Java Reference
In-Depth Information
The array can then be initialized using the
new
operator, as follows:
weightArray = new float[5];
weightArray
The array now has space to store five floating numbers—the first num-
ber is stored at index position 0 and the last number is stored at index
position 4. The values stored initially will be the default values for the
data type, which is 0.0 for float. Figure 2-11 gives a visual representa-
tion of the array in this initialized state.
0
1
2
3
4
index
figure 2-11
You can now populate the array as follows:
weightArray[0] = 85f;
weightArray[1] = 72f;
weightArray[2] = 68f;
weightArray[3] = 94f;
weightArray[4] = 78f;
weightArray
Remember the letter
f
is added at the end of each of the numbers to
indicate that they are floating point numbers. The
weightArray
popu-
lated with the specified floats is visualized in Figure 2-12.
85
72
68
94
78
0
1
2
3
4
index
Initializing and populating the array can both be done more concisely,
as follows:
figure 2-12
float[] weightArray = {85f, 72f, 68f, 94f, 78f};
This way, the data type is given, the array is indicated with the square brackets (
[]
), the name is given
as
weightArray
, and the size is set based on the number of elements given between the brackets (
{}
).
The following Java program illustrates how arrays can be used to calculate the BMI for a set of five
people.
public class BMIcalculator {
// This is our main method.
public static void main(String[] args){
// initialize the three arrays as each having 5 elements
float[] weightArray = new float[5];
float[] heightArray = new float[5];
float[] BMIArray = new float[5];
// assign the values to the weight array
weightArray[0] = 85f;
weightArray[1] = 72f;
weightArray[2] = 68f;
weightArray[3] = 94f;
weightArray[4] = 78f;
//assign the values to the height array
heightArray[0] = 1.74f;
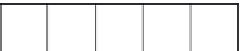
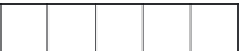
Search WWH ::

Custom Search