Java Reference
In-Depth Information
8.4.1
Inheritance and access rights
To objects of other classes,
MessagePost
or
PhotoPost
objects appear just like all other
types of objects. As a consequence, members defined as
public
in either the superclass or sub-
class portions will be accessible to objects of other classes, but members defined as
private
will be inaccessible.
In fact, the rule on privacy also applies between a subclass and its superclass: a subclass
cannot access private members of its superclass. It follows that if a subclass method
needed to access or change private fields in its superclass, then the superclass would
need to provide appropriate accessor and/or mutator methods. However, an object of a
subclass may call any public methods defined in its superclass as if they were defined lo-
cally in the subclass—no variable is needed, because the methods are all part of the same
object.
This issue of access rights between super- and subclasses is one we will discuss further in
Chapter 9 when we introduce the
protected
modifier.
Exercise 8.4
Open the project
network-v2.
This project contains a version of the
network
application, rewritten to use inheritance, as described above. Note that the class diagram
displays the inheritance relationship. Open the source code of the
MessagePost
class and
remove the “
extends Post
” phrase. Close the editor. What changes do you observe in the
class diagram? Add the “
extends Post
” phrase again.
Exercise 8.5
Create a
MessagePost
object. Call some of its methods. Can you call the
inherited methods (for example,
addComment
)? What do you observe about the inherited
methods?
Exercise 8.6
In order to illustrate that a subclass can access non-private elements of
its superclass without any special syntax, try the following slightly artificial modification to
the
MessagePost
and
Post
classes. Create a method called
printShortSummary
in
the
MessagePost
class. Its task is to print just the phrase “Message post from
NAME
”,
where
NAME
should show the name of the author. However, because the
username
field
is private in the
Post
class, it will be necessary to add a public
getUserName
method
to
Post
. Call this method from
printShortSummary
to access the name for printing.
Remember that no special syntax is required when a subclass calls a superclass method.
Try out your solution by creating a
MessagePost
object. Implement a similar method in the
PhotoPost
class.
8.4.2
Inheritance and initialization
When we create an object, the constructor of that object takes care of initializing all object
fields to some reasonable state. We have to look more closely at how this is done in classes that
inherit from other classes.
When we create a
MessagePost
object, we pass two parameters to the message post's con-
structor: the name of the author and the message text. One of these contains a value for a field
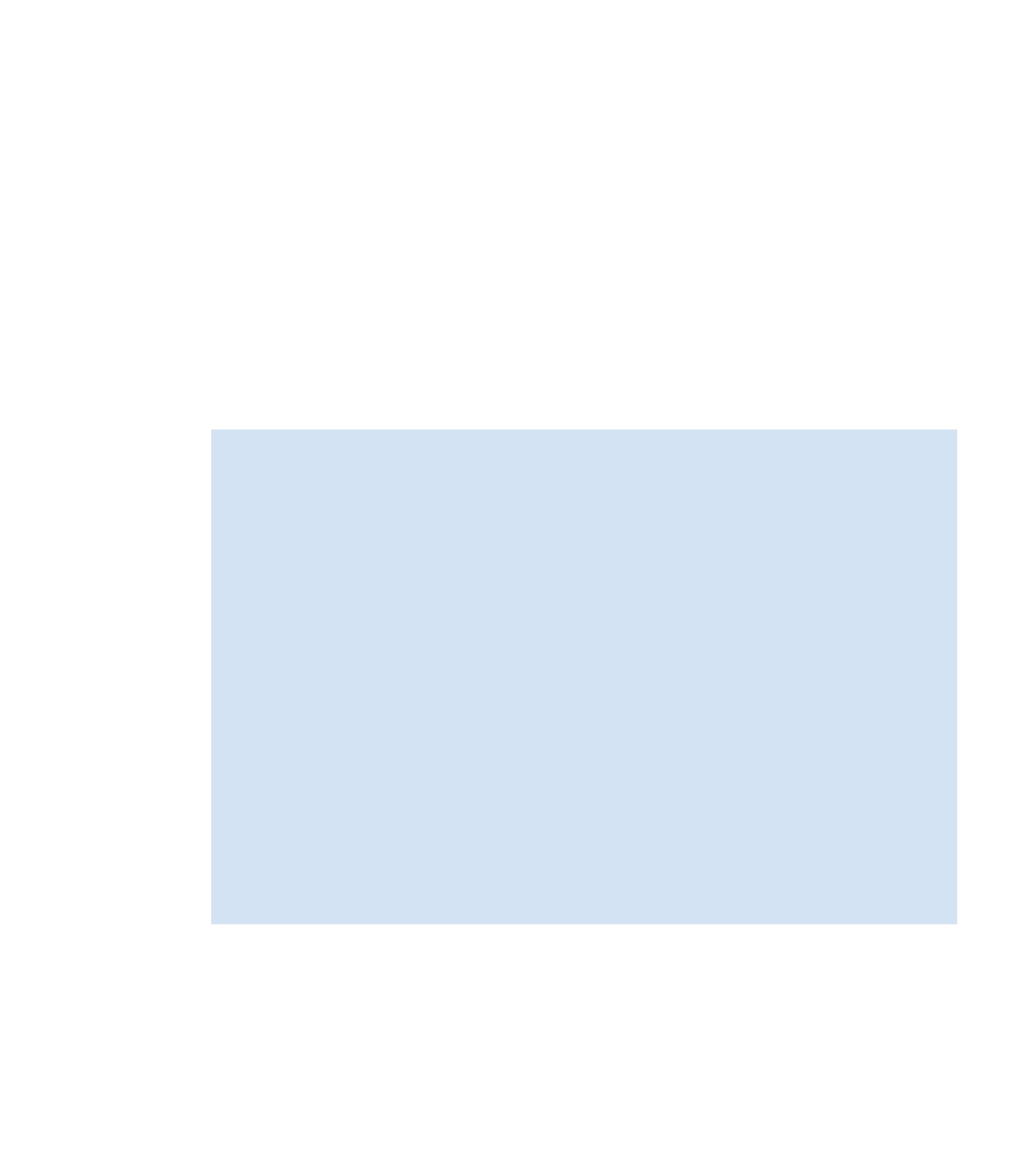
Search WWH ::

Custom Search