Java Reference
In-Depth Information
WindowListener that can listen for a WindowEvent. The EventDemo2 program
that follows demonstrates using SimpleWindowCloser to end a program when
a user closes the Frame. It is similar to the EventDemo program earlier. Study
the program, and try to determine how the event handling ends the program.
import java.awt.*;
public class EventDemo2 extends Frame
{
private Button go;
public EventDemo2(String title)
{
super(title);
go = new Button(“Go”);
RandomColor changer = new RandomColor(this);
go.addActionListener(changer);
this.setLayout(new FlowLayout());
this.add(go);
//Register a listener to this Frame.
SimpleWindowCloser closer = new SimpleWindowCloser();
this.addWindowListener(closer);
}
public static void main(String [] args)
{
Frame f = new EventDemo2(“SimpleWindowCloser”);
f.setSize(300,300);
f.setVisible(true);
}
}
The output of the EventDemo2 program looks the same as the window shown
in Figure 13.1. When the “X” is clicked, the Frame generates a WindowEvent and
invokes the windowClosing() method on the SimpleWindowCloser object. The
following statement causes the JVM to terminate (which closes the Frame as
well):
System.exit(0);
A Frame can be closed by invoking either the setVisible() method and
passing in false, or invoking the dispose() method. Both of these methods
are defined in the java.awt.Window class, which is the parent class of
Frame. Both methods hide the window, but the dispose() method also
frees up any screen resources that the window may be consuming.
We have discussed the delegation model and how listeners must implement
the event listener interfaces. I want to spend the remainder of this chapter
focusing on the various GUI components. Specifically, I want to discuss how
each component is instantiated and the types of events each generates.

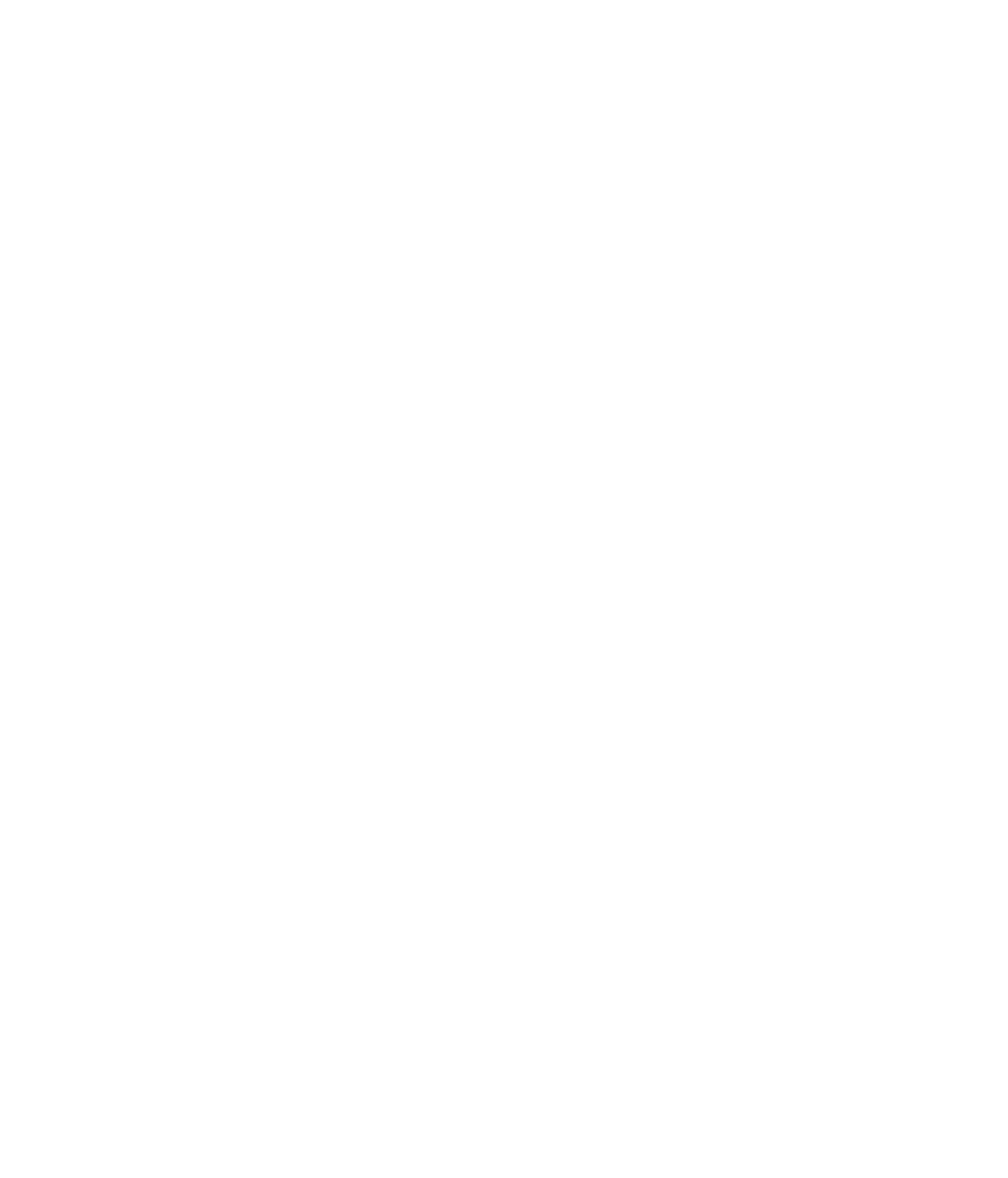