Java Reference
In-Depth Information
Working with double arrays often involves nested loops because rows and
columns are involved. The DoubleArray program contains nested for loops
that fill the sums array with values. (The values are the sum of the row index
plus the column index.) Study the program carefully and try to determine its
output, which is shown in Figure 9.4.
public class DoubleArray
{
public static void main(String [] args)
{
System.out.println(“Instantiating a double array”);
int [] [] sums = new int[10][12];
System.out.println(“Filling the array with data”);
for(int row = 0; row < 10; row++)
{
for(int col = 0; col < 12; col++)
{
sums[row][col] = row + col;
}
}
System.out.println(“Displaying the array”);
for(int row = 0; row < sums.length; row++)
{
for(int col = 0; col < sums[row].length; col++)
{
System.out.print(sums[row][col] + “\t”);
}
System.out.println();
}
}
}
Notice in the DoubleArray program that the first set of nested for loops
used 10 and 12 as the upper limits of their respective loop control
variables. This works fine, but with arrays you should take advantage of
the length attribute.
Notice that the second set of nested for loops uses sums.length for the
row loop control variable. The value of sums.length is 10 because sums
refers to an array of 10 references. For the column loop control variable,
the for loop uses sums[row].length, which is 12 for each value of row.

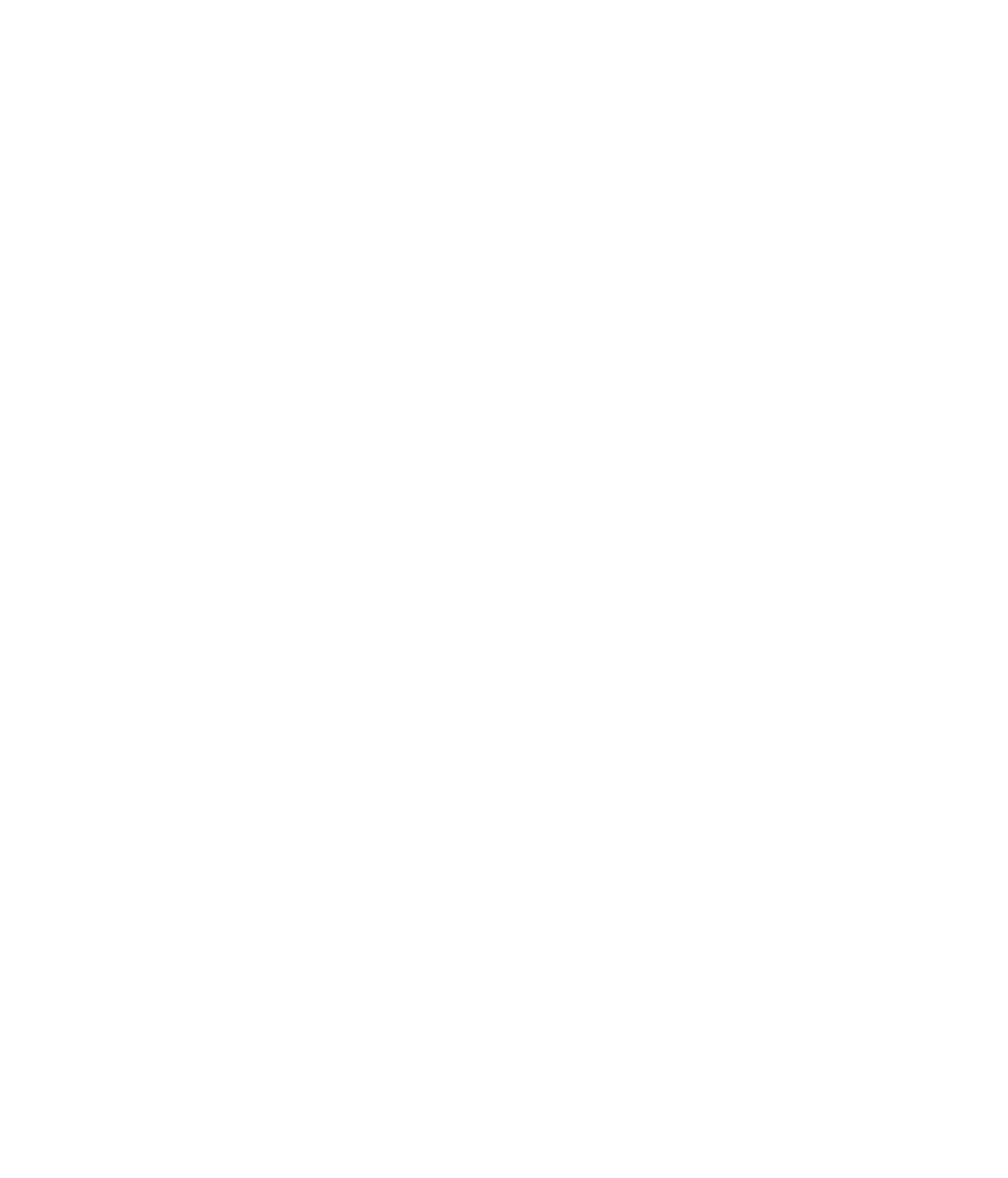