Java Reference
In-Depth Information
package payroll;
public class Employee
{
public String name;
public String address;
public int SSN;
public int number;
public Employee(String name, String address, int SSN, int number)
{
System.out.println(“Constructing an Employee”);
this.name = name;
this.address = address;
this.SSN = SSN;
this.number = number;
}
public void mailCheck()
{
System.out.println(“Mailing a check to “ + this.name
+ “ “ + this.address);
}
}
The payroll package is not something that needs to be instantiated or physi-
cally created. The compiler sees the package declaration and treats Employee
as being in the payroll package, even if Employee is the first class written in
payroll.
The package declaration must appear first in the class file. Notice the
following statement, which is the first statement in the Employee source
code. The class will not compile if an attempt is made to put any statement
besides comments before the package declaration.
package payroll;
If we want Salary and Hourly in the payroll package also, we simply add the
package statement to their class definition as well. For example, the Salary
class would look similar to:
package payroll;
public class Salary extends Employee
{
//Salary definition...
}
and the Hourly class would look similar to:
package payroll;
public class Hourly extends Employee
{

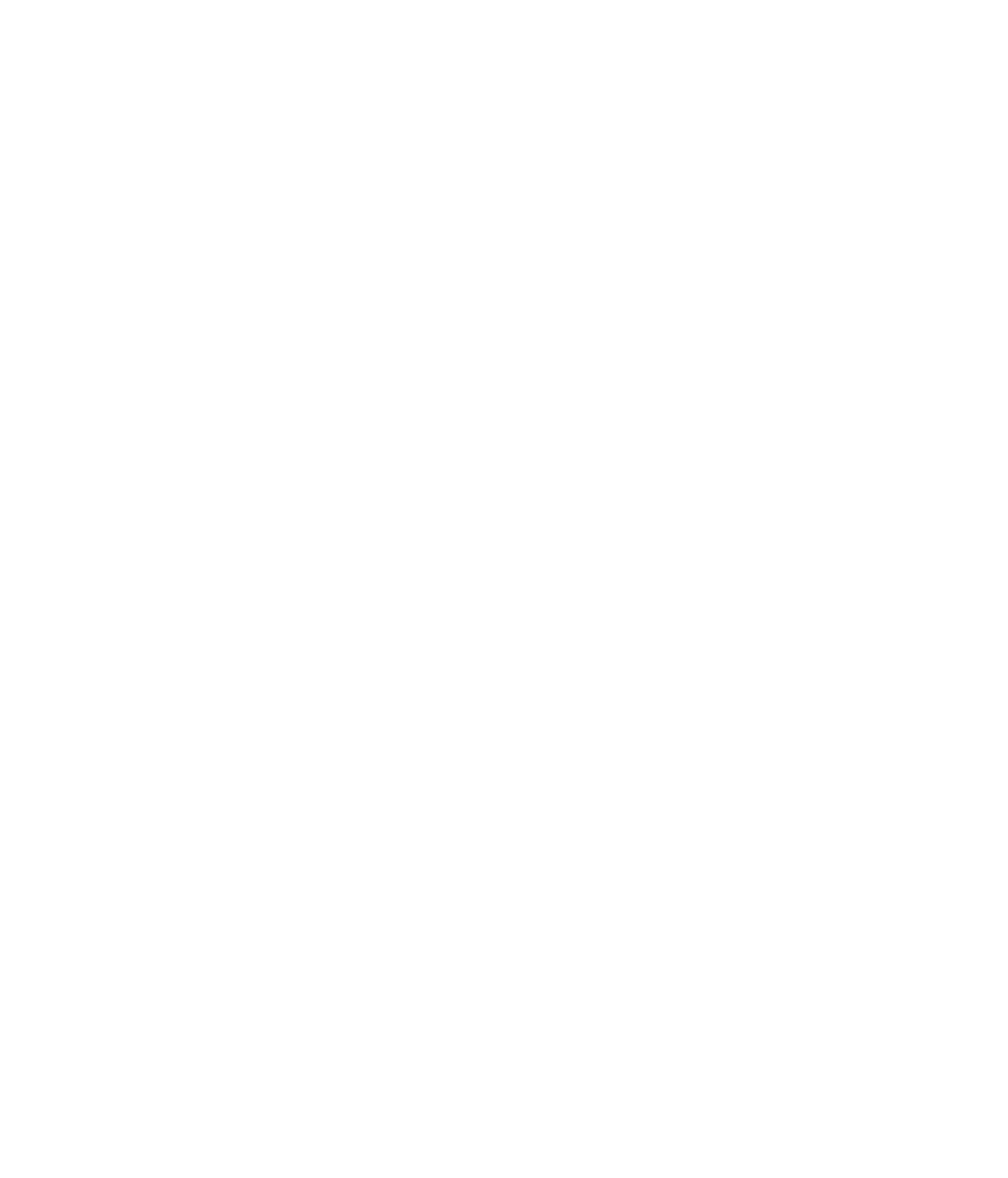