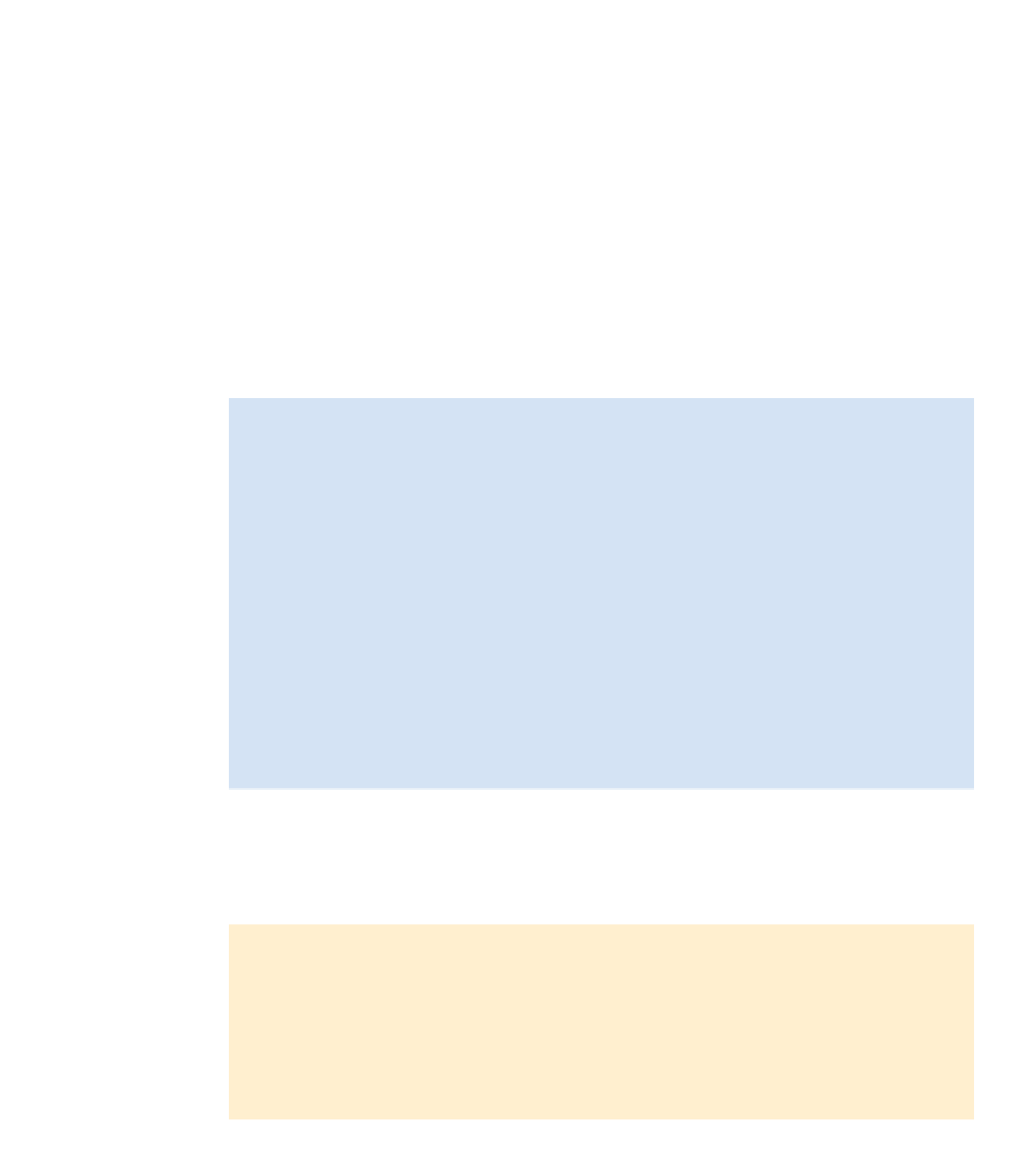
Search WWH ::

Custom Search