Java Reference
In-Depth Information
allowed if class
Post
had no
toString
method. However, the
toString
method in class
Object
guarantees that this method is always available for any class.)
■
The output appears properly with all details, because each possible dynamic type
(
MessagePost
and
PhotoPost
) overrides the
toString
method and the dynamic method
lookup ensures that the redefined method is executed.
The
toString
method is generally useful for debugging purposes. Often, it is very convenient
if objects can easily be printed out in a sensible format. Most of the Java library classes override
toString
(all collections, for instance, can be printed out like this), and often it is a good idea
to override this method for our classes as well.
9.8
Object equality:
equals
and
hashCode
It is often necessary to determine whether two objects are “the same.” The
Object
class defines
two methods,
equals
and
hashCode
, that have a close link with determining similarity. We
actually have to be careful when using phrases such as “the same”; this is because it can mean
two quite different things when talking about objects. Sometimes we wish to know whether two
different variables are referring to the same object. This is exactly what happens when an object
variable is passed as a parameter to a method: there is only one object, but both the original vari-
able and the parameter variable refer to it. The same thing happens when any object variable is as-
signed to another. These situations produce what is called
reference equality.
Reference equality is
tested for using the
==
operator. So the following test will return
true
if both
var1
and
var2
are
referring to the same object (or are both
null
), and
false
if they are referring to anything else:
var1 == var2
Reference equality takes no account at all of the
contents
of the objects referred to, just whether
there is one object referred to by two different variables or two distinct objects. That is why we also
define
content equality
, as distinct from reference equality. A test for content equality asks whether
two objects are the same internally—that is, whether the internal states of two objects are the same.
This is why we rejected using reference equality for making string comparisons in Chapter 5.
What content equality between two particular objects means is something that is defined by the
objects' class. This is where we make use of the
equals
method that every class inherits from
the
Object
superclass. If we need to define what it means for two objects to be equal accord-
ing to their internal states, then we must override the
equals
method, which then allows us to
write tests such as
var1.equals(var2)
This is because the
equals
method inherited from the
Object
class actually makes a test for
reference equality. It looks something like this:
public boolean equals(Object obj)
{
return this == obj;
}
Because the
Object
class has no fields, there is no state to compare, and this method obviously
cannot anticipate fields that might be present in subclasses.
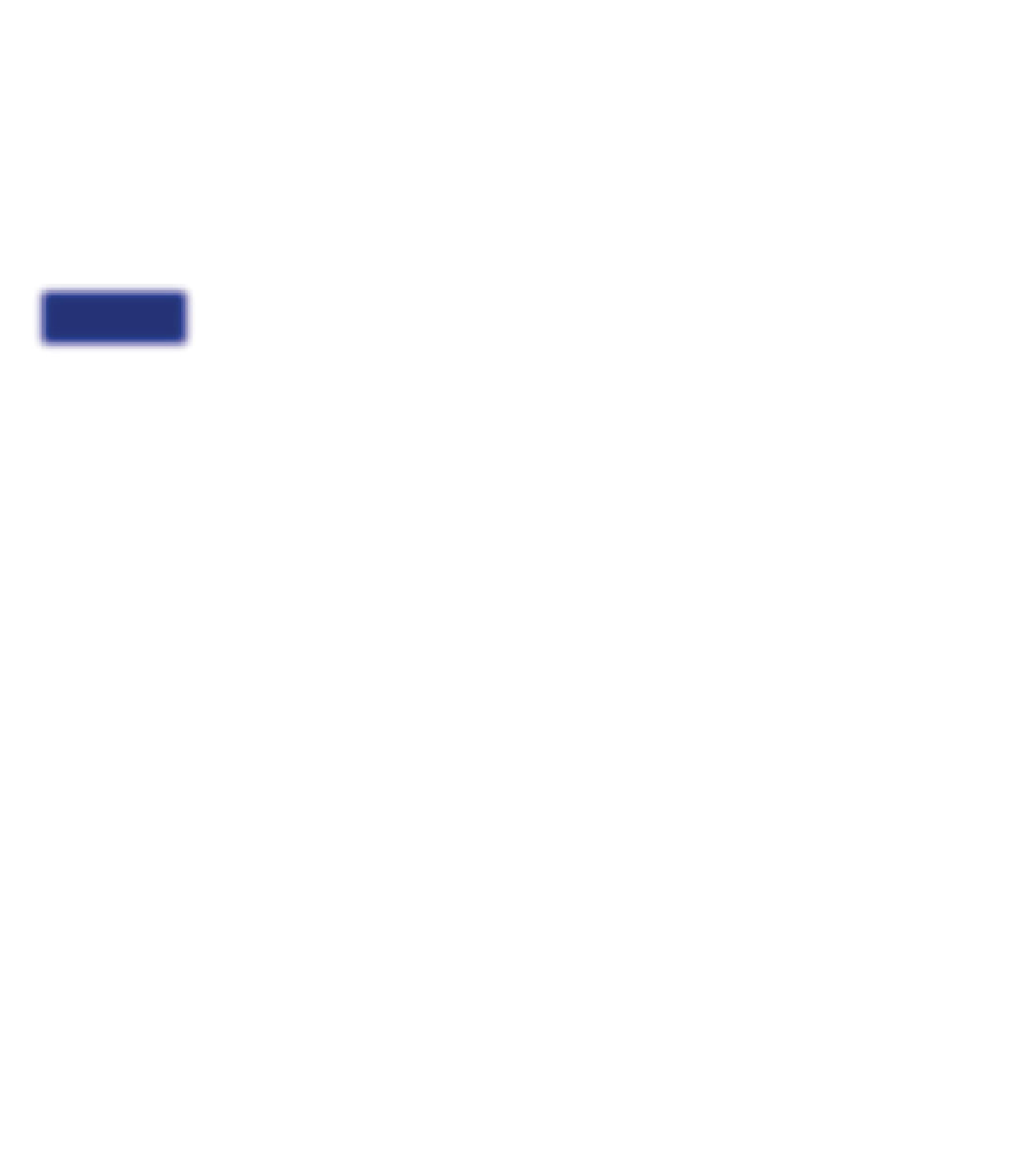
Search WWH ::

Custom Search