Java Reference
In-Depth Information
more specific subclass. These kinds of classes, which are not intended for creating objects but
serve only as superclasses, are known as
abstract classes.
For animals, for example, we can say
that each animal can act, but we cannot describe exactly how it acts without referring to a more
specific subclass. This is typical for abstract classes, and it is reflected in Java constructs.
For the
Animal
class, we wish to state that each animal has an
act
method, but we cannot give
a reasonable implementation in class
Animal
. The solution in Java is to declare the method
abstract.
Here is an example of an abstract
act
method:
Concept:
An
abstract
method
definition
consists of a
method header
without a method
body. It is marked
with the keyword
abstract
.
abstract public void act(List<Animal> newAnimals);
An abstract method is characterized by two details:
■
It is prefixed with the keyword
abstract
.
■
It does not have a method body. Instead, its header is terminated with a semicolon.
Because the method has no body, it can never be executed. But we have already established that
we do not want to execute an
Animal
's
act
method, so that is not a problem.
Before we investigate in detail the effects of using an abstract method, we shall introduce more
formally the concept of an abstract class.
10.3.3 Abstract classes
It is not only methods that can be declared abstract; classes can be declared abstract as well.
Code 10.7 shows an example of class
Animal
as an abstract class. Classes are declared abstract
by inserting the keyword
abstract
into the class header.
Concept:
An
abstract class
is a class that is not
intended for creating
instances. Its pur-
pose is to serve as a
superclass for other
classes. Abstract
classes may contain
abstract methods.
Classes that are not abstract (all classes we have seen previously) are called
concrete classes.
Declaring a class abstract serves several purposes:
■
No instances can be created of abstract classes. Trying to use the
new
keyword with an
abstract class is an error and will not be permitted by the compiler. This is mirrored in BlueJ:
right-clicking on an abstract class in the class diagram will not list any constructors in the
Code 10.7
Animal
as an abstract
class
public abstract
class
Animal
{
//
fields omitted
/**
* Make this animal act - that is, make it do whatever
* it wants/needs to do.
* @param newAnimals A list to return newly born animals.
*/
abstract public
void
act(List<Animal> newAnimals);
//
other methods omitted
}
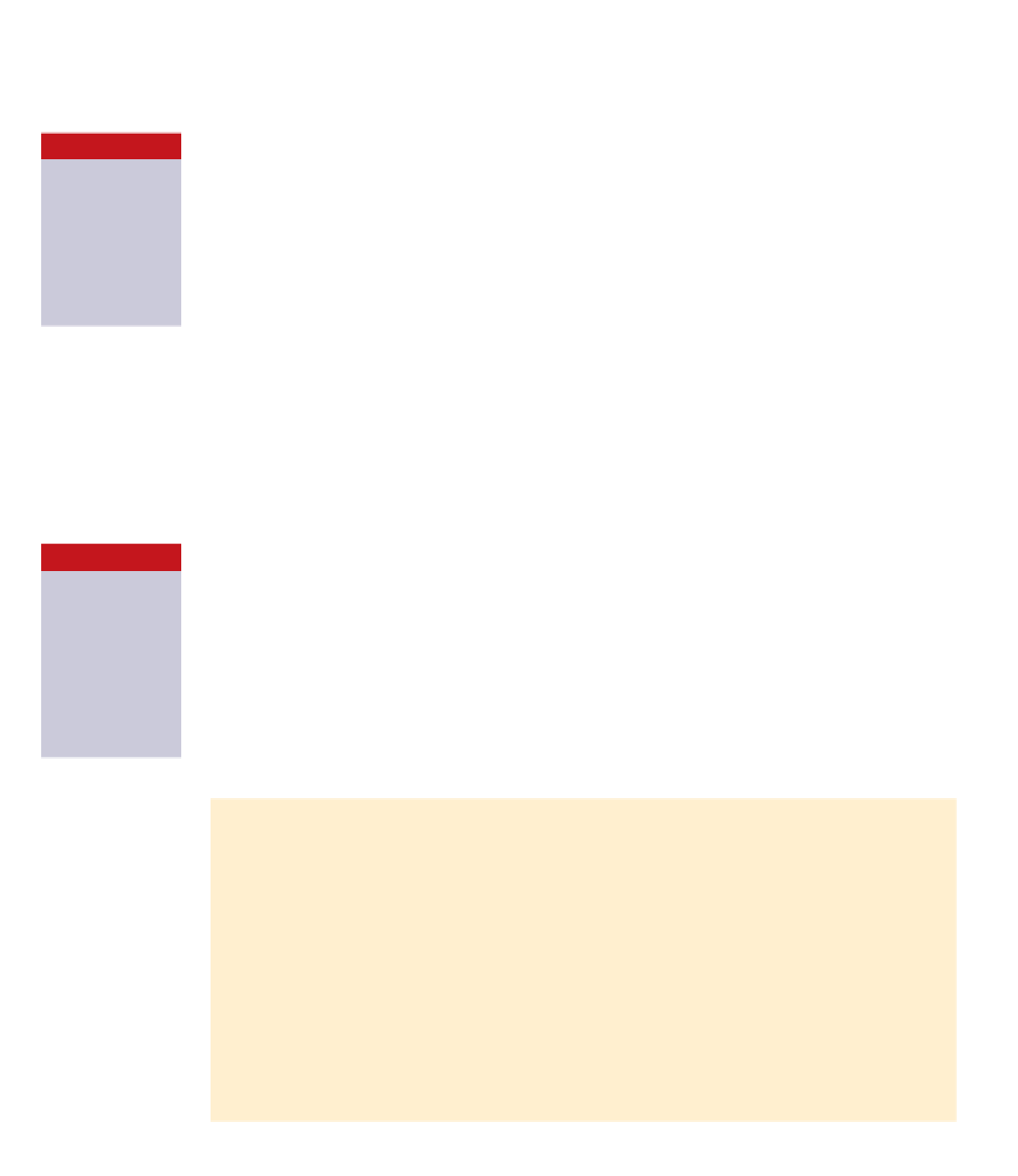
Search WWH ::

Custom Search