Java Reference
In-Depth Information
I'll explain this by providing an example. Let's modify the
Book
class so it looks as follows:
class Book {
static int maxAmountOfPages = 500;
String title = "Unknown Title";
String[] authors = new String[]{"Anonymous"};
int yearReleased = 2014, copiesSold = 0, nrOfPages = 1400;
}
Note the
static
keyword in front of the
maxAmountOfPages
class
variable. This variable denotes
the maximum amount of pages you currently support for topics, which could be used in combi-
nation with the
nrOfPages
instance
variable to check whether you can publish a given book, for
instance. Although the number of pages is different for each book you define, the maximum amount
of pages the printers support is a global property throughout all
Book
objects, is defined as a class
variable, and is thus accessible by all
Book
objects.
A code sample illustrating this concept might look like this:
Book superLargeBook = new Book();
superLargeBook.title = "Super Large Boring Book";
System.out.println("I have a book here with the title: "+superLargeBook.title);
System.out.println("Written by: "+superLargeBook.authors);
System.out.println("Released in: "+superLargeBook.yearReleased);
System.out.println("With number of pages: "+superLargeBook.nrOfPages);
System.out.println("However, we only support topics with max. pages: "
+superLargeBook.maxAmountOfPages);
Running this code outputs:
I have a book here with the title: Super Large Boring Book
Written by: [Ljava.lang.String;@1271ba
Released in: 2014
With number of pages: 1400
However, we only support topics with max. pages: 500
Note
You might have spotted the following line:
Written by: [
Ljava.lang.String;@1271ba
representation of composite types, w
hich is not very user friendly in this case.
There are two ways to resolve this issue. The first is to loop through the array
and show each element one by one (you will look at looping constructs in the
next chapter). The second way is to use so‐called “Collection” classes, which
are similar to arrays in the sense that they hold a bunch of information, but are
also much more flexible and efficient. In addition, their
String
representation
looks much friendlier. You will learn about collections later in this chapter.
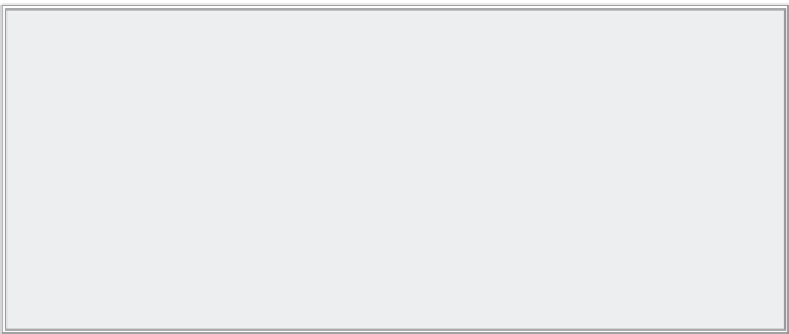
Search WWH ::

Custom Search