Java Reference
In-Depth Information
It is not difficult to inadvertently write an infinite while loop. Your
program will simply run indefinitely. To stop an infinite loop, you need to
stop the JVM. This is done by pressing Ctrl+C at the command prompt
window in which you are running your Java program.
The following WhileDemo program has three while loops. Study the pro-
gram carefully and try to determine what each while loop is doing.
The first loop in the WhileDemo program uses the print() method, which
is similar to println(), except that it does not move the cursor down to the
next line.
public class WhileDemo
{
public static void main(String [] args)
{
//Loop #1
int i = 10;
while(i > 0)
{
System.out.print(i + “ “);
i--;
}
System.out.println();
//Loop #2
int x = Integer.parseInt(args[0]);
long sum = 0;
i = 0;
while(i <= x)
{
sum += x;
i++;
}
System.out.println(“sum = “ + sum);
//Loop #3
long f = 1;
while(x >= 1)
f *= x--;
System.out.println(“f = “ + f + “ and x = “ + x);
}
}
In the first while loop in the WhileDemo program, the variable i is the loop
counter. It starts at 10 and is decremented by 1 each time through the loop.


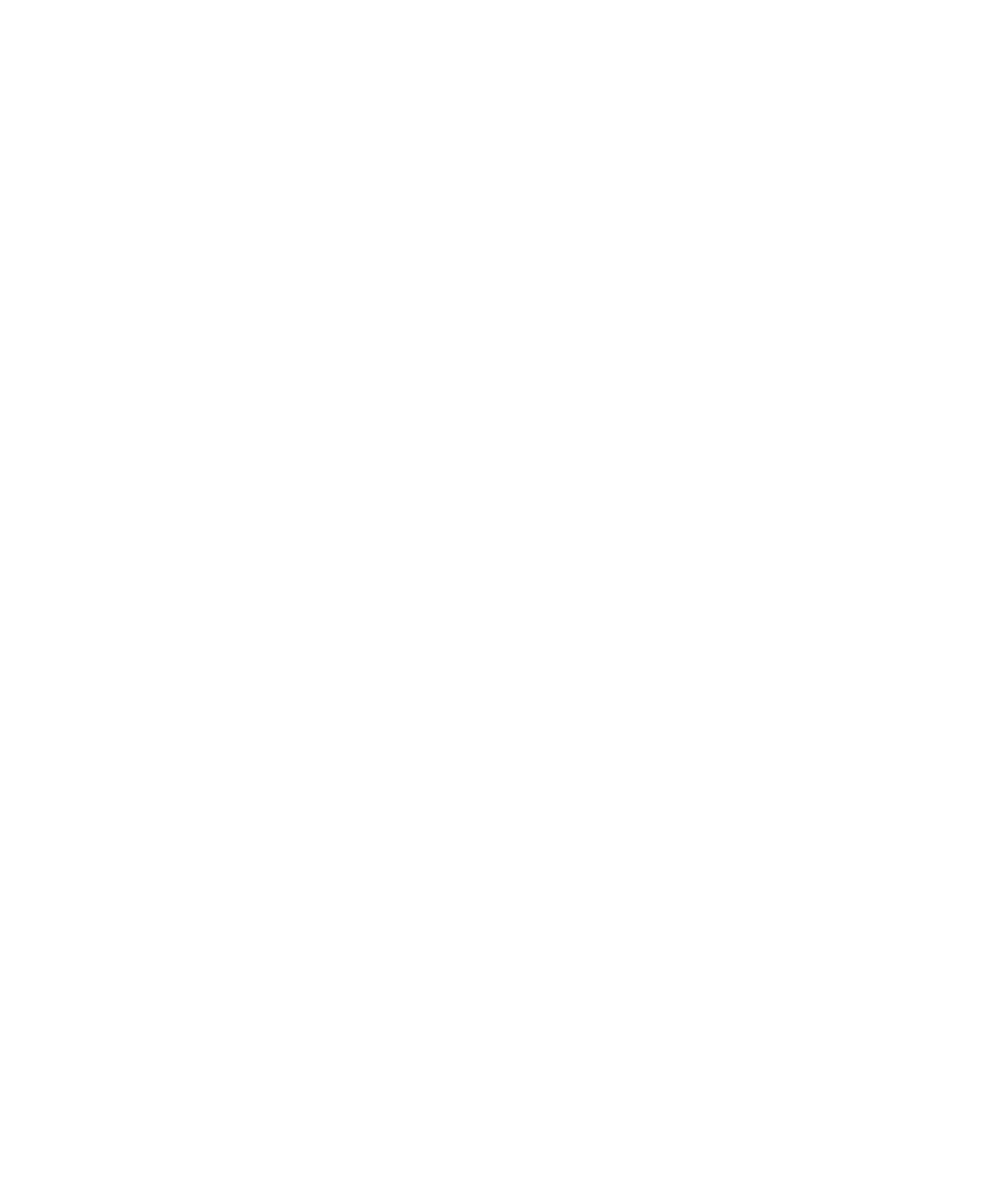