Java Reference
In-Depth Information
Frequently in programming you need to change the flow of control—that is,
the order in which statements are executed. There are three basic techniques
for changing the flow of control of a program:
Invoke a method.
This involves the flow of control leaving the current
method and moving to the method being invoked. For example, when
you invoke the println() method, flow of control leaves main(), jumps
to println(), and returns to main() when the println() method is finished.
We will cover methods in detail in Chapter 5, “Methods.”
Decision making.
This is when a certain criterion determines which path
the flow of control takes. Java has two mechanisms for making decisions:
the if/else statement and the switch statement. (The ternary operator
can also be used for decision making, but it is basically a shortcut ver-
sion of an if/else statement.)
Repetition.
Repetition occurs when a task needs to be repeated a certain
number of times, and is often referred to as a loop. Java has three loop-
ing mechanisms: the for loop, the while loop, and the do/while loop.
The decision-making and repetition statements are known as
control struc-
tures
, because you use them to control the flow of a program. All of the control
structures involve some type of Boolean decision, so you will need a good
understanding of Boolean logic and truth tables, which I will discuss next.
Boolean Logic
Boolean logic refers to the logic of combining two or more Boolean expressions
into a single Boolean expression. I will discuss four types of logic when work-
ing with combined Boolean statements:
and.
The combined expression is true only if both parts are true.
or.
The combined expression is true if either part is true.
exclusive or.
The combined expression is true only if one part is true and
the other part is false.
not.
Negates a Boolean expression.
I will now discuss the logic and truth tables for these Boolean operators.
The and Operator
Suppose I make the following statement:
It is raining out today and x is equal to 4.
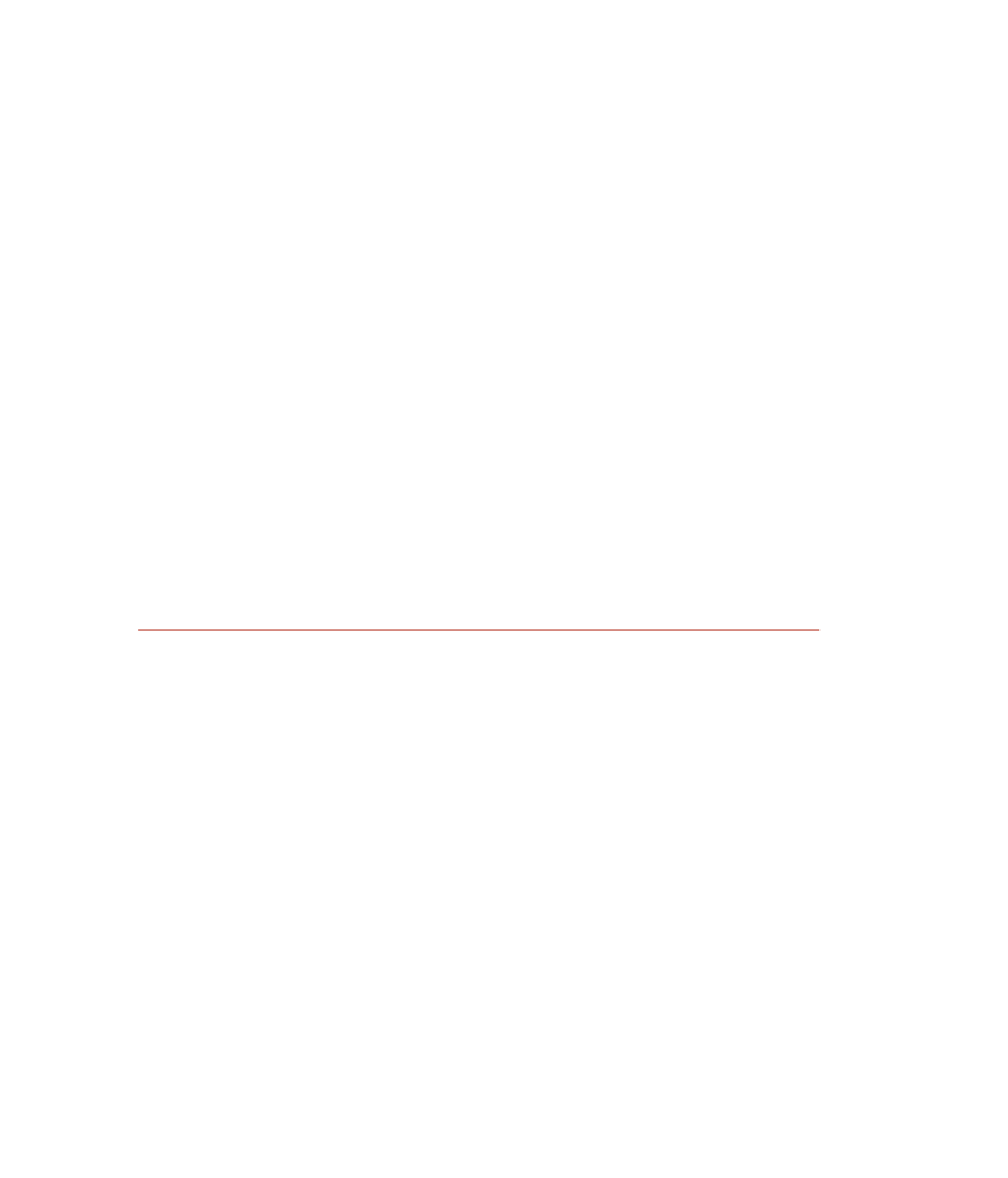
