Java Reference
In-Depth Information
You can use this method to transfer money from one bank account to another:
BankAccount momsAccount = . . . ;
BankAccount harrysAccount = . . . ;
momsAccount.transfer(1000, harrysAccount);
You can also use the method to transfer money into a
CheckingAccount
:
CheckingAccount harrysChecking = . . . ;
momsAccount.transfer(1000, harrysChecking);
//
OK to pass a
CheckingAccount
reference to a method expecting a
BankAccount
The
transfer
method expects a reference to a
BankAccount
, and it gets a
reference to the subclass
CheckingAccount
. Fortunately, rather than complaining
about a type mismatch, the compiler simply copies the subclass reference
harrysChecking
to the supercl
ass reference
other
. The
transfer
method
doesn't actually know that, in this
case,
other
refers to a
CheckingAccount
reference. It knows only that
other
is a
BankAccount
, and it doesn't need to
know anything else. All it cares about is that the
other
object can carry out the
deposit
method.
453
454
Very occasionally, you need to carry out the opposite conversion, from a superclass
reference to a subclass reference. For example, you may have a variable of type
Object
, and you know that it actually holds a
BankAccount
reference. In that
case, you can use a cast to convert the type:
BankAccount anAccount = (BankAccount) anObject;
However, this cast is somewhat dangerous. If you are wrong, and
anObject
actually refers to an object of an unrelated type, then an exception is thrown.
To protect against bad casts, you can use the
instanceof
operator. It tests whether
an object belongs to a particular type. For example,
anObject
instanceof
BankAccount
returns
true
if the type of
anObject
is convertible to
BankAccount
. This
happens if
anObject
refers to an actual
BankAccount
or a subclass such as
SavingsAccount
. Using the
instanceof
operator, a safe cast can be
programmed as follows:

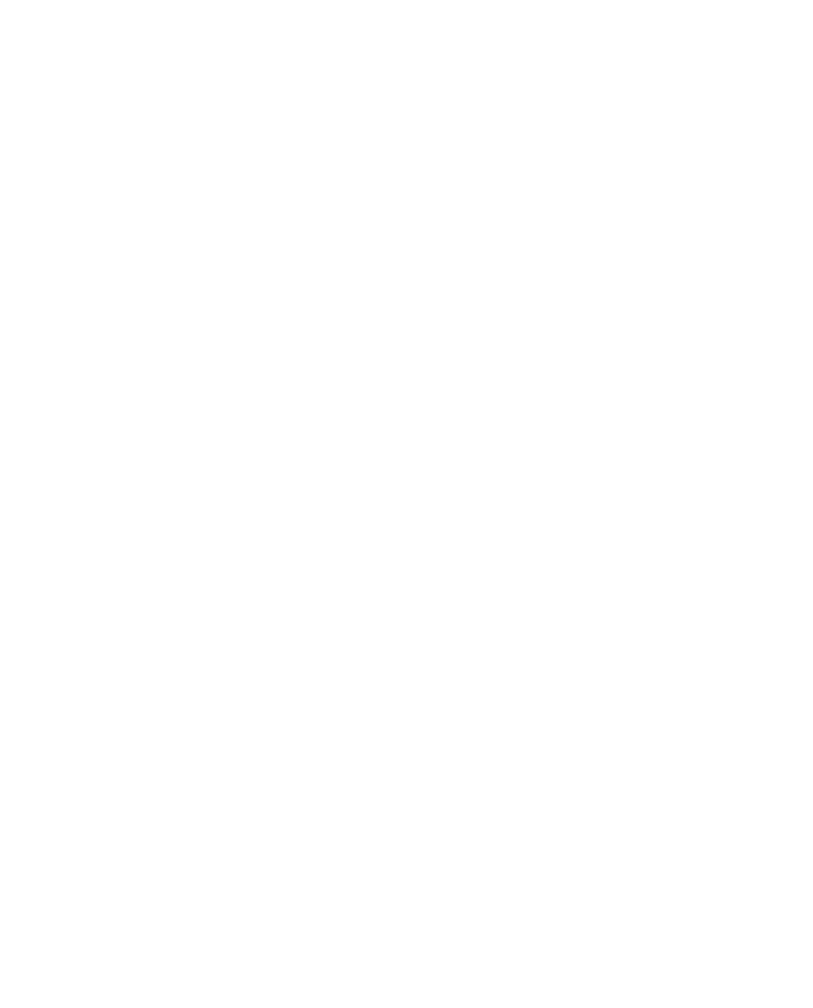
