Java Reference
In-Depth Information
13 {
14
public static void
main(String[] args)
15 {
16 JFrame frame =
new
JFrame();
17
18
//
The button to trigger the calculation
19 JButton button =
new
JButton(
"Add
Interest"
);
20
21
//
The application adds interest to this bank account
22
final
BankAccount account =
new
BankAccount(INITIAL_BALANCE);
23
24
//
The label for displaying the results
25
final
JLabel label =
new
JLabel(
26
"balance: "
+
account.getBalance());
27
28
//
The panel that holds the user interface components
29 JPanel panel =
new
JPanel();
30 panel.add(button);
31 panel.add(label);
32 frame.add(panel);
33
34
class
AddInterestListener
implements
ActionListener
35 {
36
public void
actionPerformed(ActionEvent event)
37 {
38
double
interest =
account.getBalance()
39 * INTEREST_RATE /
100
;
40 account.deposit(interest);
41 label.setText(
42
"balance: "
+
account.getBalance());
43 }
44 }
45
46 ActionListener listener =
new
AddInterestListener();
47 button.addActionListener(listener);
48
416
417

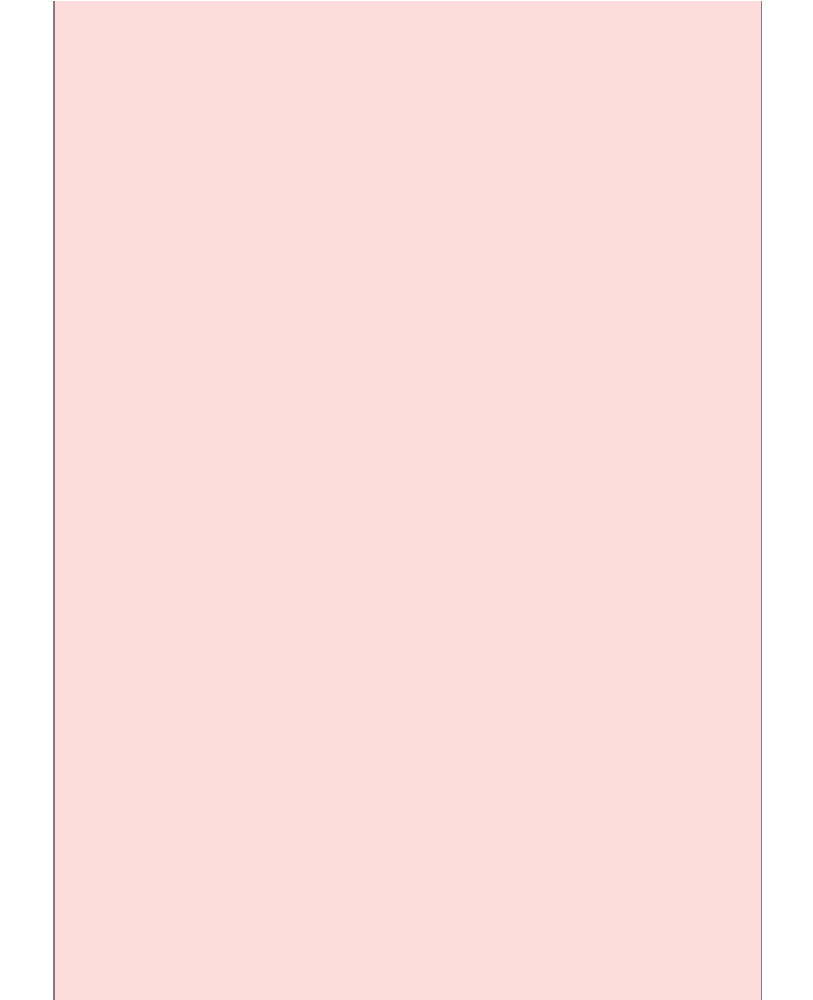
