Java Reference
In-Depth Information
public double getAmount() {
return amount;
}
}
You can use the
Account
class as is to encapsulate the data and the behavior, which has to do with
the concept of an account. This is exactly what Object-Oriented Programming is about. Poorly
designed
singleton
classes are oftentimes “helpers” or “utility” classes that add functionality to
other classes. If possible, just move that behavior to the class itself.
NOTe
This misuse of the singleton is captured in the so‐called “god object”
anti‐pattern. Just as patterns recommend best practices, anti‐patterns indicate
commonly made mistakes and depict bad design decisions. A god object is a
centralized object that contains and does too many things. It shows up in all
other places of the codebase and becomes too tightly coupled to the other
code.
There are cases, however, where the use of singletons is helpful. A big insight lies in the fact that the
singleton pattern solves two problems at once:
➤
Limits a class to a single instance.
➤
Provides a global point of access to an instance.
In Java, you should look at both requirements separately. If you want to limit a class to a single
instance, this doesn't mean you want to allow every other object out there to access this instance. In
those cases, providing a public, global point of access is a weak architectural decision. How, then,
do you allow only one creation of a class? Like so:
public class Singleton {
private static boolean CREATED = false;
public Singleton() {
if (CREATED)
throw new RuntimeException(
"Class "+this.getClass()+" can only be created once");
CREATED = true;
}
// Other methods follow here
public static void main(String args[]) {
Singleton one = new Singleton();
Singleton two = new Singleton();
}
}
The
singleton
class can now be constructed at any time in any place, but will throw an error if
you try to construct it more than once. This code ensures single instantiation, but does not dictate
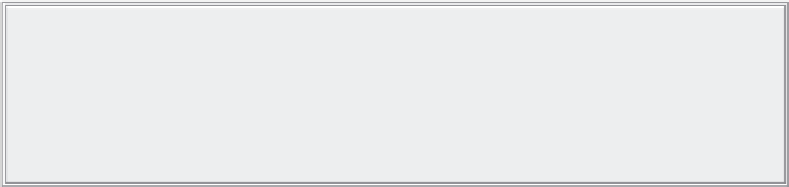
Search WWH ::

Custom Search