Java Reference
In-Depth Information
4.
5. public class FileDemo {
6. public static void main(String [] args) {
7. File test = new File(“./test.html”);
8. if(!test.exists()) {
9. try {
10. test.createNewFile();
11. }catch(IOException e) {
12. System.out.println(e.getMessage());
13. return;
14. }
15. }
16. try {
17. FileWriter fw = new FileWriter(test);
18. BufferedWriter bw = new BufferedWriter(fw, 1024);
19. PrintWriter out = new PrintWriter(fw);
20. out.println(“<html><body><h1>”);
21. out.println(args[0]);
22. out.println(“</h1></body></html>”);
23. out.close();
24. bw.close();
25. fw.close();
26. }catch(IOException e) {
27. e.printStackTrace();
28. }
29. }
30. }
The following sequence of events occurs when running the
FileDemo
program:
1.
Line 7 creates a new
File
object for the pathname
“./test.html”
. Keep in mind this
does not create a new file on your file system. The
File
class represents pathnames to
files and directories, not actual files and directories.
2.
Line 10 creates a new file named
test.html
on the file system if no such file already
exists in the current directory.
3.
Line 17 instantiates a new
FileWriter
object that opens the file and prepares it for
writing. If the file contained any existing data, that data is now lost. (If you need to
append to a file, use the
FileWriter
constructor that takes in an additional
boolean
argument.)
4.
Line 18 chains a
BufferedWriter
to the
FileWriter
with an initial buffer size of
1,024.
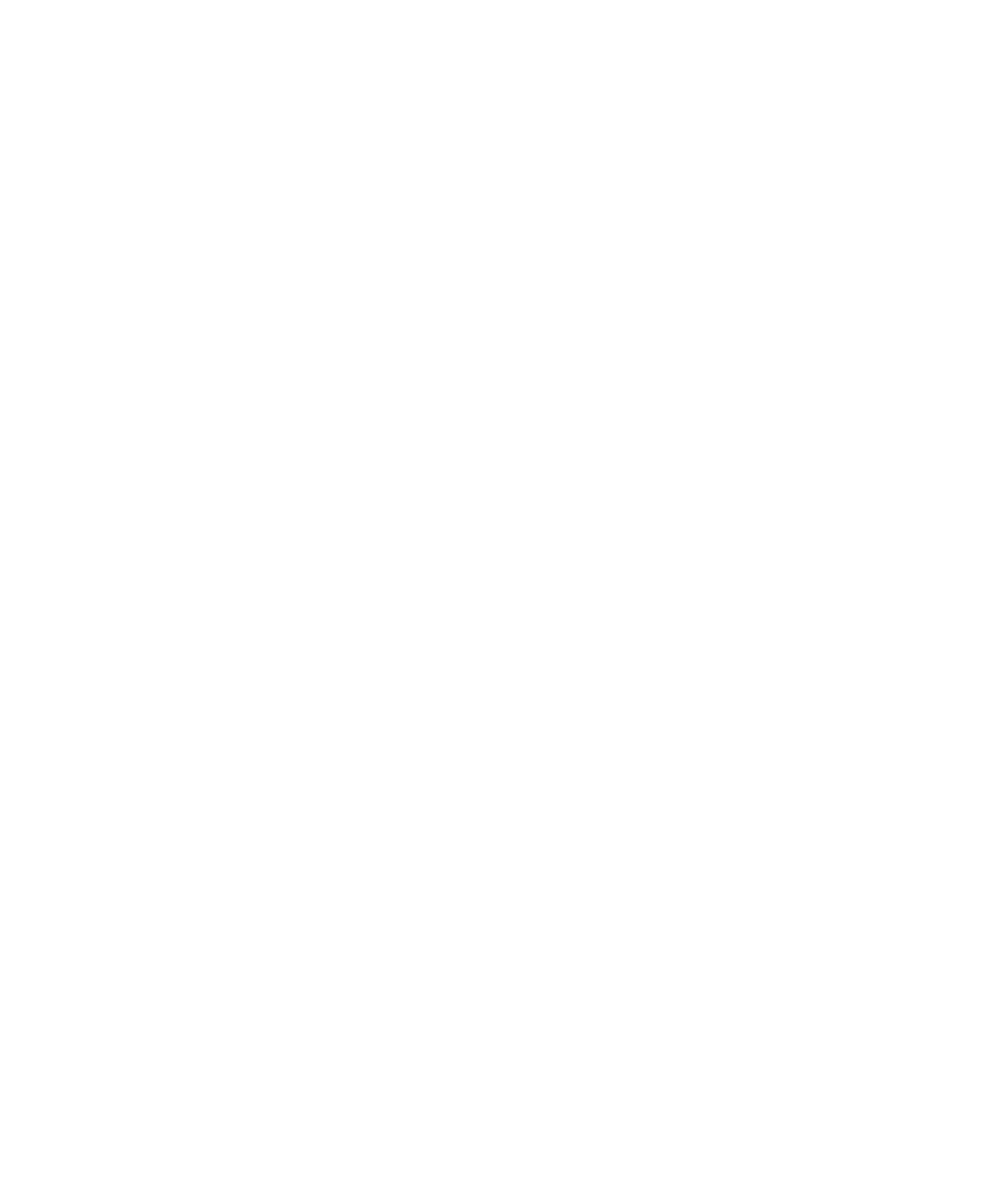




Search WWH ::

Custom Search