Java Reference
In-Depth Information
FIGURE 7.5
The
Map
interfaces and implementing classes
interface
Map
class
HashMap
interface
SortedMap
class
LinkedHashMap
interface
NavigableMap
class
TreeMap
The following overview shows the implementing classes of
Map
:
HashMap
A map that stores its elements in a hash table. There is no ordering to the
elements, and they are placed in the hash table based on their
hashCode
.
LinkedHashMap
A map that stores its element in a hash table and doubly linked lists. The
linked list provides an ordering to the elements.
TreeMap
A map that stores its elements in a tree data structure with a natural or
user-defi ned ordering.
TreeMap
provides log(
n
) time for the methods that view or change
elements in the tree.
The
Map
interface provides methods for accessing the elements of the collection as a set
of keys, a list of values, or a set of key-value mappings. We discuss maps in detail in the
section “Using Generics” later in this chapter as well as the details for using lists, sets, and
queues. But before we move on to generics, I need to discuss one more topic on the subject
of collections: the
Comparable
interface.
Using a
Map
to Count Keywords
A common use for search engines is to determine the relevance of the content on a web
page, and an important factor that search engines use is the number of times a particular
word or phrase appears on the page. This scenario is a good example of when to use a
Map
object, where the key of the map is the keyword on the web page and the value of the
map is the number of occurrences. The code might look something like the following:
import java.util.HashMap;
public class KeywordCounter {
public HashMap<String, Integer> keywords = new HashMap<String, Integer>();
public void keywordFound(String keyword) {
Integer count = keywords.get(keyword);







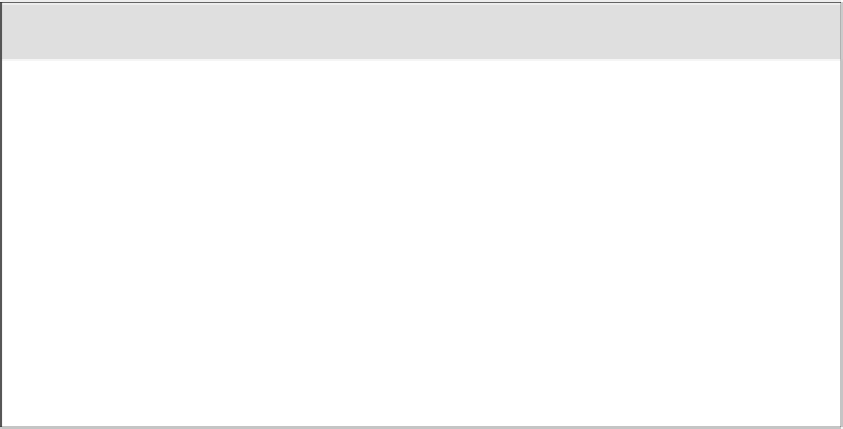






Search WWH ::

Custom Search