Java Reference
In-Depth Information
Note
By using the term event listener, you might expect the listening objects
to be the active players in this setup, but actually, it is the event source (e.g., a
button) that will notify its listeners when something interesting happens, by call-
ing one of their methods. This listener‐notifier setup is a typical programming
pattern, commonly referred to as the observer pattern. The following chapter
has much more information on Object‐Oriented Programming patterns.
Generally speaking, there are three ways you will see listener objects being defined and registered.
First of all, for simple event listeners you know you'll only need with one particular component, it is
common to define the listener directly as an anonymous inner class, like so:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
public class ActionListenerExample extends JFrame {
public ActionListenerExample() {
super();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JButton btn = new JButton("Click Me!");
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
JOptionPane.showMessageDialog(
null,
"You clicked me, nice!",
"Aw yeah!",
JOptionPane.PLAIN_MESSAGE);
}
});
this.add(btn);
pack();
setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new ActionListenerExample();
}
});
}
}
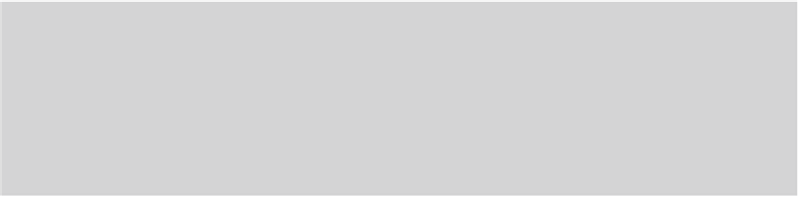
Search WWH ::

Custom Search