Java Reference
In-Depth Information
When implementing overriding methods, the
super
keyword can be used to invoke the super-
class version of the method. If fields or methods are declared with the
protected
access modi-
fier, subclasses are allowed to access them, but other classes are not.
Terms introduced in this chapter
static type, dynamic type, overriding, redefinition, method lookup, method
dispatch, method polymorphism, protected
Concept summary
■
static type
The static type of a variable
v
is the type as declared in the source code in the
variable declaration statement.
■
dynamic type
The dynamic type of a variable
v
is the type of the object that is currently stored in
v
.
■
overriding
A subclass can override a method implementation. To do this, the subclass de-
clares a method with the same signature as the superclass, but with a different method body.
The overriding method takes precedence for method calls on subclass objects.
■
method polymorphism
Method calls in Java are polymorphic. The same method call may at
different times invoke different methods, depending on the dynamic type of the variable used
to make that call.
■
toString
Every object in Java has a
toString
method that can be used to return a string
representation of itself. Typically, to make it useful, a class should override this method.
■
protected
Declaring a field or a method
protected
allows direct access to it from (direct or
indirect) subclasses.
Exercise 9.11
Assume that you see the following lines of code:
Device dev = new Printer();
dev.getName();
Printer
is a subclass of
Device
. Which of these classes must have a definition of method
getName
for this code to compile?
Exercise 9.12
In the same situation as in the previous exercise, if both classes have an
implementation of
getName
, which one will be executed?
Exercise 9.13
Assume that you write a class
Student
that does not have a declared su-
perclass. You do not write a
toString
method. Consider the following lines of code:
Student st = new Student();
String s = st.toString();
Will these lines compile? If so, what exactly will happen when you try to execute?
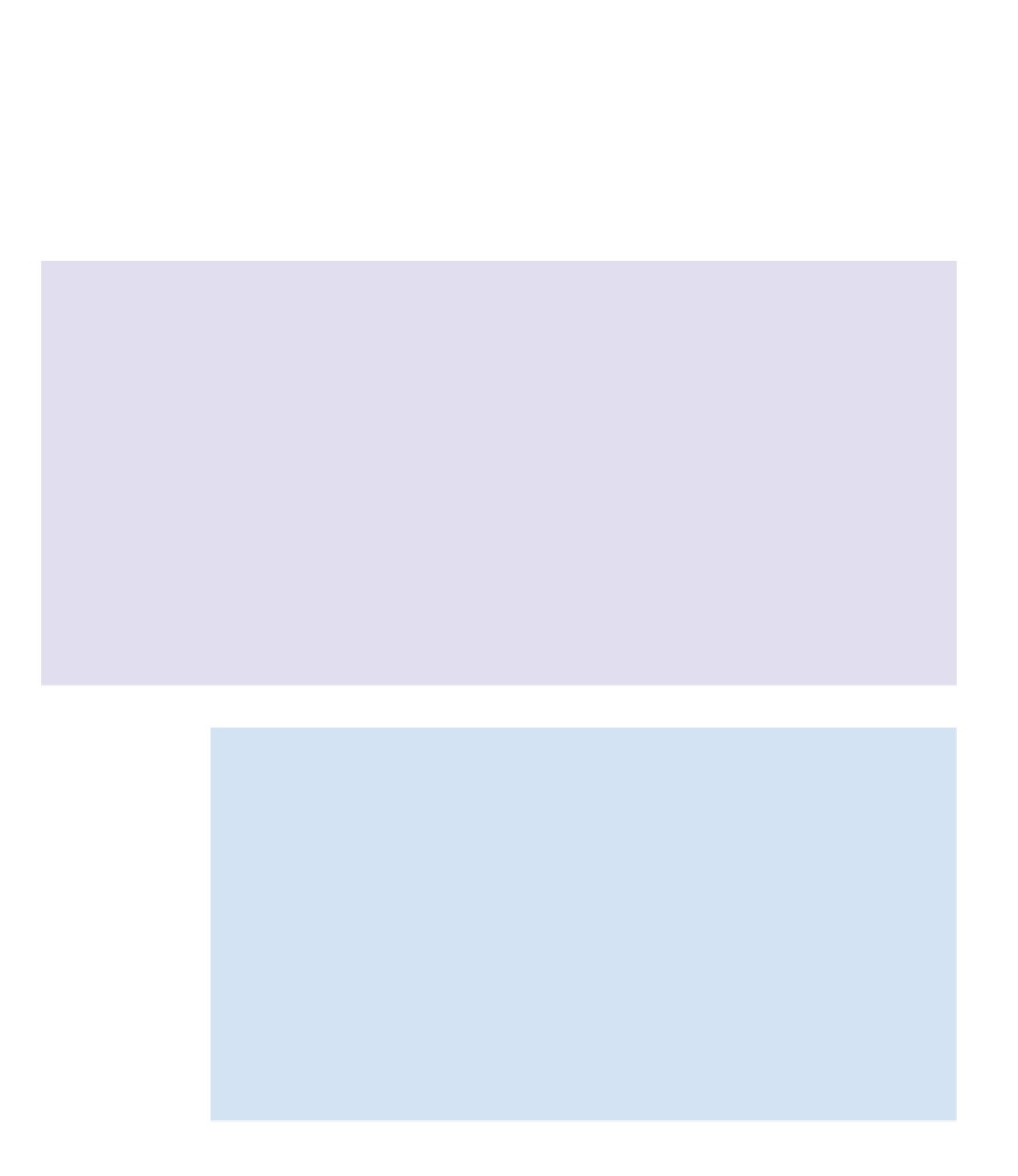
Search WWH ::

Custom Search