Java Reference
In-Depth Information
The key point here is that we have kept the references to potentially
heavyweight objects. Since we clean up in the
finally
clause, our
cleanup code is guaranteed to be executed regardless of whether the
exception is thrown.
9.2.6 Buffer I/O
Most stream implementations in Java ME are not internally buffered. This
means that reading or writing, a byte at a time, can result in extremely
slow code. Therefore, it is always preferable to read and write using
buffers.
Our first example copies from an input stream to an output stream,
known as 'piping':
public void pipe(InputStream in, OutputStream out) throws IOException
{
byte [] buffer = new byte[1024];
int read = in.read(buffer);
while ( read > 0 )
{
out.write(buffer, 0, read);
read = in.read(buffer);
}
}
The second example for buffered I/O shows how to fully read a stream
into a buffer when we know exactly how much we need to read in
advance.
public void readFully(InputStream in, byte[] buffer) throws IOException
{
int numBytes = buffer.length;
int read = 0;
while (read != numBytes )
{
int rd = in.read(buffer, read, numBytes - read);
if(rd==-1)
{
throw new IOException("End of stream!");
}
read += rd;
}
}
If the length of the stream is unknown, we can use our pipe function
with a
ByteArrayOutputStream
:
public byte[] readFully(InputStream in) throws IOException
{
ByteArrayOutputStream baos = new ByteArrayOutputStream();
pipe(in, baos);
return baos.toByteArray();
}
9.2.7 Use the Record Management System Correctly
When using the Java ME Record Management System (RMS), it is impor-
tant to consider using filters and comparators. Filters are used to determine
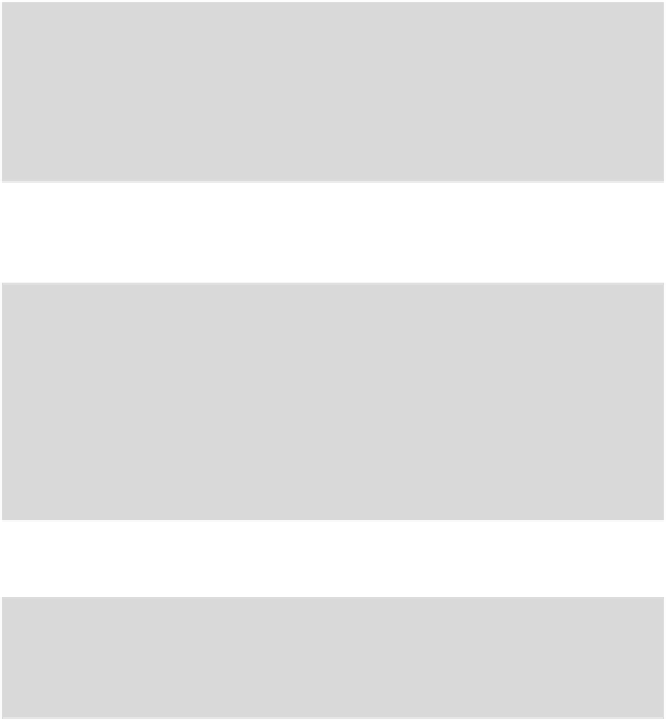