Java Reference
In-Depth Information
5.12.3 The
bouncing-balls
demo
Open the
bouncing-balls
project and find out what it does. Create a
BallDemo
object and ex-
ecute its
bounce
method.
Exercise 5.62
Change the method
bounce
in class
BallDemo
to let the user choose how
many balls should be bouncing.
For this exercise, you should use a collection to store the balls. This way, the method can deal
with 1, 3, or 75 balls—any number you want. The balls should initially be placed in a row along
the top of the canvas.
Which type of collection should you choose? So far, we have seen an
ArrayList
, a
HashMap
,
and a
HashSet
. Try the next exercises first, before you write your implementation.
Exercise 5.63
Which type of collection (
ArrayList
,
HashMap
, or
HashSet
) is most suita-
ble for storing the balls for the new
bounce
method? Discuss in writing, and justify your choice.
Exercise 5.64
Change the
bounce
method to place the balls randomly anywhere in the top
half of the screen.
Exercise 5.65
Write a new method named
boxBounce
. This method draws a rectan-
gle (the “box”) on screen and one or more balls inside the box. For the balls, do not use
BouncingBall
, but create a new class
BoxBall
that moves around inside the box, bounc-
ing off the walls of the box so that the ball always stays inside. The initial position and speed of
the ball should be random. The
boxBounce
method should have a parameter that specifies
how many balls are in the box.
Exercise 5.66
Give the balls in
boxBounce
random colors.
5.13
Class variables and constants
So far, we have not looked at the
BouncingBall
class. If you are interested in really under-
standing how this animation works, you may want to study this class as well. It is reasonably
simple. The only method that takes some effort to understand is
move
, where the ball changes
its position to the next position in its path.
We shall leave it largely to the reader to study this method, except for one detail that we want to
discuss here. We start with an exercise.
Exercise 5.67
In class
BouncingBall
, you will find a definition of gravity (a simple inte-
ger). Increase or decrease the gravity value; compile and run the bouncing ball demo again. Do
you observe a change?
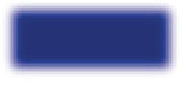
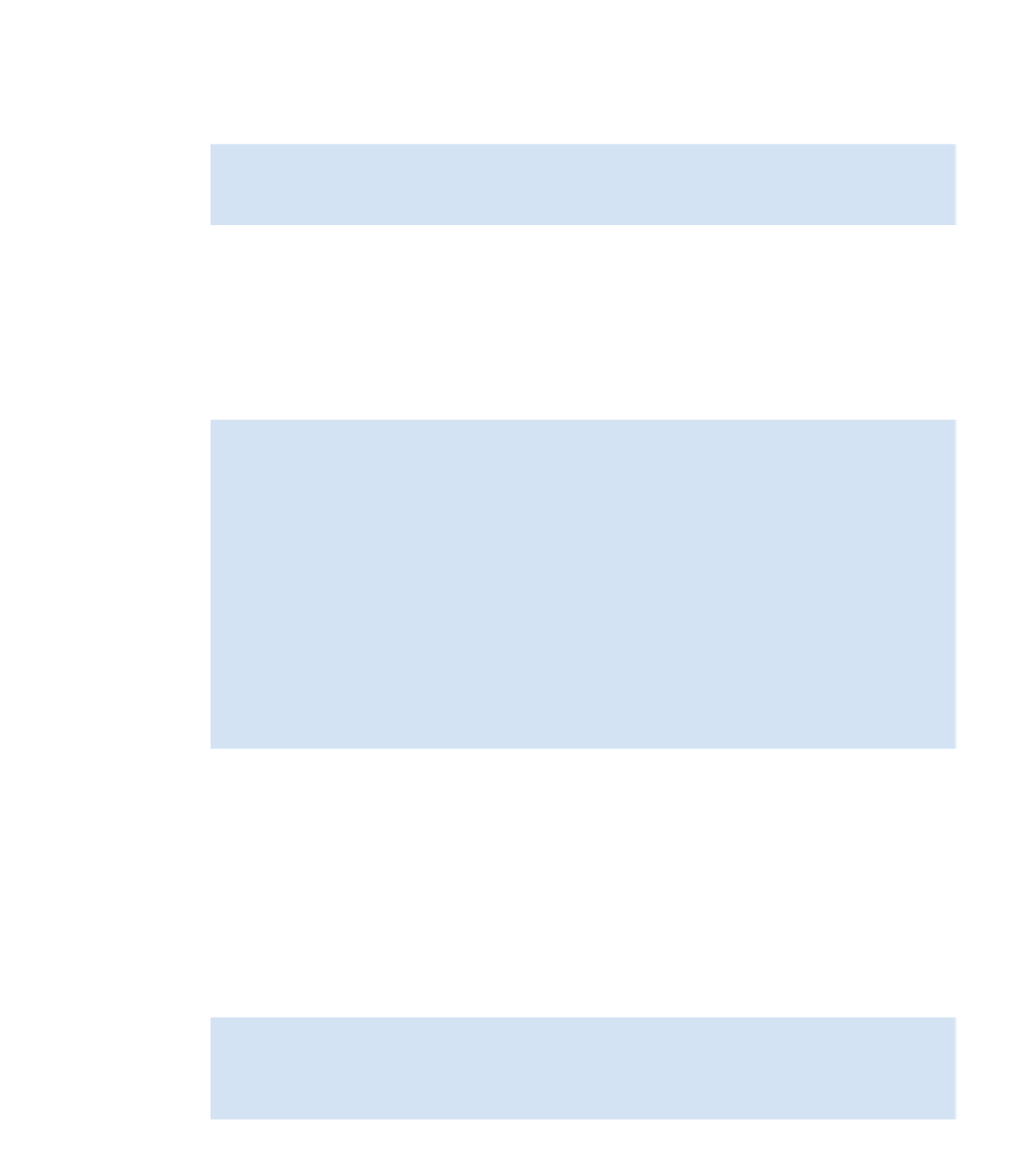
Search WWH ::

Custom Search