Java Reference
In-Depth Information
When declaring a method final, the final keyword can appear anywhere
before the return value. (Typically it appears after the access specifier.) The fol-
lowing Employee class demonstrates declaring a final method.
public class Employee
{
public String name;
public String address;
public int SSN;
public int number;
public final void mailCheck()
{
System.out.println(“Inside Employee mailCheck: “
+ super.toString());
System.out.println(“Mailing a check to “ + this.name + “ “
+ this.address);
}
}
The Employee class declares its mailCheck() method as final. By doing
this, the Salary class will no longer compile because it attempts to
override mailCheck().
The Instantiation Process
A child object is an extension of its parent. When a child class is instantiated,
the parent class object needs to be constructed first. More specifically, a con-
structor in a parent class must execute before any constructor in the child class
executes.
In addition, if the child class has a grandparent, the grandparent object
needs to be constructed first. This process continues up the tree.
When an object is instantiated, the following sequence of events occurs:
1.
The new operator invokes a constructor in the child class.
2.
The child class may use the this keyword to invoke another constructor
in the child class. Eventually, a constructor in the child class will be
invoked that does not have this() as its first line of code.
3.
Before any statements in the child constructor execute, a parent class
constructor must be invoked. This is done using the super keyword. If a
constructor does not explicitly make a call using super, the compiler will
call super() with empty parentheses, thereby invoking the no-argument
parent class constructor.

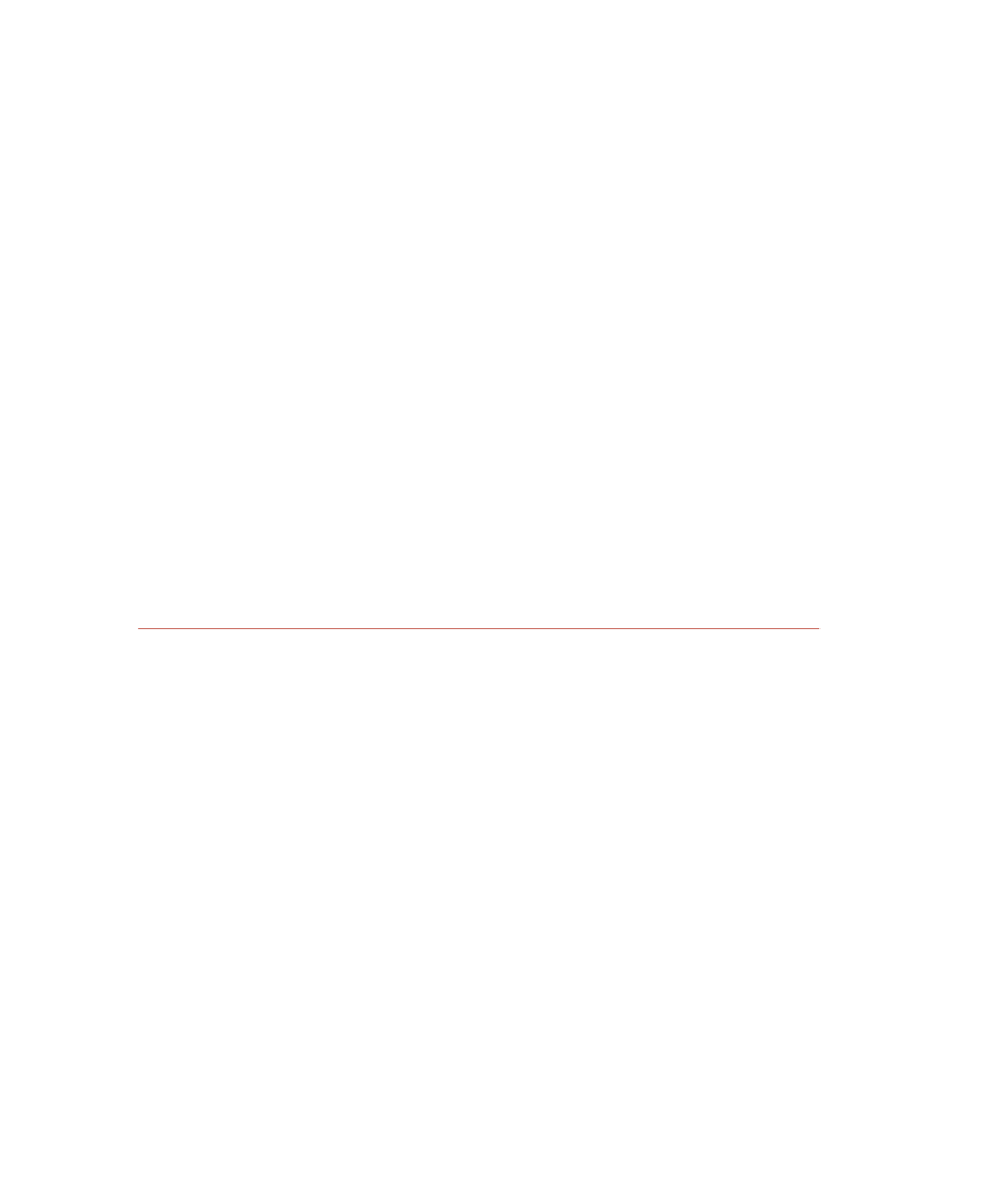
