Java Reference
In-Depth Information
Review Questions
1.
In OOP, a class can extend another class. This concept is referred to as
________________.
2.
Suppose a class is declared as
public class Rectangle extends Triangle
What might be wrong with this design?
3.
True or False: A child inherits all the fields of its parent class.
4.
True or False: The first line of code in every constructor is either a call to this() or
a call to super() (possibly with arguments in both cases).
5.
True or False: The compiler adds a call to this() if a constructor does not add it
explicitly.
6.
If a class does not declare that it extends another class, the parent of this class is
_____________________.
7.
True or False: A class in Java can extend more than one class.
Use the following class definitions to answer the next four questions.
public class Vehicle
{
public int numOfWheels;
public Vehicle(int x)
{
numOfWheels = x;
}
public void drive()
{
System.out.println(“Driving a vehicle”);
}
}
public class Car extends Vehicle
{
public int numOfDoors;
public Car(int d, int w)
{
numOfDoors = d;
super(w);
}
public void drive()
{
System.out.println(“Driving a car”);
}
}
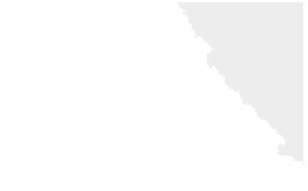
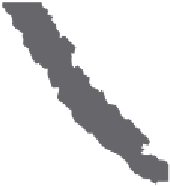

