Java Reference
In-Depth Information
Code 10.1
continued
The
Rabbit
class
/**
* Increase the age.
* This could result in the rabbit's death.
*/
private
void
incrementAge()
{
age++;
if
(age > MAX_AGE) {
setDead();
}
}
/**
* Check whether or not this rabbit is to give birth at this step.
* New births will be made into free adjacent locations.
* @param newRabbits A list to return newly born rabbits.
*/
private
void
giveBirth(List<Rabbit> newRabbits)
{
// New rabbits are born into adjacent locations.
// Get a list of adjacent free locations.
List<Location> free = field.getFreeAdjacentLocations(location);
int
births = breed();
for
(
int
b = 0; b < births && free.size() > 0; b++) {
Location loc = free.remove(0);
Rabbit young =
new
Rabbit(false, field, loc);
newRabbits.add(young);
}
}
/**
* Generate a number representing the number of births,
* if it can breed.
* @return The number of births (may be zero).
*/
private
int
breed()
{
int
births = 0;
if
(canBreed() && rand.nextDouble() <= BREEDING_PROBABILITY) {
births = rand.nextInt(MAX_LITTER_SIZE) + 1;
}
return
births;
}
//
other methods omitted
}
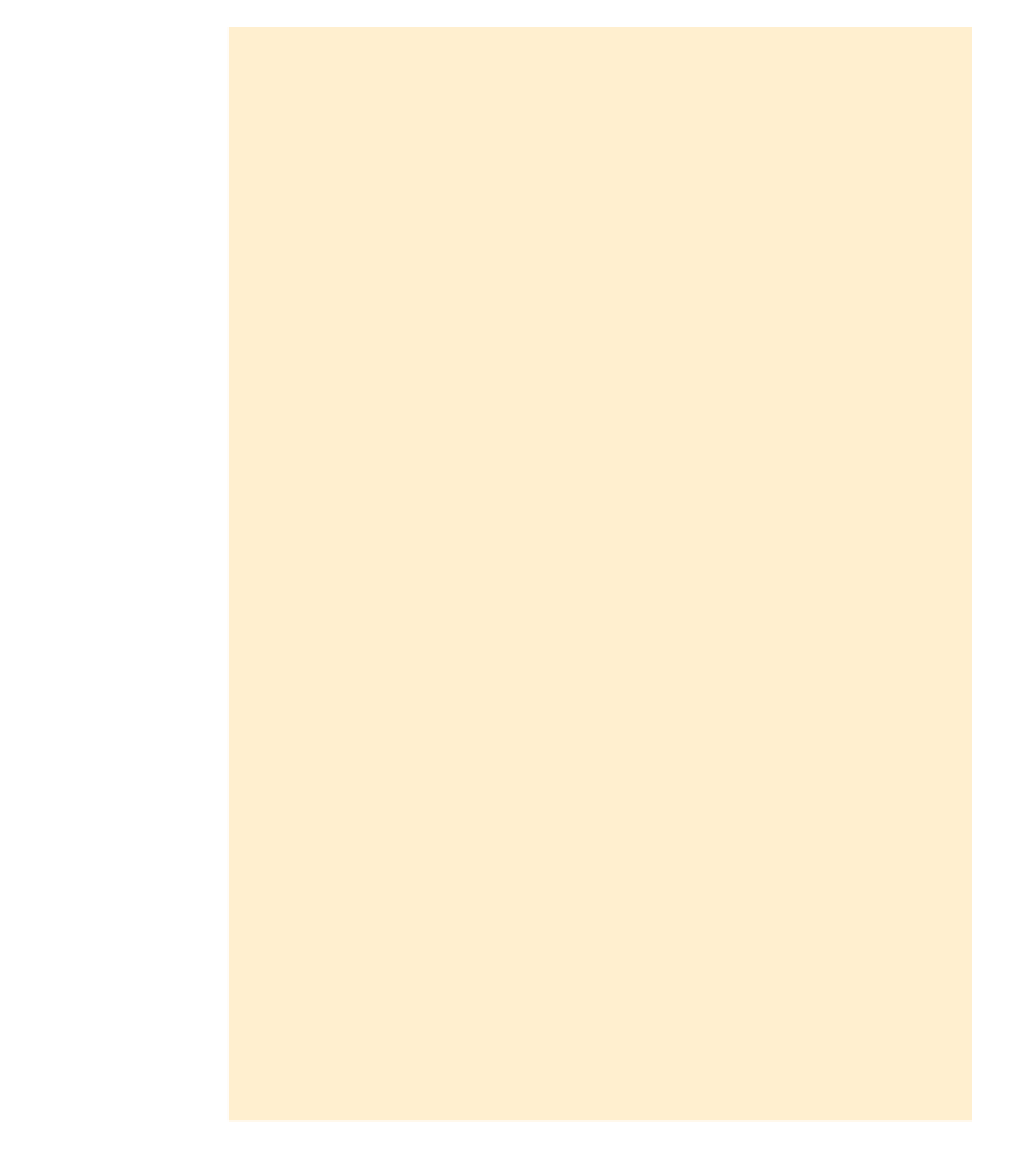
Search WWH ::

Custom Search