Java Reference
In-Depth Information
7.5.3 Finding the Maximum or Minimum
Suppose you want to find the account with the largest balance in the bank. Keep a
candidate for the maximum. If you find an element with a larger value, then replace
the candidate with that value. When you have reached the end of the array list, you
have found the maximum.
To compute the maximum or minimum value of an array list, initialize a
candidate with the starting element. Then compare the candidate with the
remaining elements and update it if you find a larger or smaller value.
There is just one problem. When you visit the beginning of the array, you don't yet
have a candidate for the maximum. One way to overcome that is to set the
candidate to the starting element of the array and start the comparison with the next
element.
302
303
BankAccount largestYet = accounts.get(0);
for (int i = 1; i < accounts.size(); i++)
{
BankAccount a = accounts.get(i);
if (a.getBalance() > largestYet.getBalance())
largestYet = a;
}
return largestYet;
Now we use an explicit
for
loop because the loop no longer visits all elementsȌit
skips the starting element.
Of course, this approach works only if there is at least one element in the array list.
It doesn't make a lot of sense to ask for the largest element of an empty collection.
We can return
null
in that case:
if (accounts.size() == 0) return null;
BankAccount largestYet = accounts.get(0);
. . .
See Exercises R7.5 and R7.6 for slight modifications to this algorithm.

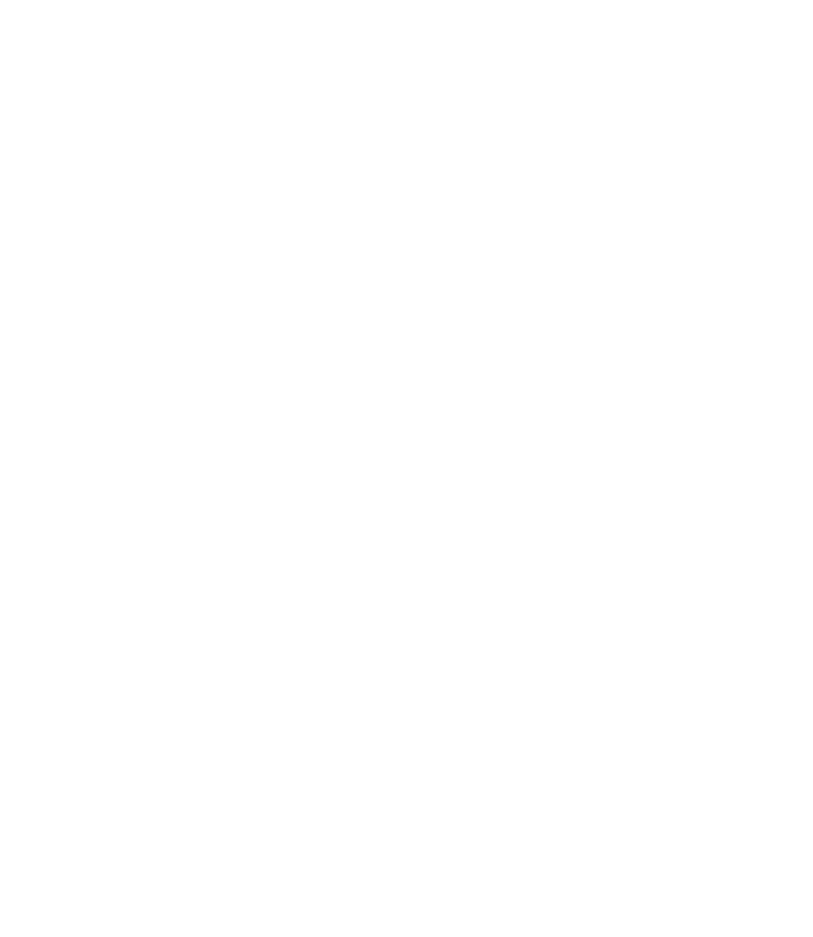
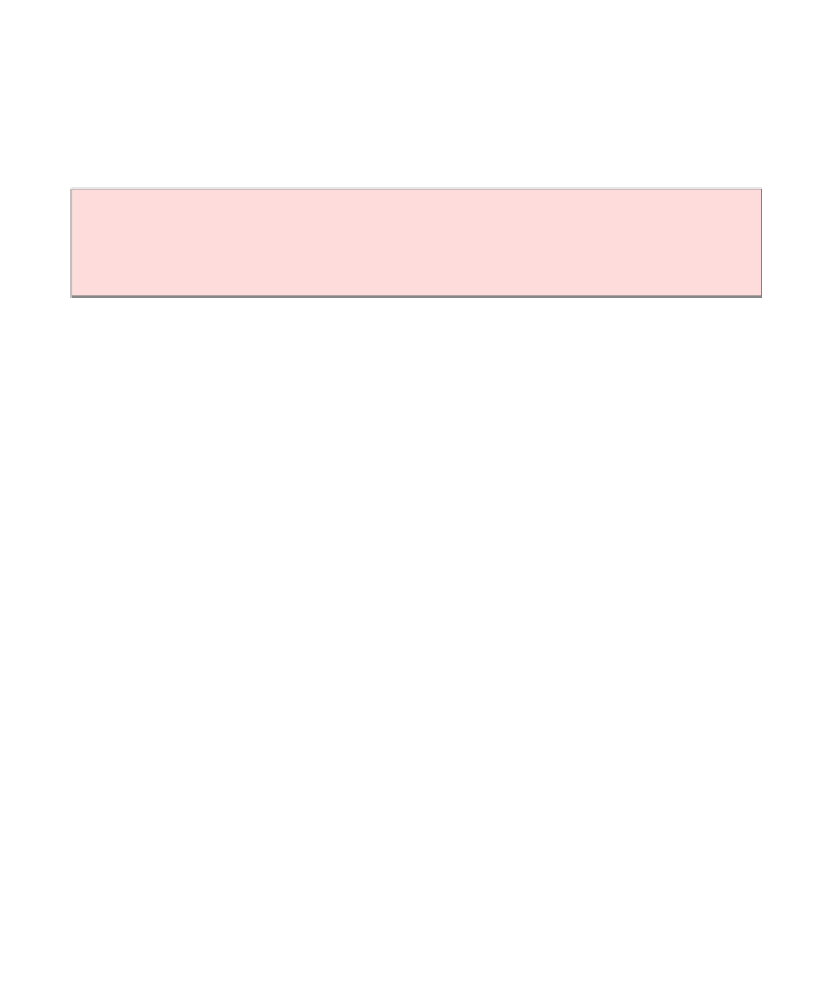