Java Reference
In-Depth Information
Product other = (Product) obj;
return this.id == other.id;
}
public int hashCode() {
return id;
}
public String toString() {
return id + “ “ + description;
}
}
Let's add some
Product
objects to a set. Using generics, the following statements create
a
HashSet
for
Product
objects. Study the code carefully and see if you can determine its
result:
7. Product one = new Product(“Laptop”, 1299.99, 101);
8. Product two = new Product(“Television”, 1099.00, 202);
9. Product three = new Product(“Cellphone”, 200.00, 303);
10. Product four = new Product(“PC”, 699.99, 101);
11. Set<Product> set = new HashSet<Product>();
12. set.add(one);
13. set.add(two);
14. set.add(three);
15. set.add(four);
16. set.add(null);
17. set.add(null);
18. for(Product p : set) {
19. System.out.println(p);
20. }
The four
Product
objects are added to
set
using the
add
method of
Set
. Notice that the
objects
one
and
four
have the same
id
and are therefore equal, so adding
four
on line 15
does not modify the set. A
HashSet
allows a null entry, but only once. Adding
null
on line
16 modifi es the set, while line 17 does not. Sets are not ordered, so the elements of the set
are displayed in the
for-each
loop in no particular order. The output of the code is
null
101 Laptop
202 Television
303 Cellphone
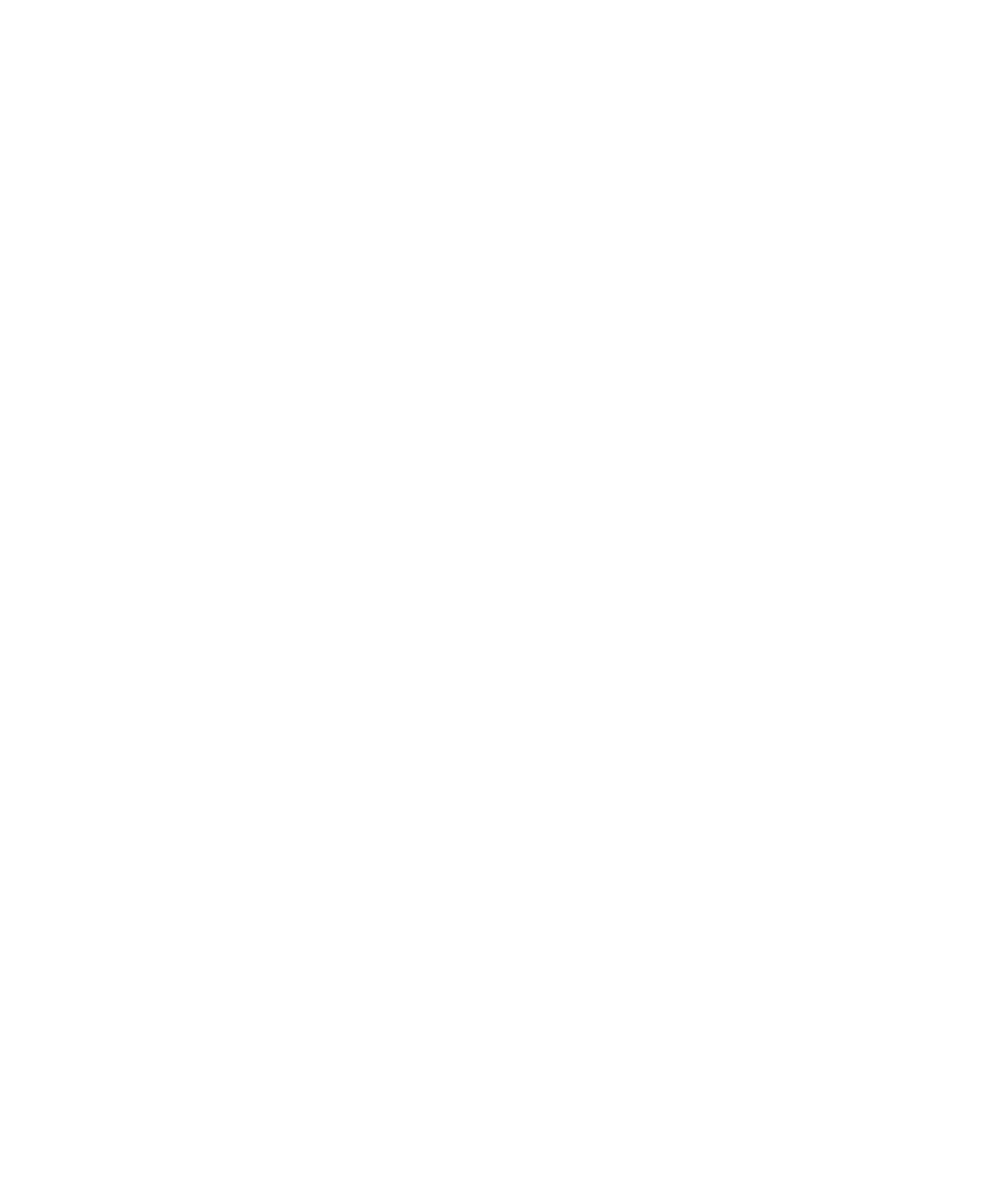




Search WWH ::

Custom Search