Java Reference
In-Depth Information
Threads that have the same priority execute in a round-robin fashion, meaning that the
currently executing thread runs until it either terminates or transitions into a waiting state.
Use the static
yield
or
sleep
method of the
Thread
class if you are concerned a thread is
hogging the CPU. The
sleep
method causes a thread to transition into the
TIMED_WAITING
state until the specifi ed amount of time elapses. The
yield
method causes the currently
running thread to give up the CPU, allowing another thread to be scheduled. Figure 5.3
shows the transitions for
sleep
and
yield
.
FIGURE 5.3
The thread state transition of the sleep and yield methods
TIMED_WAITING
timeout
sleep()
yield()
RUNNABLE
CPU
scheduled
Notice that invoking
yield
does not change the state of a thread; it just pushes the
thread to the back of the line of other runnable threads. The
sleep
method actually
transitions a thread from
RUNNABLE
to
TIMED_WAITING
. When the specifi ed time elapses, the
thread becomes
RUNNABLE
and goes to the back of the line of runnable threads.
To demonstrate the
yield
method, I added a call to
yield
in the
run
method of the
SayHello
class from the previous section:
public void run() {
for(int i = 1; i <= 10; i++) {
System.out.print(greeting);
Thread.yield();
}
System.out.println(“End of run”);
}
Similarly, I added a call to
yield
in the
CountToTen
program, which now yields after
printing each
int
:
for(int k = 1; k <= 10; k++) {
System.out.print(k);
Thread.yield();
}
Running the program again results in an entirely different output than before the yields
were added:
1Hi2Hi3Hi4Hi5Hi6Hi7Hi8Hi9Hi10HiEnd of main
End of run

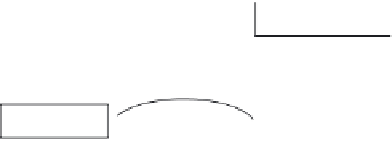




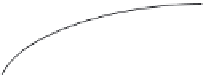






Search WWH ::

Custom Search