Java Reference
In-Depth Information
Declaring Fields in Interfaces
Interfaces can contain fields, as long as the fields are declared both static and
final. They must be static because an interface cannot be instantiated, and the
requirement to be final is for program reliability.
It does not make sense for an interface to contain a nonstatic field
because the interface can never be instantiated. Recall that static fields do
not require an instance of a class to exist in memory, but nonstatic fields
are created only when an object is instantiated. Static fields in an
interface are allocated in memory when the interface is loaded by the
JVM's class loader.
The following PhoneHandler interface demonstrates an interface with three
fields. As with the PhoneHandler example, most examples of fields in an inter-
face are some type of enumeration.
package customer.service;
public interface PhoneHandler
{
public static final int LOCAL = 0;
public static final int LONG_DISTANCE = 1;
public static final int COLLECT = 2;
public void answer();
public boolean forward(int extension);
public void takeMessage(String message, String recipient);
}
Static members of a class are accessed using the class name. Similarly, static
fields in an interface are accessed using the name of the interface. For example,
the following statement accesses the LOCAL field of the PhoneHandler
interface:
if(x == PhoneHandler.LOCAL)
The following FieldDemo program demonstrates using the fields of the
PhoneHandler interface. Study the program and try to determine its output,
which is shown in Figure 10.10.
Figure 10.10
The output of the FieldDemo program.
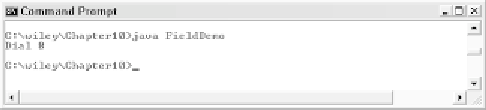

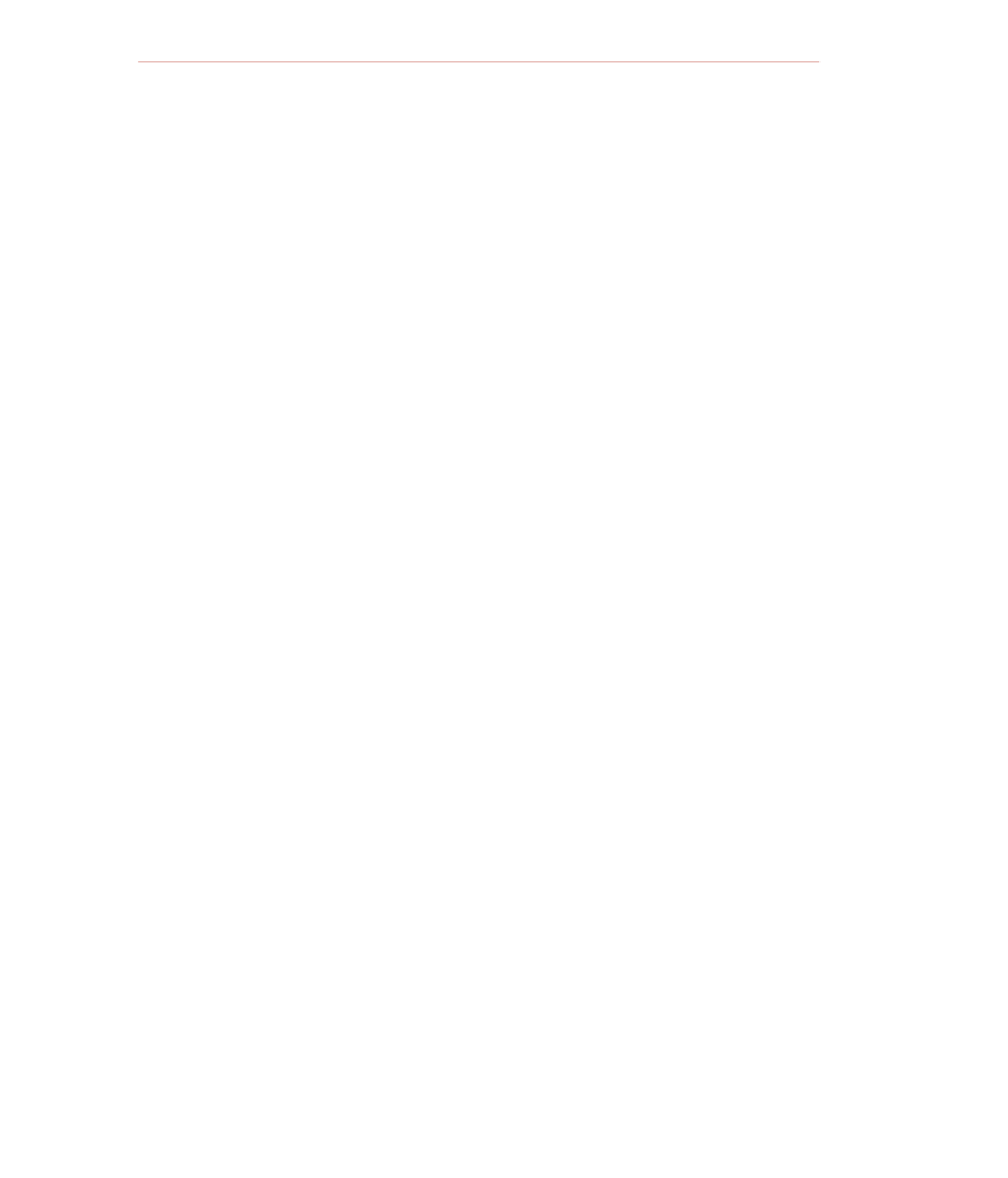
