Java Reference
In-Depth Information
addPoint(int x, int y)
), the entity selection (method
contains(int x, int y)
), the
entity representation in XML format (method
toXML()
), and the entity
visualization (method
paint()
).
package
gis.model;
import
java.awt.*;
import
java.util.*;
public abstract class
MapEntity {
// variable "closed" is used to set the entity's endpoint
protected boolean
closed
#
false
;
public
MapEntity(){}
public void
close() {
this
.closed
#
true
; }
public abstract void
paint(Graphics2D g, Color color);
// these methods should be implemented in every subclass
public abstract void
addPoint(
int
x,
int
y);
public abstract boolean
contains(
int
x,
int
y);
public abstract
String toXML();
}
public class
MapSite
extends
MapEntity {
private
Point2D point
#
null
; // the site's 2D location
private static int
DIST
#
4; // the site's radius in pixel
public
MapSite(){}
// sets the site's coordinates
public void
addPoint(
int
x,
int
y) {
point
#
new
Point2D.Double(x, y);
}
// returns true if point x,y is inside the site's circle
public boolean
contains(
double
x,
double
y) {
double
dist
#
point.distance(x, y);
if
(dist <
#
DIST)
return true
;
return false
;
}
// converts the site's data to XML format
public
String toXML() {
StringBuffer stream
#
new
StringBuffer();
stream.append("\n <ENTITY>");
stream.append("\n <POINT X
#
"
!
'"'
!
(
int
)Math.round(point.getX())
!
'"'
!
" Y
#
"
!
'"'
!
(
int
)Math.round(point.getY())
!
'"'
!
"/>");
stream.append("\n </ENTITY>");
return
stream.toString();
}
public void
paint(Graphics2D g, Color color) { . . . }
}
public class
MapLink
extends
MapEntity {
private static int
DIST
#
4;// link's surrounding in pixel
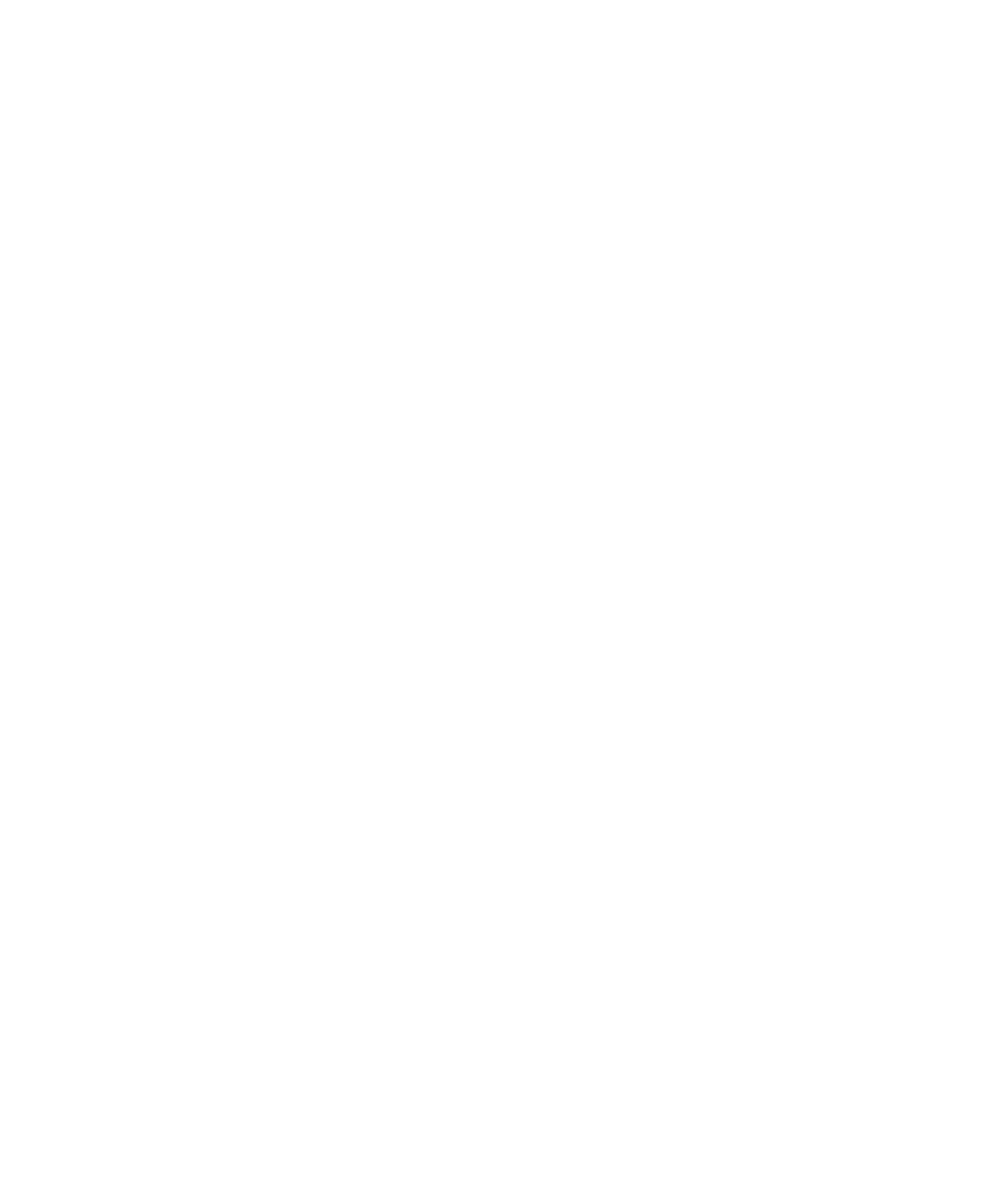