Java Reference
In-Depth Information
356
There are three ways to initialize a static field:
1. Do nothing. The static field is then initialized with
0
(for numbers),
false
(for
boolean
values), or
null
(for objects).
2. Use an explicit initializer, such as
public class BankAccount
{
. . .
private static int lastAssignedNumber =
1000
;
}
The initialization is executed once when the class is loaded.
3. Use a static initialization block (see
Advanced Topic 8.3
).
Like instance fields, static fields should always be declared as
private
to ensure
that methods of other classes do not change their values. The exception to this rule are
static constants, which may be either private or public. For example, the
BankAccount
class may want to define a public constant value, such as
public class BankAccount
{
. . .
public static final double OVERDRAFT_FEE = 5;
}
Methods from any class refer to such a constant as
BankAccount.OVERDRAFT_FEE
.
It makes sense to declare constants as
static
Ȍyou wouldn't want every object of
the
BankAccount
class to have its own set of variables with these constant values.
It is sufficient to have one set of them for the class.
Why are class variables called
static
? As with static methods, the
static
keyword itself is just a meaningless holdover from C++. But static fields and static
methods have much in common: They apply to the entire class, not to specific
instances of the class.


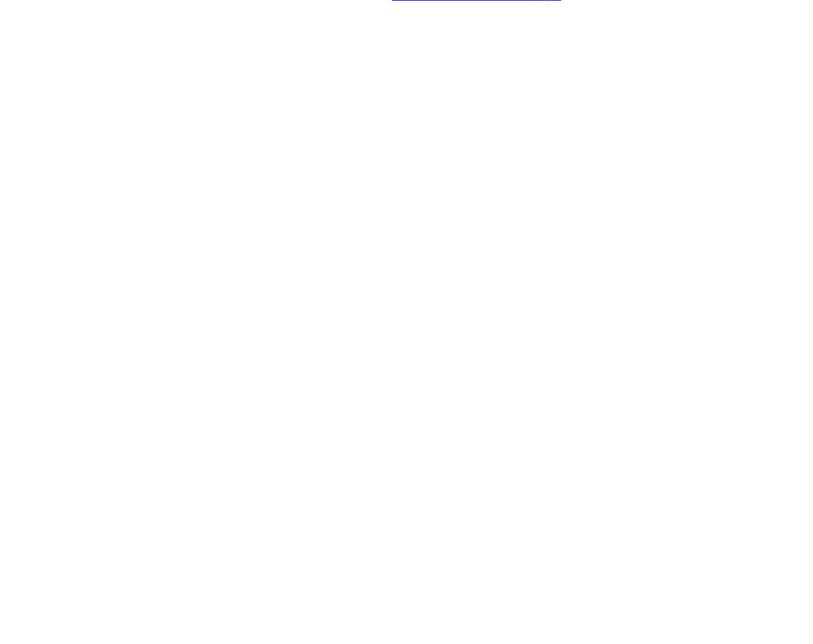