Java Reference
In-Depth Information
private static final File DEST;
static {
DEST = new File(“mylogfile.txt”);
}
public final static void logMessage(final String MESSAGE) {
final long TIME = new java.util.Date().getTime();
//write time and message to file...
}
}
The following
MyNewLogger
class extends
MyStaticLogger
and attempts to override
logMessage
:
public class MyNewLogger extends MyStaticLogger {
public static void logMessage(final String MESSAGE) {
System.out.println(“Using MyNewLogger...”);
//write message to DEST file
}
}
The compiler generates the following error:
MyNewLogger.java:2: logMessage(java.lang.String) in MyNewLogger cannot
override logMessage(java.lang.String) in MyStaticLogger; overridden
method is static final
public static void logMessage(final String MESSAGE) {
^
1 error
Recall from Chapter 2 that a
non-final static
method can be overridden in Java,
which is referred to as method hiding. For example, if
logMessage
in
MyStaticLogger
is not
declared
final
, then overriding
logMessage
in
MyNewLogger
would be valid.
Now that we have discussed the details of the various modifi ers, let's change subjects
and discuss the object-oriented concept of polymorphism and how to use polymorphism in
your Java applications.
Polymorphism
Polymorphism refers to how an object in Java can take on “many forms.” Be prepared to
develop code that demonstrates the use of polymorphism.
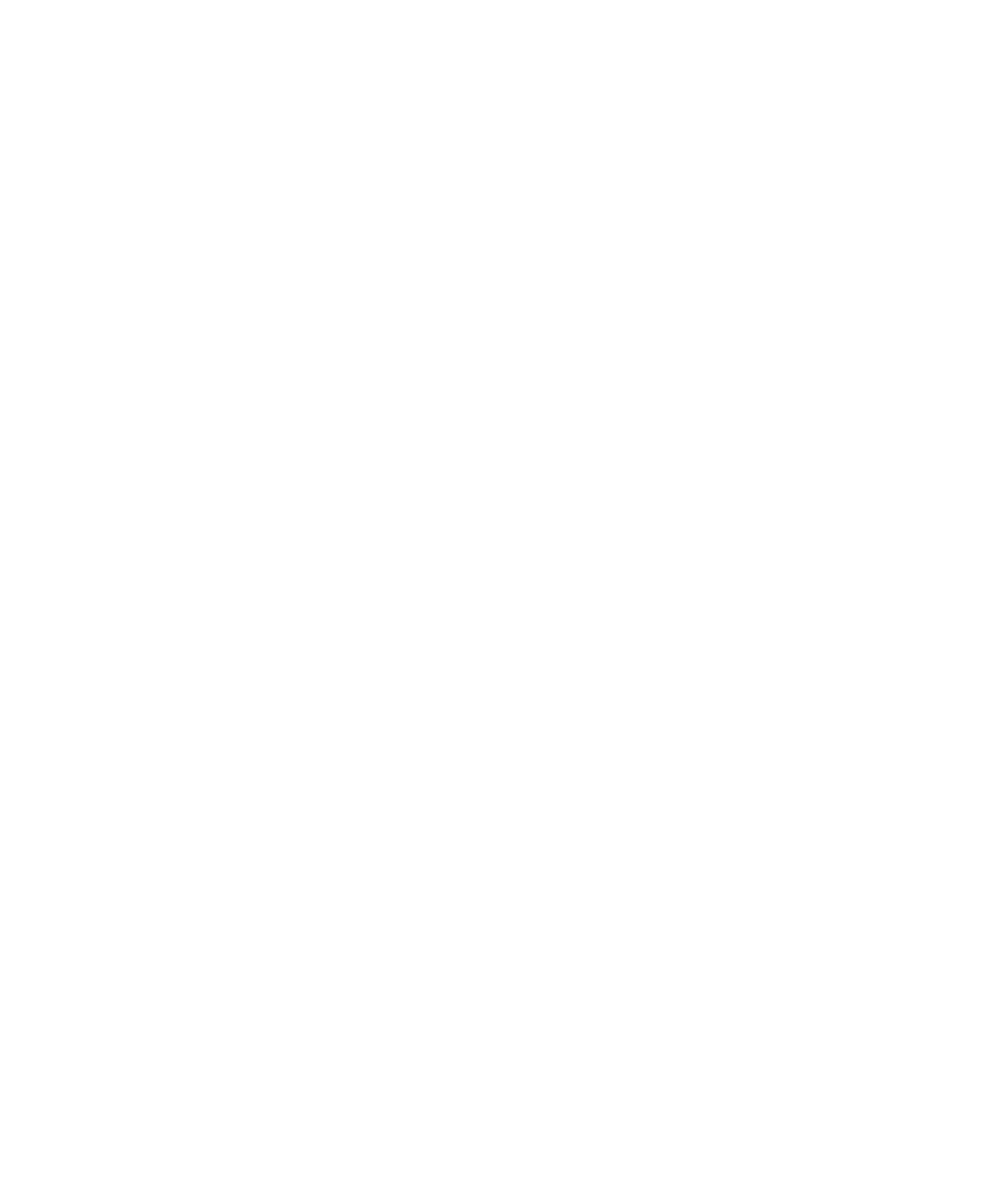




Search WWH ::

Custom Search