Java Reference
In-Depth Information
To generate a random number, we have to:
■
create an instance of class
Random
and
■
make a call to a method of that instance to get a number.
Looking at the documentation, we see that there are various methods called
next
Something
for
generating random values of various types. The one that generates a random integer number is
called
nextInt
.
The following illustrates the code needed to generate and print a random integer number:
Random randomGenerator;
randomGenerator = new Random();
int index = randomGenerator.nextInt();
System.out.println(index);
This code fragment creates a new instance of the
Random
class and stores it in the
randomGen-
erator
variable. It then calls the
nextInt
method to receive a random number, stores it in the
index variable, and eventually prints it out.
Exercise 5.14
Write some code (in BlueJ) to test the generation of random numbers. To do
this, create a new class called
RandomTester
. You can create this class in the
tech-support1
project, or you can create a new project for it—it doesn't matter. In class
RandomTester
,
implement two methods:
printOneRandom
(which prints out one random number) and
printMultiRandom(int howMany)
(which has a parameter to specify how many num-
bers you want, and then prints out the appropriate number of random numbers).
Your class should create only a single instance of class
Random
(in its constructor) and store it
in a field. Do not create a new
Random
instance every time you want a new number.
5.4.2
Random numbers with limited range
The random numbers we have seen so far were generated from the whole range of Java integers
(-2147483648 to 2147483647). That is okay as an experiment, but seldom useful. More often,
we want random numbers within a given limited range.
The
Random
class also offers a method to support this. It is again called
nextInt
, but it has a
parameter to specify the range of numbers that we would like to use.
Exercise 5.15
Find the
nextInt
method in class
Random
that allows the target range of
random numbers to be specified. What are the possible random numbers that are generated
when you call this method with 100 as its parameter?
Exercise 5.16
Write a method in your
RandomTester
class called
throwDice
that re-
turns a random number between 1 and 6 (inclusive).
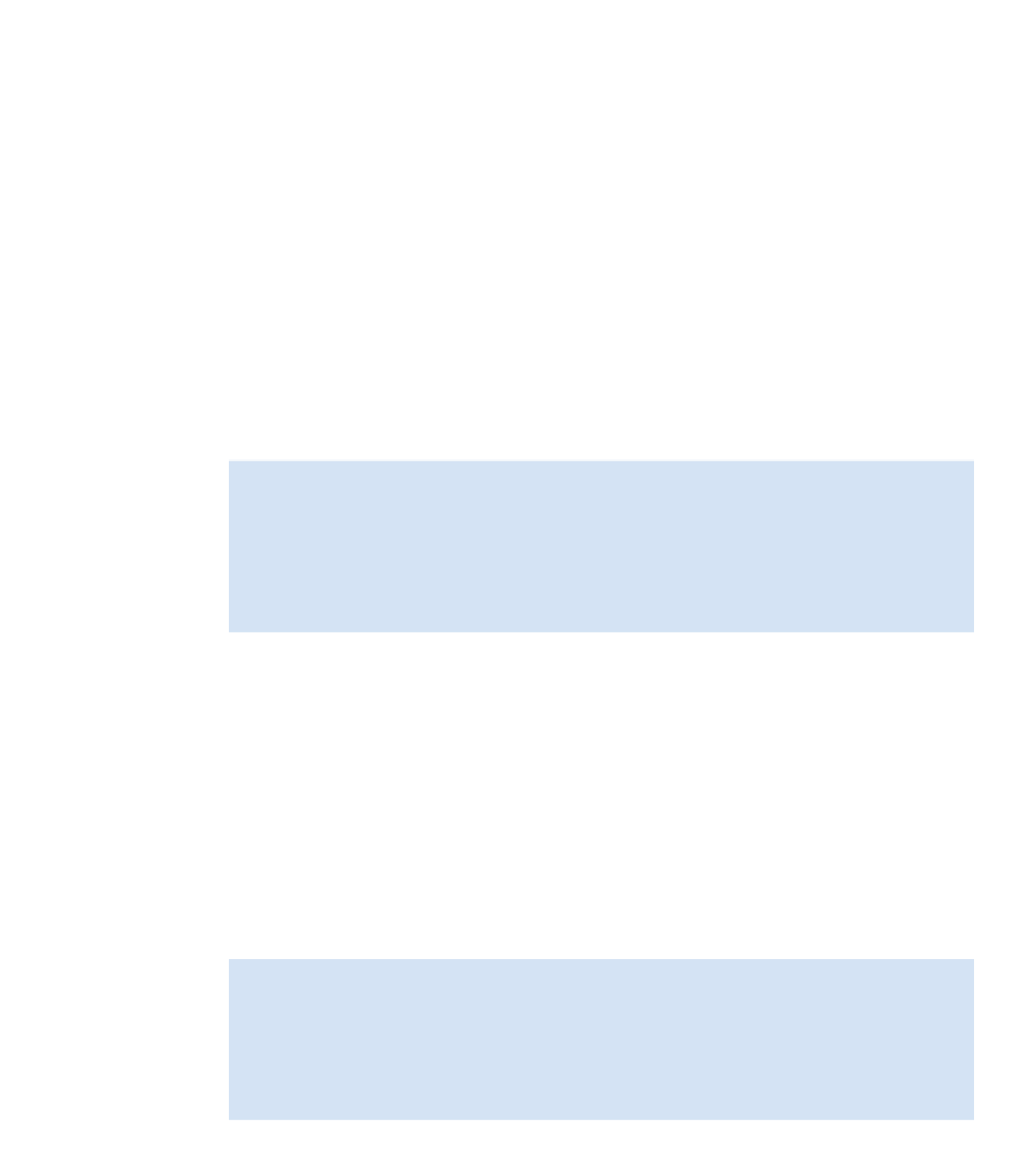
Search WWH ::

Custom Search