Java Reference
In-Depth Information
. . .
}
That code would produce errors if another statement in the method referred to
amount expecting it to be the value of the parameter, and it will confuse later
programmers maintaining this method. You should always treat the parameter
variables as if they were constants. Don't assign new values to them. Instead,
introduce a new local variable.
public void deposit(double amount)
{
double newBalance = balance + amount;
. . .
}
345
346
A
DVANCED
T
OPIC
8.1: Call by Value and Call by
Reference
In Java, method parameters are copied into the parameter variables when a method
starts. Computer scientists call this call mechanism Ȓcall by valueȓ. There are some
limitations to the Ȓcall by valueȓ mechanism. As you saw in
Common Error 8.1
, it
is not possible to implement methods that modify the contents of number
variables. Other programming languages such as C++ support an alternate
mechanism, called Ȓcall by referenceȓ. For example, in C++ it would be an easy
matter to write a method that modifies a number, by using a so-called reference
parameter. Here is the C++ code, for those of you who know C++:
// This is C++
class BankAccount
{
public:
void transfer(double amount, double
&
otherBalance)
// otherBalance is a double
&
, a reference to a double
{
balance = balance - amount;
otherBalance = otherBalance + amount;
// Works in
C++
}
. . .



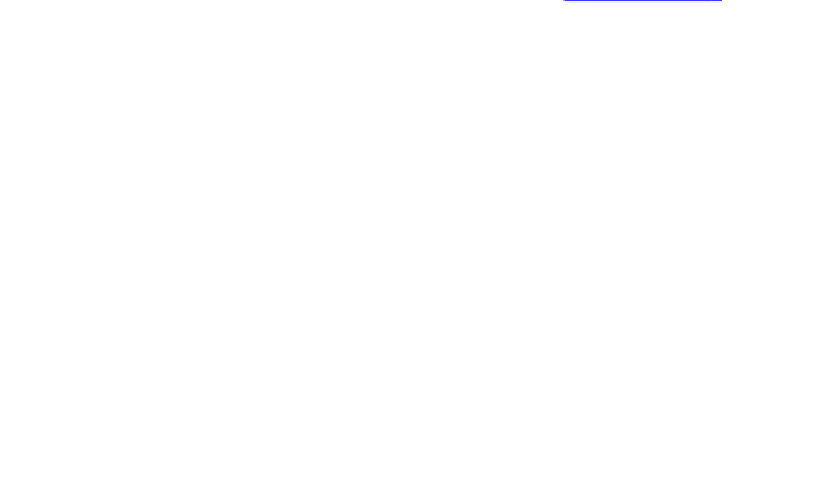