Java Reference
In-Depth Information
Each wrapper class has a “value” method that unwraps the primitive type. For
example, the
Float
class has a
floatValue
method that returns the
float
.
Let's look at an example. The following code wraps an
int
into an
Integer
object:
int x = 357;
Integer w = new Integer(x);
The
Integer
class has a method named
intValue
that unwraps the
int
:
int y = w.intValue();
Autoboxing
As of Java 5.0, primitive types can be automatically boxed and unboxed into their
corresponding wrapper classes, eliminating the need for instantiating a new wrapper
type to box a primitive, or using a value method to retrieve the wrapped primitive.
Let's look at an example where a wrapper class is necessary. The following class con-
tains a method named
addScore
with an
Object
parameter. Because a primitive type is not
an
Object
, a primitive type needs to be wrapped before it can be passed into
addScore
.
Study the following code and try to determine its output:
1. public class ScoreKeeper {
2. public java.util.ArrayList<Object> scores =
3. new java.util.ArrayList<Object>();
4.
5. public void addScore(Object score) {
6. scores.add(score);
7. }
8.
9. public void printScores() {
10. for(Object score : scores) {
11. System.out.println(score);
12. }
13. }
14.
15. public static void main(String [] args) {
16. ScoreKeeper keeper = new ScoreKeeper();
17. Integer one = new Integer(50);
18. Double two = new Double(23.4);
19. Float three = new Float(18.5);
20. keeper.addScore(one);

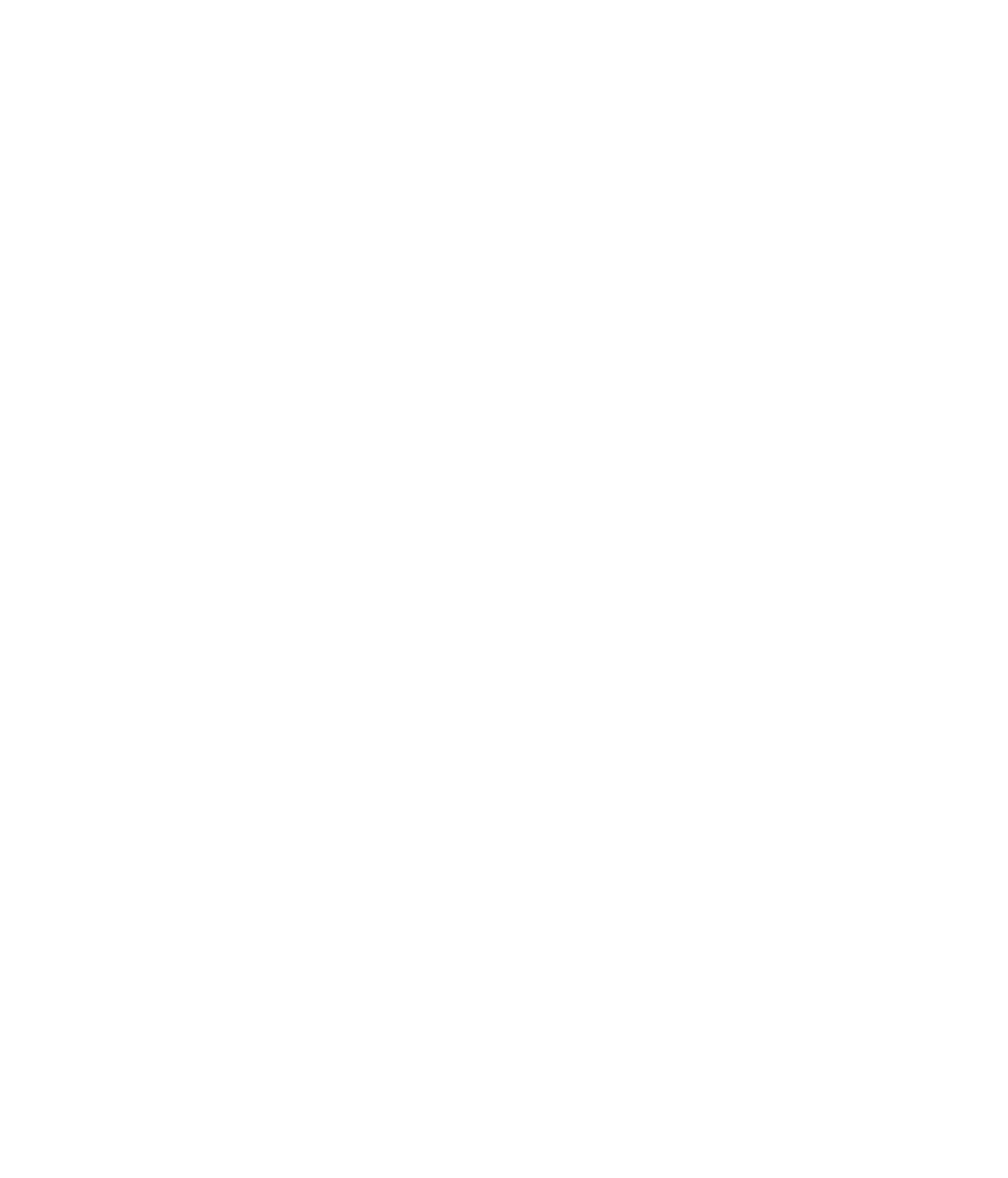




Search WWH ::

Custom Search