Java Reference
In-Depth Information
The output of the previous statements is
one two three
Notice that the invocation of
toArray
requires the generic type to be specifi ed so
that the
toArray
method knows what type of array to create and return. The syntax
list.<String>toArray
lets the
toArray
method know to return an array of
String
objects.
Sorting lists is a common task in programming, and the
Collections.sort
methods
implement an effi cient sorting algorithm that offers
n
log(
n
) performance, where
n
is the
number of elements in the list. The next section discusses another common programming
task: searching a list.
Searching Lists
The
Collections
class contains two methods for searching the elements in a
List
:
public static <T> int binarySearch(List<? extends Comparable<? super T>>
list, T key)
searches the given
List
for the specified object. The list must be sorted
first and the elements in the list must implement
Comparable
.
public static <T> int binarySearch(List<? extends T> list, T key,
Comparator<? super T> c)
searches the given
List
for the specified object. The
List
must be sorted first, and the
c
parameter represents the
Comparator
object used to sort
the list.
Both
binarySearch
methods require the given list to be sorted prior to searching. If the
list is not sorted, the result of the search is undefi ned. The return value of both methods
is the index in the list where the object was found or negative if the given object does not
appear in the list.
Let's look at an example. The following list contains 20
Integer
objects with random
values:
6. List<Integer> list = new ArrayList<Integer>();
7. for(int i = 1; i <= 20; i++) {
8. int x = (int) (Math.random() * 10);
9. list.add(x);
10. }
Before it can be searched, the list must be sorted. The following statements sort the list
and then search for the number
5
:
11. Collections.sort(list);
12. for(Integer i : list) {
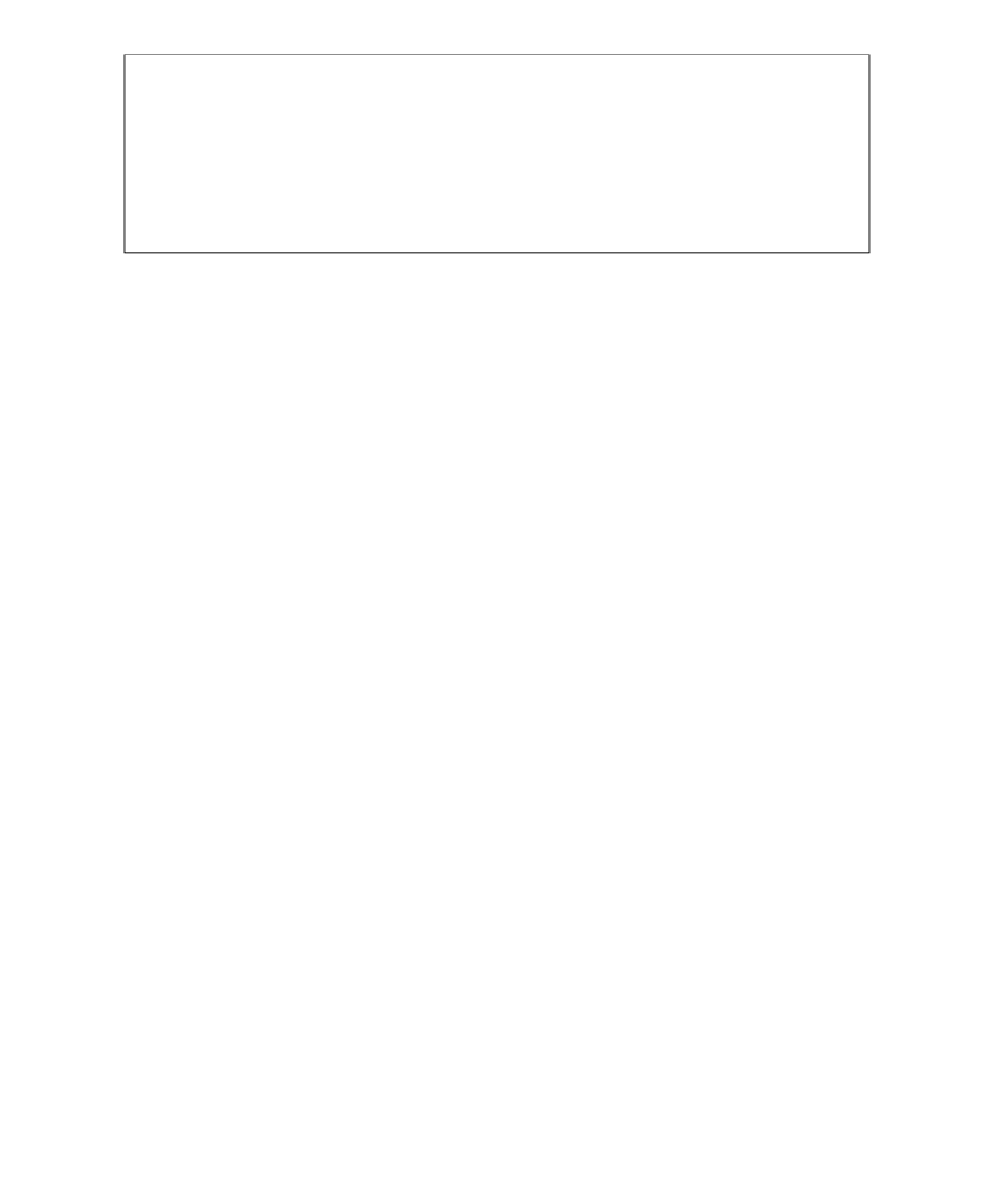




Search WWH ::

Custom Search