Java Reference
In-Depth Information
Name, which can be any valid identifier
■■
List of parameters, which appears within parentheses
■■
Definition of the method
■■
In Java, the
definition
(often referred to as the
body
) of a method must appear
within the curly brackets that follow the method declaration. The details of
writing and invoking a method are discussed in Chapter 5, “Methods.” The fol-
lowing class demonstrates methods by adding two methods to the Employee
class.
public class Employee
{
public String name;
public String address;
public int number;
public int SSN;
public double salary;
public void mailCheck()
{
System.out.println(“Mailing check to “ + name
+ “\n” + address);
}
public double computePay()
{
return salary/52;
}
}
Keep in mind that this Employee class demonstrates how to add a method
to a class, so the method implementations are kept simple. For example, the
mailCheck() method simply prints out the name and address of the employee
receiving the check. The computePay() method simply divides the employee's
salary by 52, which assumes that the salary is an annual amount. The details of
computing taxes and so forth are omitted for brevity.
Methods have access to the fields of the class. Notice in the Employee class
that the mailCheck() method prints out the name and address of the employee
being paid using the name and address fields of the class. Similarly, the com-
putePay() method accesses the salary field.
What I want you to notice about this Employee class is the following:
The name of the class is Employee.
■■
The class has five public fields.
■■
The class has two public methods.
■■
The Employee class needs to appear in a file named Employee.java, and the
compiled bytecode will appear in a file named Employee.class.
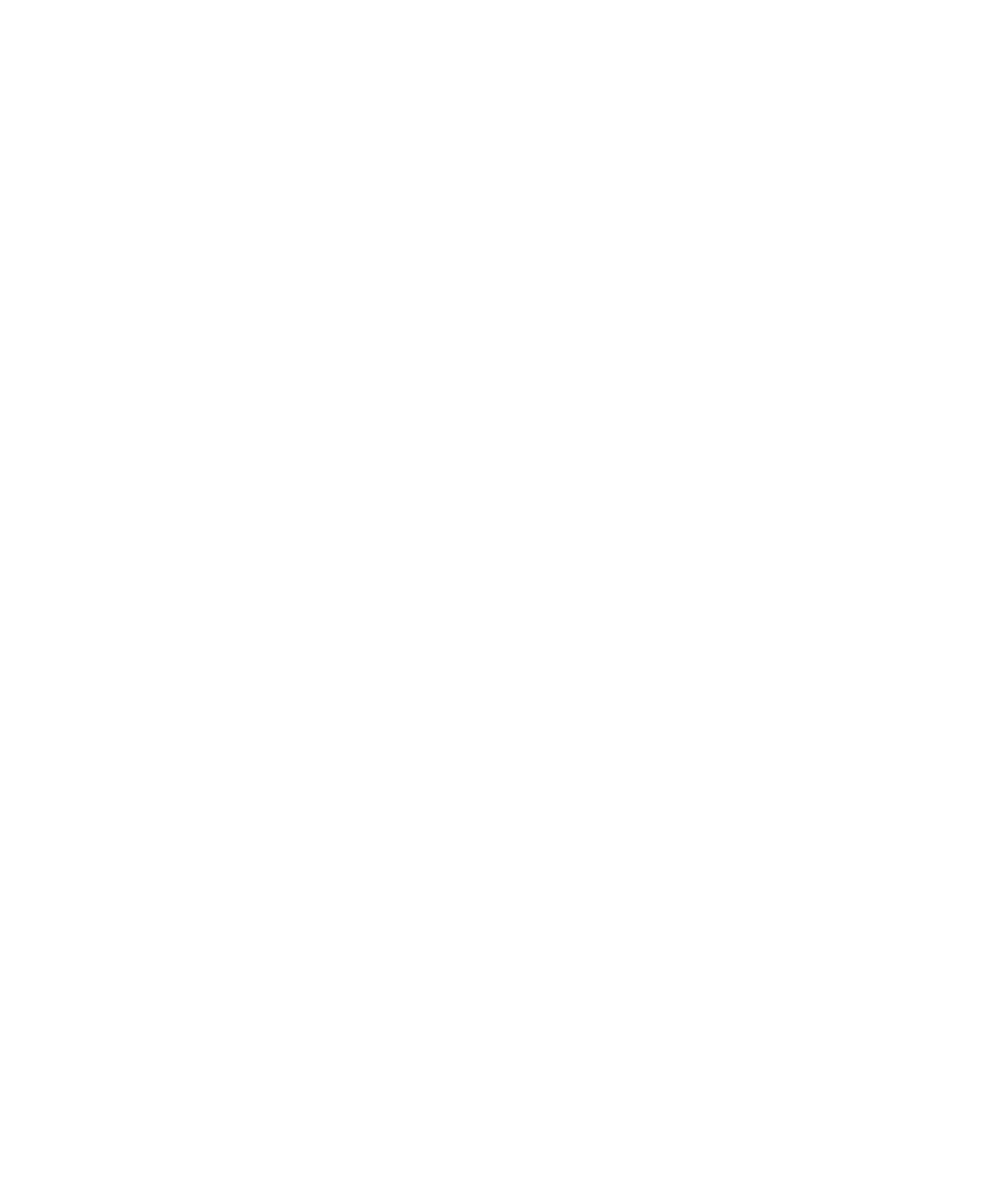