Java Reference
In-Depth Information
A
DVANCED
T
OPIC
10.7: Enumerated Types Revisited
In
Advanced Topic 5.3
, we introduced the concept of an enumerated type: a type
with a finite number of values. An example is
public enum FilingStatus { SINGLE, MARRIED }
In Java, enumerated types are classes with special properties. They have a finite
number of instances,
namely the objects defined inside the braces. For example,
there are exactly two
objects of the
FilingStatus
class:
FilingStatus.SINGLE
and
FilingStatus.MARRIED
. Since
FilingStatus
has no public constructor, it is impossible to construct
additional objects.
475
476
Enumeration classes extend the
Enum
class, from which they inherit
toString
and
clone
methods. The
toString
method returns a string that equals the
object's name. For example,
FilingStatus.SINGLE.toString()
returns
Ȓ
SINGLE
ȓ. The
clone
method returns the given object without making a copy.
After all, it should not be possible to generate new objects of an enumeration
class.
The
Enum
class inherits the
equals
method from its superclass,
Object
.
Thus, two enumeration constants are only considered equal when they are
identical.
You can add your own methods and constructors to an enumeration class, for
example
public enum CoinType
{
PENNY(0.01), NICKEL(0.05), DIME(0.1),
QUARTER(0.25);
CoinType(double aValue) { value = aValue; }
public double getValue() { return value; }
private double value;
}
This
CoinType
class has exactly four instances:
CoinType.PENNY
,
CoinType.NICKEL
,
CoinType.DIME
, and
CoinType.QUARTER
. If you



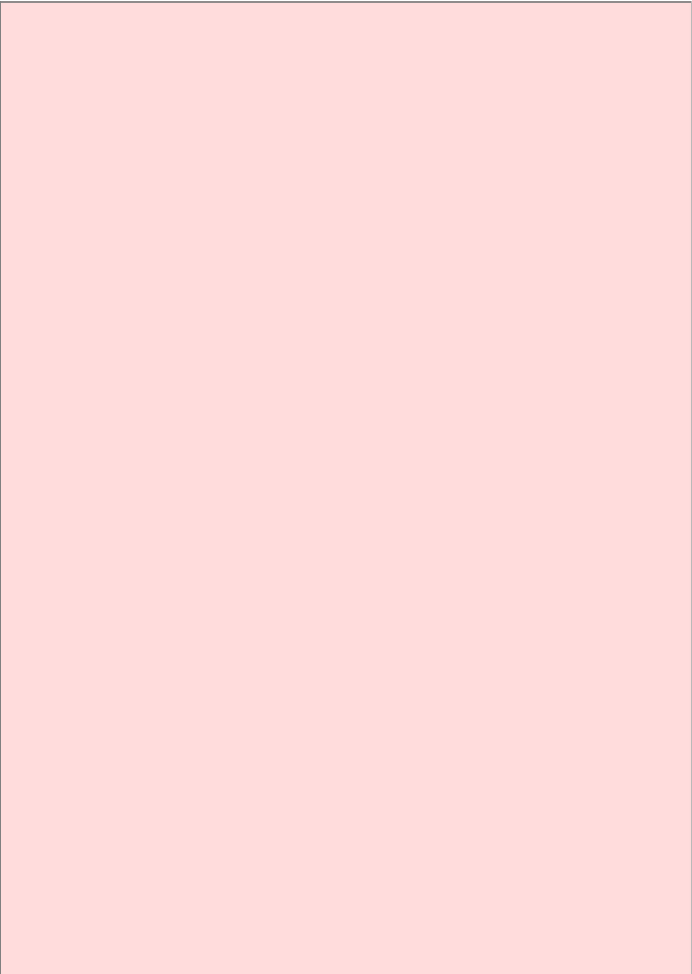

