Java Reference
In-Depth Information
2.
Note earlier that we mentioned that you can use
InputStreamReader
and
OutputStreamReader
to create byte-to-character bridges. It is therefore, in theory, possible to write the following:
BufferedReader in = new BufferedReader(
new InputStreamReader(
new FileInputStream("groceries.txt")));
But it goes without saying that this is not an efficient nor clear manner to achieve the desired
effect. Therefore, only use
InputStreamReader
and
OutputStreamReader
when no other option
is available.
3.
The rest of the program works similarly, but now reads data line by line. Note that the newline
terminator will be stripped from the read line, so we use
System.lineSeparator()
to add a plat-
form-dependent line ending (
\r
or
\n
or
\r\n
) when writing the line to the output file. Note that
the system line separator might be different than the one used in the original file, so this implemen-
tation of our file copier might not make exact copies in this case (if you do want to create an exact
copy, you can use the
read()
method, but this will result in the code working more slowly, as now
every character is read, returned, and copied one by one). Note also that the
readLine
method
returns null when the end of a stream is reached.
Note
When not using try-with-resources blocks and closing your streams
manually, you might wonder which streams you need to close when wrapping
streams (such as is the case with buffered streams) and in which order. The
answer is simple: just close the topmost stream. The underlying ones will be
closed and tidied up as well.
data and object streams
Data streams support binary input and output of primitive data type values (boolean, char, byte,
short, int, long, float, and double) as well as String values. They thus offer a simple generic means to
load and store primitive data values. All data streams implement either the
DataInput
interface or
the
DataOutput
interface, i.e.
DataInputStream
and
DataOutputStream
. Note that these streams
subclass
InputStream
and
OutputStream
and are thus a specialization of byte streams.
Object streams are similar to data streams, but allow the serialization of all objects that imple-
ment the
Serializable
marker interface. The object streams implement either the
ObjectInput
or
ObjectOutput
interfaces (which themselves are subinterfaces of
DataInput
and
DataOutput
), i.e.
ObjectInputStream
and
ObjectOutputStream
. Note that these streams subclass
InputStream
and
OutputStream
and are thus a specialization of byte streams.
The following Try It Out shows you how to work with data and object streams.
Working with Data and Object Streams
try it out
In this Try It Out, you create a small class to illustrate the workings of an
ObjectOutputStream
. Note
that an
ObjectOutputStream
can write primitive data types as well (as a
DataOutputStream
using the
same method names), but can also serialize objects.
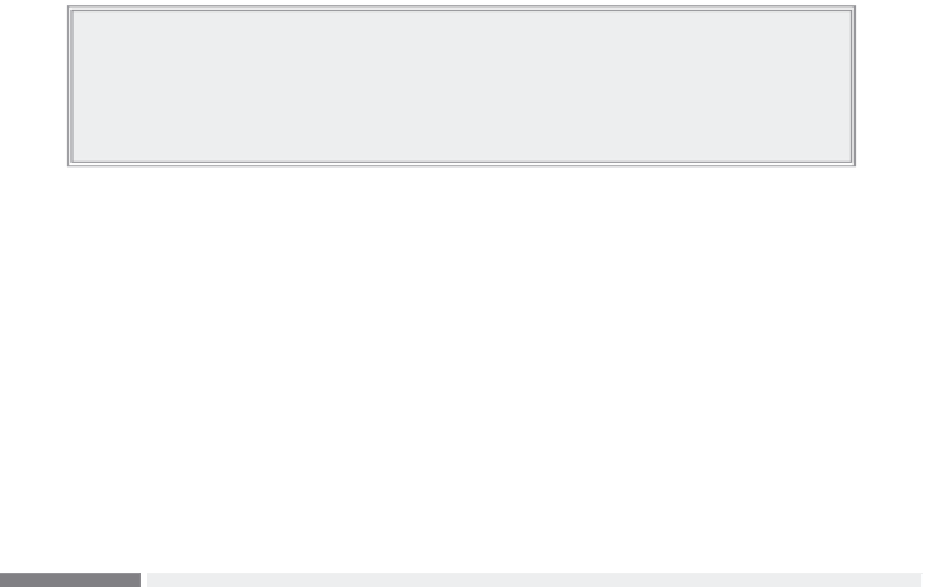


Search WWH ::

Custom Search