Java Reference
In-Depth Information
The
String
class includes an equality comparison method in the
equals()
method. This method
will return
true
for any two strings with a matching sequence of characters and
false
if there is
any difference in the characters. Consider the following code:
String myString = "I'm a string.";
String anotherString = "I'm a string, too.";
String oneMoreString = "I'm a string.";
myString.equals(oneMoreString); //this will evaluate as TRUE
myString.equals(anotherString); //this will evaluate as FALSE
eQuals versus ==
At this point, you might have tried to do something like the following:
String abc = "the letters a, b and c";
if (abc == "the letters a, b and c"){
System.out.println("Strings are equal");
}
Notice that Java actually prints out that the string
abc
equals “the letters a, b and
c”. So why do you need to use the
equals()
method?
The reasoning behind this is a bit tricky. For objects, it is perfectly fine to use
==
and
!=
to compare them, but note that Java will not check whether the two objects
are equal in the sense that they contain the same contents, but rather whether they
reference the same position in memory.
The following code sample shows this in a clearer way:
String abc = "the letters a, b and c";
String xyz = abc;
if(abc == xyz)
System.out.println("Both refer to the same memory address");
However, in some cases the use of
==
actually leads to the expected result when
checking the contents of a string. The reason behind this is due to the way the Java
Virtual Machine handles strings. Java makes use of a concept called “interning” to
reduce memory overhead when working with strings. This is particularly tricky if
you try to outsmart the JVM optimizer by instantiating two strings like this:
String abc = "the letters a, b and c";
String xyz = "the letters a, b and c";
if(abc == xyz){
System.out.println("Refers to same string");
} else {
System.out.println("Refers to different strings");
}
continues
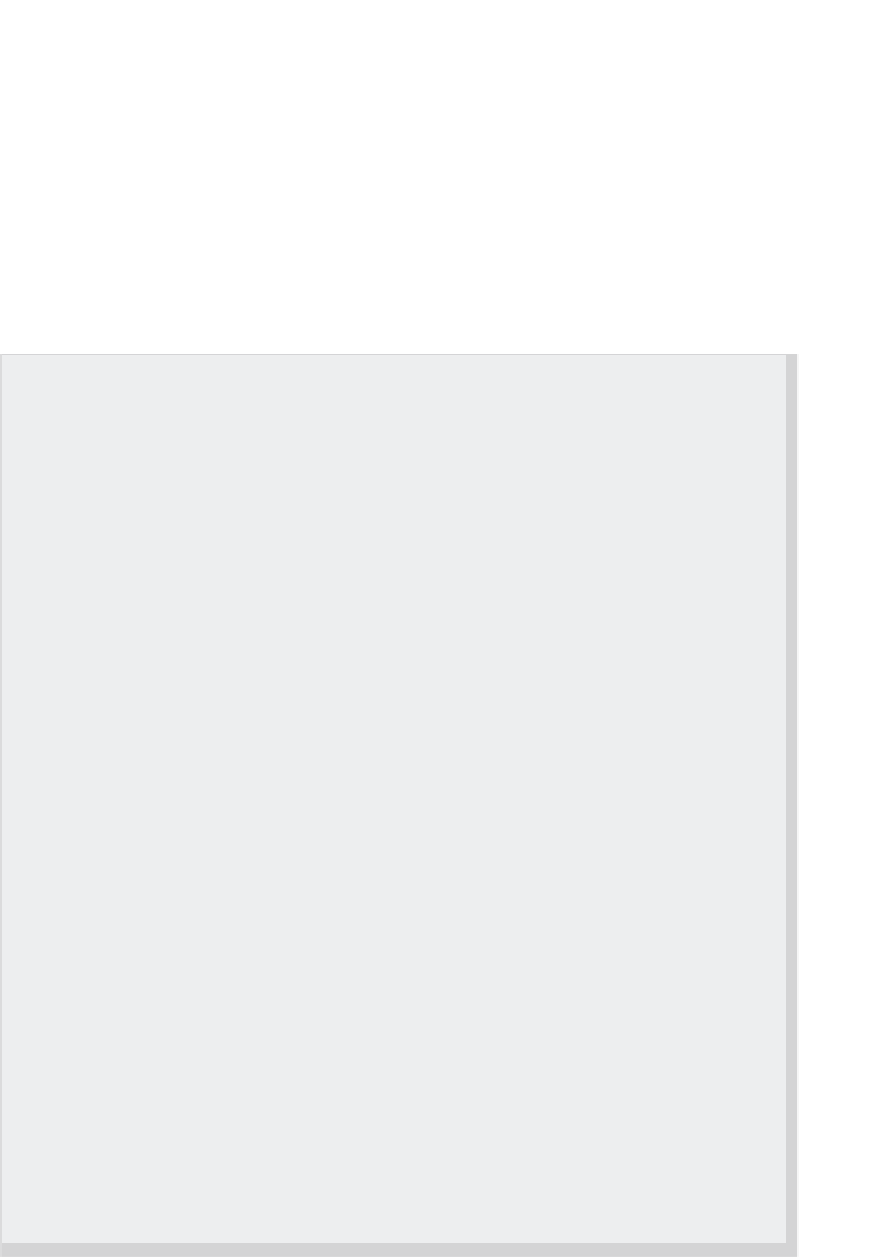
Search WWH ::

Custom Search