Java Reference
In-Depth Information
follows the try is checked. If the type of exception that occurred is listed in a
catch block, the exception is passed to the catch block much as an argument is
passed into a method parameter.
A try/catch block does not catch everything. If you say you want to catch a
football and a baseball is thrown, you will not catch it. If you say you want to
catch a NullPointerException and an ArithmeticException occurs, you will not
catch the ArithmeticException.
If you say you want to catch an Exception, then you will catch every
exception that might arise. Remember, all exceptions are child classes of
Exception, so through polymorphism, all exceptions are of type Exception.
The following try/catch block tries to catch a FileNotFoundException when
attempting to open a file:
try
{
System.out.println(“Opening file for reading...”);
file = new FileInputStream(fileName);
}catch(FileNotFoundException f)
{
System.out.println(“** Could not find “ + fileName + “ **”);
f.printStackTrace();
return -1;
}
If the file is found, no exception occurs, and the catch block is skipped. If the
file is not found, the constructor of FileInputStream throws a new FileNot-
FoundException, which is caught in the variable f of our catch block. I suppose
in a real-world situation, we would give the user an opportunity to try another
filename, but this catch block simply prints out the stack trace and returns a -1,
causing the method to stop executing and the flow of control to return to the
previous method on the call stack.
Writing try/catch Blocks
The following MyFileUtilities class has a readOneByte() method that contains
two try/catch blocks. Study the method and try to determine the flow of con-
trol if an exception does or does not occur in each try/catch block.
import java.io.*;
public class MyFileUtilities
{
private String fileName;
public MyFileUtilities(String name)
{

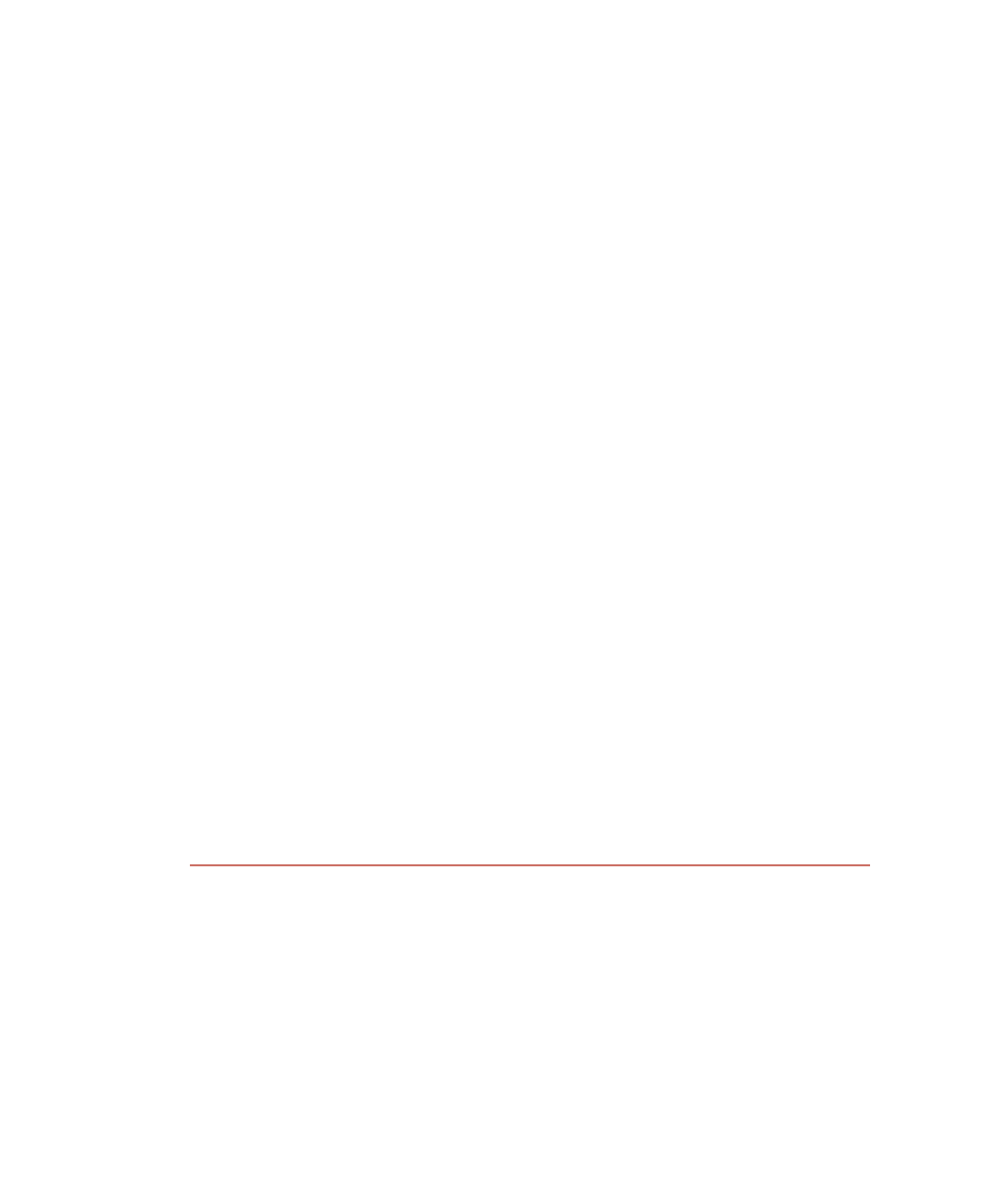
