Java Reference
In-Depth Information
Nested Loops
A
nested
loop is a loop that appears within the body of another loop. For exam-
ple, within the body of a for loop, you can have another for loop. The follow-
ing Alphabet program demonstrates nested loops by displaying the letters of
the alphabet in rows and columns. Study the program, and try to determine
the output, which is shown in Figure 3.8.
public class Alphabet
{
public static void main(String [] args)
{
char current = 'a';
for(int row = 1; row <= 3; row++)
{
for(int column = 1; column <= 10; column++)
{
System.out.print(current + “ “);
current++;
}
System.out.println();
}
}
}
The outer for loop executes three times, and the inner for loop executes 10
times. That means the print() statement that displays the current variable is
going to execute 3
×
10 = 30 times. Ten characters are printed in a row, and
there are three rows.
Figure 3.8
The output of the Alphabet program.
Lab 3.1: Cell Phone Bill
This lab shows you how to write a Java program that computes a cus-
tomer's monthly cell phone bill given the number of minutes used.
You have written several Java programs in the labs in the previous two
chapters, so I am going to give you more freedom in writing the solutions

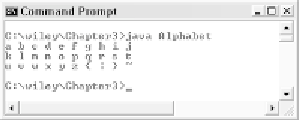


