Java Reference
In-Depth Information
If you think about it, we really don't care what computePay() does in the
Employee class because it will not ever be invoked. Our assumption is that
child classes of Employee will override computePay(), so the implementation
of computePay() is insignificant.
This is where abstract methods become useful. If you want a class to contain
a particular method but you want the actual implementation of that method to
be determined by child classes, you can declare the method in the parent class
as abstract. Abstract methods consist of a method signature, but no method
body.
Listing 8.15 shows the computePay() method declared abstract. Notice the
method has no definition, and its signature is followed by a semicolon, not
curly braces.
public abstract class Employee
{
private String name;
private String address;
private int number;
public abstract double computePay();
//Remainder of class definition
}
Listing 8.15
The computePay() method is declared abstract and has no implementation
in the Employee class.
Declaring a method as abstract has two results:
The class must also be declared abstract. If a class contains an abstract
method, the class must be abstract as well.
■■
Any child class must either override the abstract method or declare
itself abstract.
■■
From a design point of view, putting an abstract method in a parent class
forces that particular behavior onto any child classes. A child class that inher-
its an abstract method must override it. If they do not, they must be abstract,
and any of their children must override it. Eventually, a descendant class has
to implement the abstract method; otherwise, you would have a hierarchy of
abstract classes that cannot be instantiated.
Why make a method abstract then? Because forcing a behavior on other
classes has its benefits. For example, suppose if computePay() is abstract in the
Employee class, we can be guaranteed that any nonabstract child class of
Employee will contain a computePay() method.
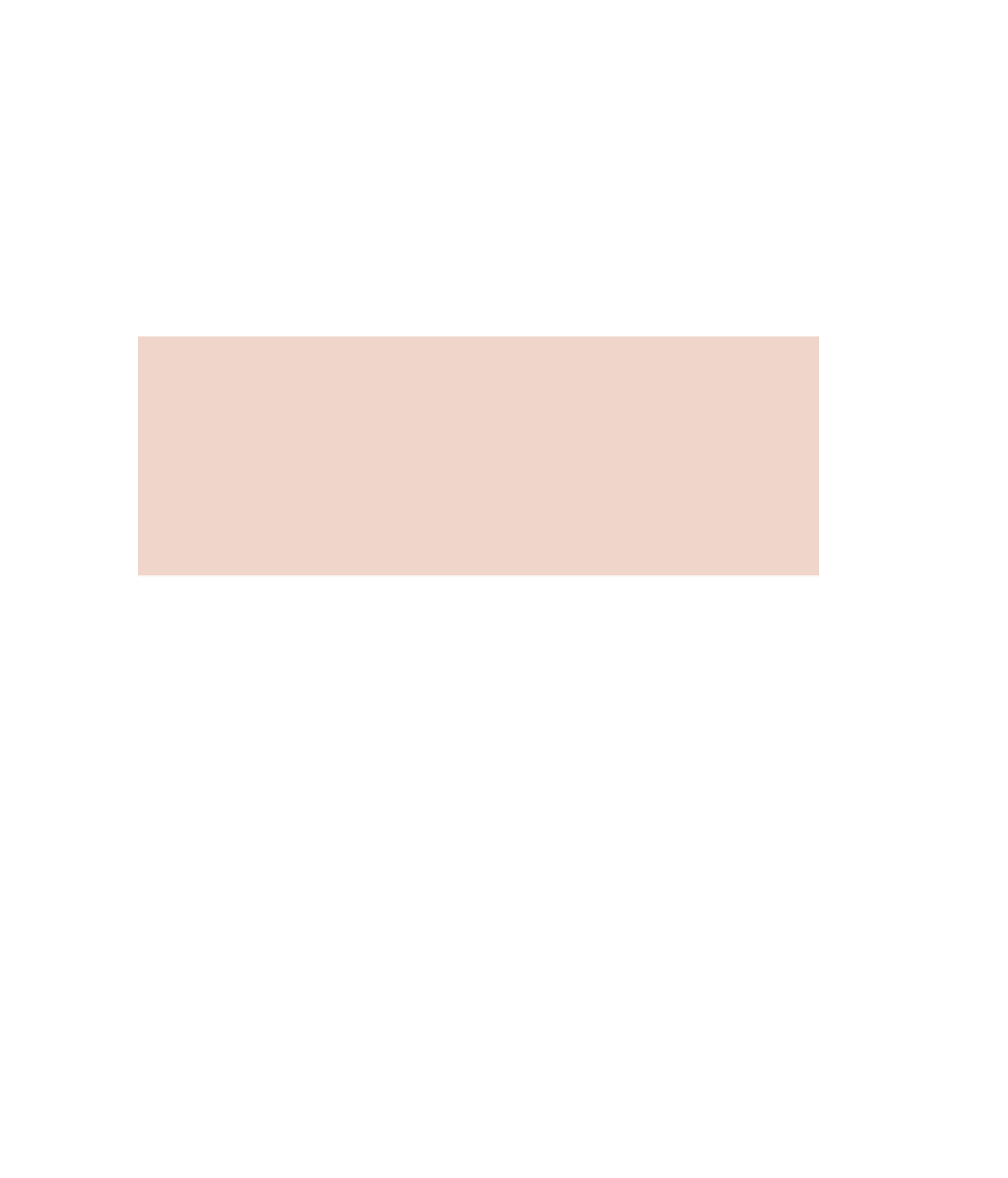