Java Reference
In-Depth Information
Line 3 instantiates a new
MyThread
object with the message
“going”
. Because
MyThread
extends the
Thread
class, it inherits the
start
method of
Thread
, so line 4 starts the new
MyThread
object. The main thread then sleeps on line 6 for six seconds. While the main
thread is sleeping,
MyThread
is printing
“going”
about every second. Upon awaking and
getting scheduled by the JVM, line 9 sets the
keepGoing
fi eld of
MyThread
to
false
. The
output of
Main
looks like
going going going going going going End of main
gone!
As I mentioned in the previous section, the output of a multithreaded application is
indeterminate. The above output of
Main
is a common output because of the timing of the
calls to
sleep
.
MyThread
prints
“going”
every second and
Main
sleeps for six seconds, then
tells
MyThread
to stop printing, so I am not surprised that
“going”
is printed six times.
However, depending on the JVM and other factors of the environment, it is possible for
“going”
to be printed a different number of times.
Stopping a Thread
The
Thread
class contains a method named
stop
, but this method is deprecated and you
are highly discouraged from invoking it. The problem with the
stop
method is that the
thread being stopped might contain object locks that other threads are waiting for, and
the stopped threads might not let go of those locks when
stop
is called.
If you need one thread to be able to stop another thread, follow the design of the
MyThread
class in this section. Notice that the
while
loop on line 15 of
MyThread
checks
the
boolean
fi eld
keepGoing
after each printing of the message. The idea behind this
design is that another thread can communicate with a
MyThread
object by setting
keepGoing
to
false
(via the
setKeepGoing
method of
MyThread
). Setting the
boolean
to
false
won't stop the thread immediately, but the thread only does a small amount of
work before checking the
boolean
again. Providing a mechanism in your threads so that
they can be stopped cleanly by another thread is commonly done in multithreaded Java
applications.
Now that you have seen the details of writing a thread by implementing
Runnable
or
extending
Thread
, let's discuss the important topic of thread state and what happens to
your threads when they are not running on the CPU.

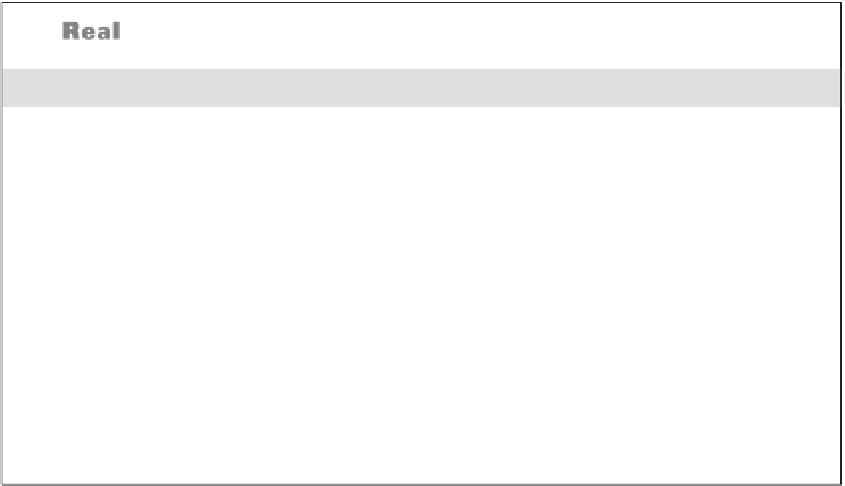


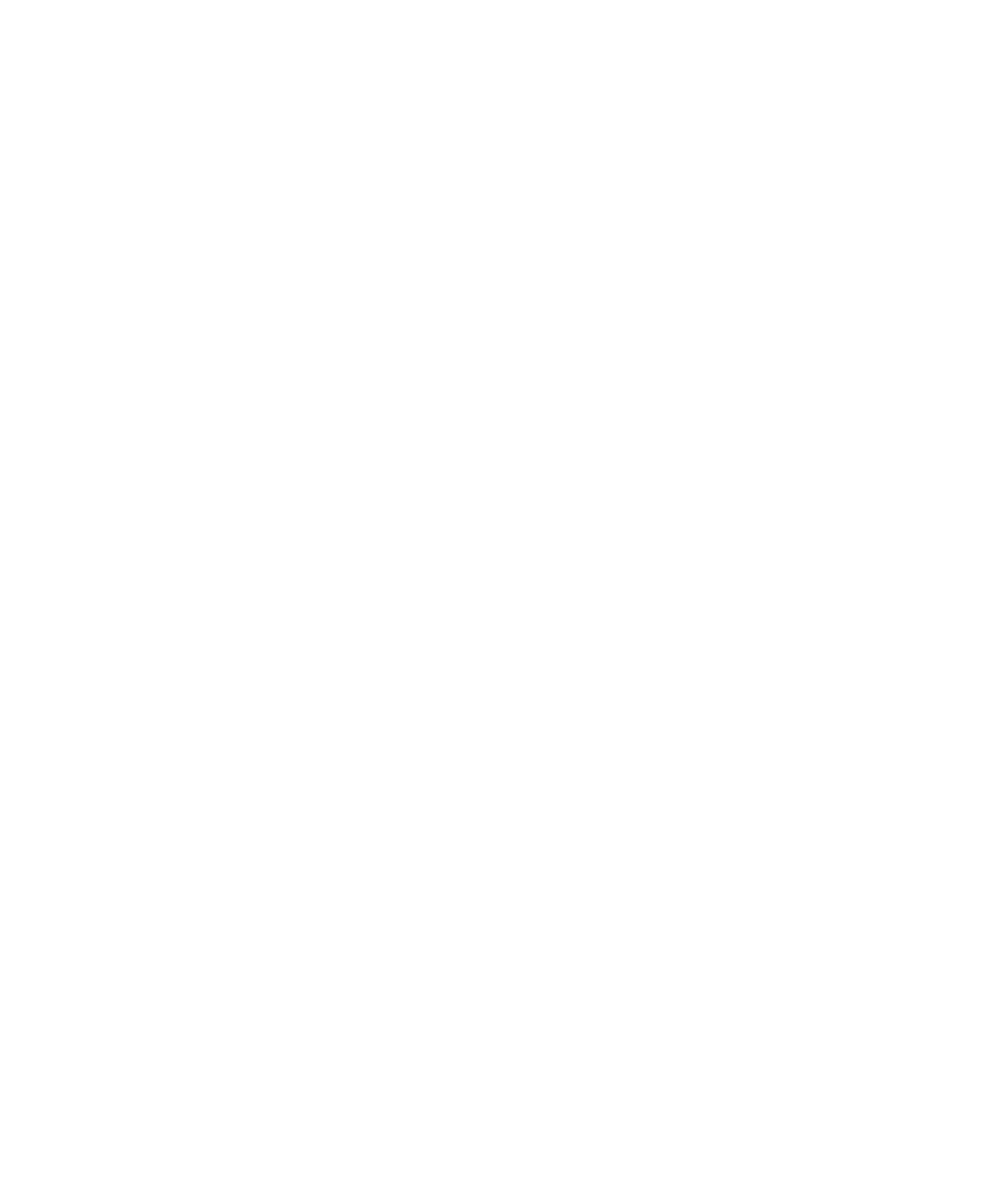




Search WWH ::

Custom Search