Java Reference
In-Depth Information
Here is a typical example of a static method that carries out some simple algebra: to
compute
p
percent of the amount
a
. Because the parameters are numbers, the method
doesn't operate on any objects at all, so we make it into a static method:
/**
Computes a percentage of an amount.
@param p
the percentage to apply
@param a
the amount to which the percentage is applied
@return p
percent of a
*/
public static double percentOf(double p, double a)
{
return (p / 100) * a;
}
352
353
You need to find a home for this method. Let us come up with a new class (similar to
the
Math
class of the standard Java library). Because the
percentOf
method has to
do with financial calculations, we'll design a class
Financial
to hold it. Here is the
class:
public class Financial
{
public static double percentOf(double p, double a)
{
return (p / 100) * a;
}
// More financial methods can be added here.
}
When calling a static method, you supply the name of the class containing the method
so that the compiler can find it. For example,
double tax =
Financial.
percentOf(taxRate, total);
Note that you do not supply an object of type
Financial
when you call the method.
Now we can tell you why the
main
method is static. When the program starts, there
aren't any objects. Therefore, the first method in the program must be a static method.
You may well wonder why these methods are called static. The normal meaning of
the word static (Ȓstaying fixed at one placeȓ) does not seem to have anything to do

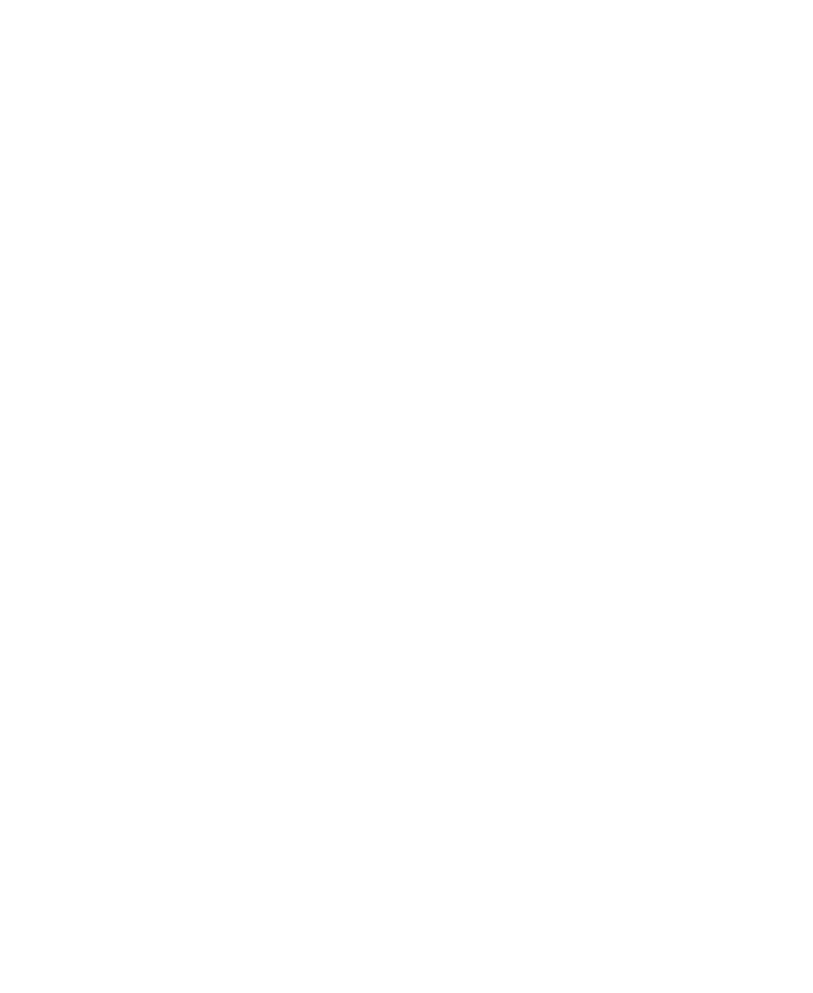
