Java Reference
In-Depth Information
The constructors in the high-level stream classes take in other streams. I
can tell that BufferedOutputStream is a high-level stream because both of
its constructors take in an object of type OutputStream. Checking the
constructor's parameters is the simplest way to determine if a stream is
high level or low level. I can tell that FileOutputStream is a low-level
stream because the parameters of its constructors are types like String
and File.
Study the following ChainDemo program and try to determine what the
program is doing.
import java.io.*;
public class ChainDemo
{
public static void main(String [] args)
{
try
{
FileOutputStream fileOut =
new FileOutputStream(“data.txt”);
BufferedOutputStream buffer =
new BufferedOutputStream(fileOut);
DataOutputStream dataOut =
new DataOutputStream(buffer);
dataOut.writeUTF(“Hello!”);
dataOut.writeInt(4);
dataOut.writeDouble(100.0);
dataOut.writeDouble(72.0);
dataOut.writeDouble(89.0);
dataOut.writeDouble(91.0);
dataOut.close();
buffer.close();
fileOut.close();
}catch(IOException e)
{
e.printStackTrace();
}
}
}
The ChainDemo program does not display any output, but it does create a
new file named data.txt. Because the data is created using a DataOutput-
Stream, you need to use a DataInputStream to read the data. And because the
data is in a file, you need to also use a FileInputStream. If you want to buffer
the data, a BufferedInputStream can also be used. In the following ReadData

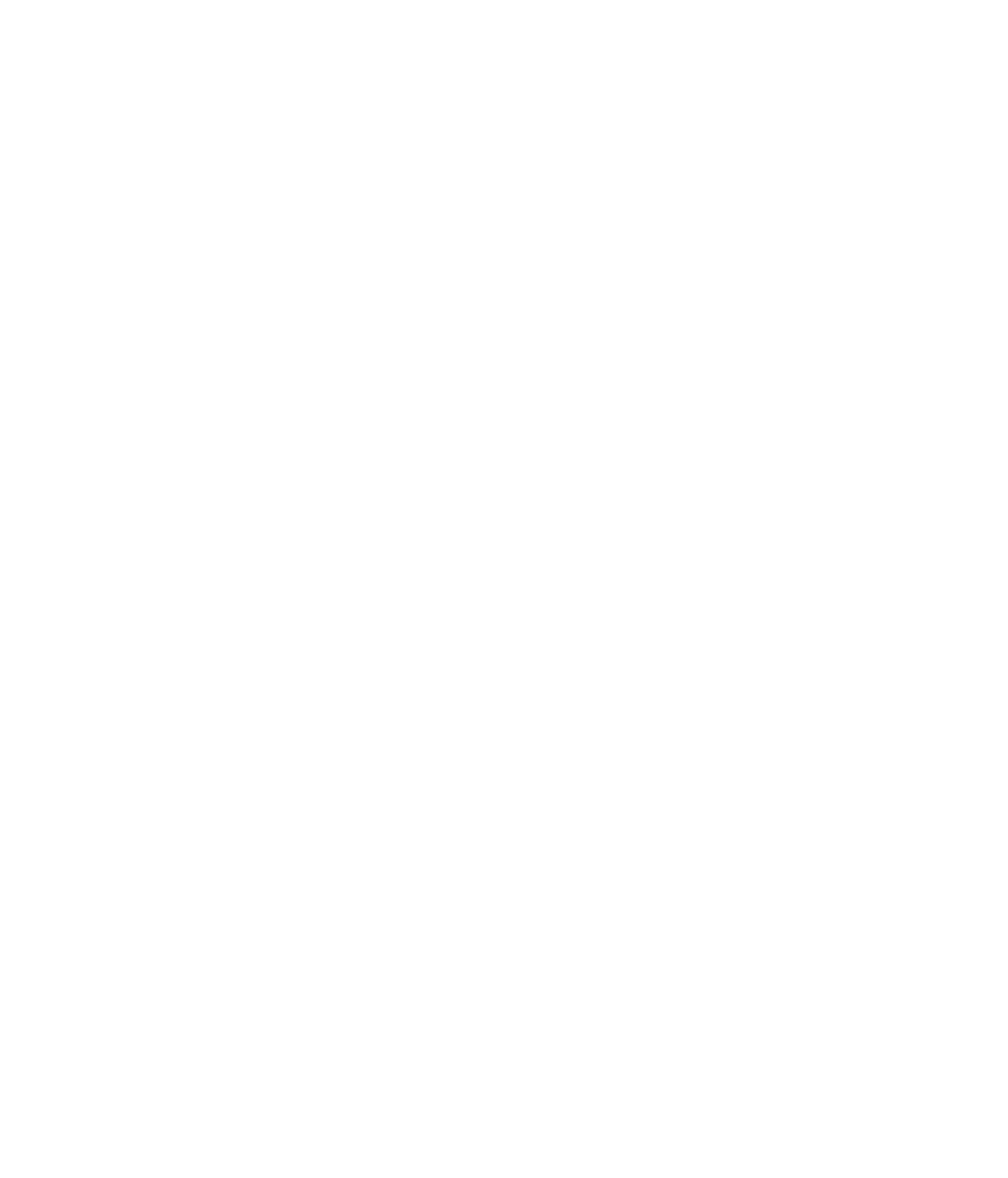