Java Reference
In-Depth Information
After you have determined the objects in the problem, you write a class to
describe the attributes and behaviors of each object. For example, we will need
an Employee class that contains the attributes and behaviors of an employee.
The attributes of an Employee object will be what the employee “has,” such
as a name, address, employee number, Social Security number, and so on. Each
of these attributes will be represented by a field in the Employee class.
The behaviors of an Employee object are what the employee object “does”
(or, more specifically, what we want the object to do). Employees do many
things, but for our purposes, we want to be able to compute their pay and mail
them a paycheck once a week. These desired behaviors become
methods
in the
Employee class.
For each employee in the company, we would instantiate an Employee object.
If we have 50 employees, we need 50 Employee objects. In memory, this would
create 50 names, addresses, salaries, and so on. Each employee would be distin-
guished by a reference, so we would need 50 references as well. Later in this
chapter, you will see how to instantiate an object and assign a reference to it.
With object-oriented programming, data is still passed around between
method calls as in procedural programs. However, there is an important
distinction to be made when comparing procedural programming with
object-oriented programming. The data that is passed around in an object-
oriented program is typically varying data, such as the number of hours an
employee has worked in a week. It is not the entire Employee object that
gets passed around.
If a procedure in a procedural program needs data to perform a task,
the necessary data is passed in to the procedure. With object-oriented
programming, the object performs the task for you, and the method can
access the necessary data without having to pass it in to the method.
For example, if you want to compute an employee's pay, you do not pass
the corresponding Employee object to a computePay() method. Instead,
you invoke the computePay() method on the desired Employee object.
Because it is a part of the Employee object, the computePay() method has
access to all the fields in the Employee object, including the Employee
object's hourly pay, salary, and any other required data.
Object-Oriented Analysis and Design
Programs written with an object-oriented language revolve around the objects
in the problem domain, not the individual tasks that need to be performed in
solving the problem. How you decide what the objects are and what the objects
look like is an important but unique process.

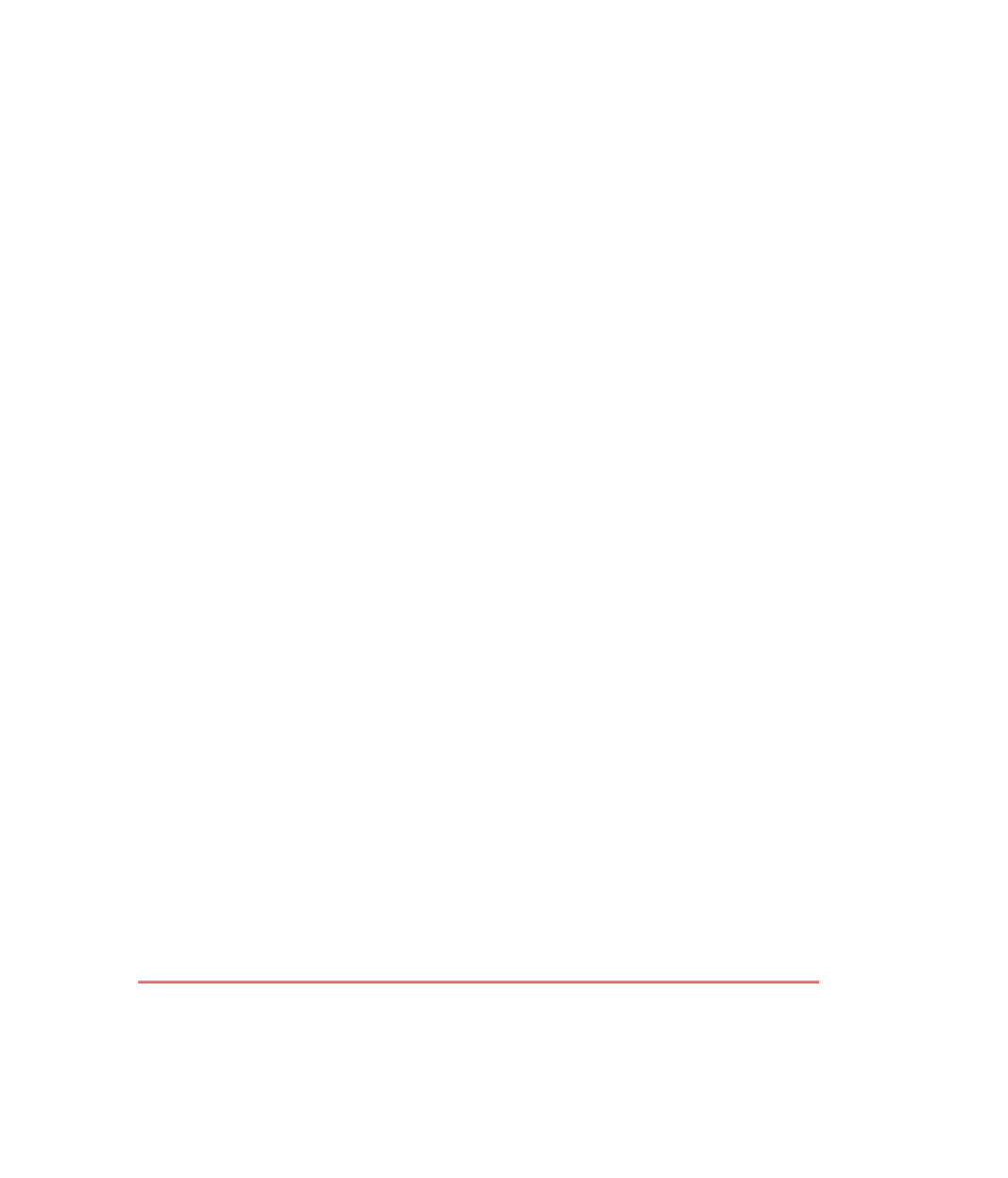
