Java Reference
In-Depth Information
Several observations can be made here. First, the class
Post
has a constructor, even though
we do not intend to create an instance of class
Post
directly.
2
This constructor receives the
parameters needed to initialize the
Post
fields, and it contains the code to do this initialization.
Second, the
MessagePost
constructor receives parameters needed to initialize both
Post
and
MessagePost
fields. It then contains the following line of code:
super(author);
The keyword
super
is a call from the subclass constructor to the constructor of the superclass.
Its effect is that the
Post
constructor is executed as part of the
MessagePost
constructor's
execution. When we create a message post, the
MessagePost
constructor is called, which, in
turn, as its first statement, calls the
Post
constructor. The
Post
constructor initializes the post's
fields, and then returns to the
MessagePost
constructor, which initializes the remaining field
defined in the
MessagePost
class. For this to work, those parameters needed for the initializa-
tion of the post fields are passed on to the superclass constructor as parameters to the
super
call.
In Java, a subclass constructor must always call the
superclass constructor
as its first statement.
If you do not write a call to a superclass constructor, the Java compiler will insert a superclass
call automatically, to ensure that the superclass fields get properly initialized. The inserted call
is equivalent to writing
Concept:
Superclass
constructor
The
constructor of a
subclass must
always invoke the
constructor of its
superclass as its
first statement. If
the source code
does not include
such a call, Java
will attempt to insert
a call automatically.
super();
Inserting this call automatically works only if the superclass has a constructor without param-
eters (because the compiler cannot guess what parameter values should be passed). Otherwise,
an error will be reported.
In general, it is a good idea to always include explicit superclass calls in your constructors, even
if it is one that the compiler could generate automatically. We consider this good style, because
it avoids the possibility of misinterpretation and confusion in case a reader is not aware of the
automatic code generation.
Exercise 8.7
Set a breakpoint in the first line of the
MessagePost
class's constructor. Then
create a
MessagePost
object. When the debugger window pops up, use
Step Into
to step
through the code. Observe the instance fields and their initialization. Describe your observations.
8.5
Network
: adding other post types
Now that we have our inheritance hierarchy set up for the
network
project so that the common
elements of the items are in the
Post
class, it becomes a lot easier to add other types of posts.
For instance, we might want to add event posts, which consist of a description of a standard event
(e.g., “Fred has joined the 'Neal Stephenson fans' group.”). Standard events might be a user join-
ing a group, a user becoming friends with another, or a user changing their profile picture. To
2
Currently, there is nothing actually preventing us from creating a
Post
object, although that was not
our intention when we designed these classes. In Chapter 10, we shall see some techniques that allow
us to make sure that
Post
objects cannot be created directly, but only
MessagePost
or
PhotoPost
objects.
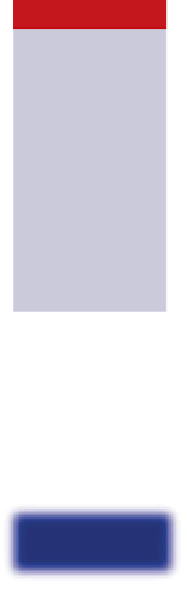

Search WWH ::

Custom Search