Java Reference
In-Depth Information
As with most errors in Java, you cannot recover from an
ExceptionInInitializerError
because there is no way to catch it.
StackOverflowError
A
StackOverflowError
is thrown by the JVM when the method call stack overfl ows,
typically due to method recursion that does not end. The following example causes a
StackOverflowError
due to its infi nite recursion:
1. public class StackOverflowDemo {
2. private int x = 0;
3.
4. public void go() {
5. System.out.println(++x);
6. go();
7. }
8. public static void main(String [] args) {
9. new StackOverflowDemo().go();
10. }
11. }
The
go
method invokes itself recursively on line 6, so eventually the method call stack
will consume all of its allotted memory, causing a
StackOverflowError
. Running the
previous program generates the following stack trace:
Exception in thread “main” java.lang.StackOverflowError
at sun.nio.cs.SingleByteEncoder.encodeArrayLoop(SingleByteEncoder.
java:91)
at sun.nio.cs.SingleByteEncoder.encodeLoop(SingleByteEncoder.java:130)
at java.nio.charset.CharsetEncoder.encode(CharsetEncoder.java:544)
at sun.nio.cs.StreamEncoder.implWrite(StreamEncoder.java:252)
at sun.nio.cs.StreamEncoder.write(StreamEncoder.java:106)
at java.io.OutputStreamWriter.write(OutputStreamWriter.java:190)
at java.io.BufferedWriter.flushBuffer(BufferedWriter.java:111)
at java.io.PrintStream.newLine(PrintStream.java:495)
at java.io.PrintStream.println(PrintStream.java:687)
at StackOverflowDemo.go(StackOverflowDemo.java:5)
at StackOverflowDemo.go(StackOverflowDemo.java:6)
at StackOverflowDemo.go(StackOverflowDemo.java:6)
The output actually displays the last line (the call to
go
on line 6) in the stack trace for
each time the method is invoked, which is several thousand calls to
go
. You can catch a
StackOverflowError
and recover from it, but that would be an unusual situation because
the error is typically avoided by using proper code.
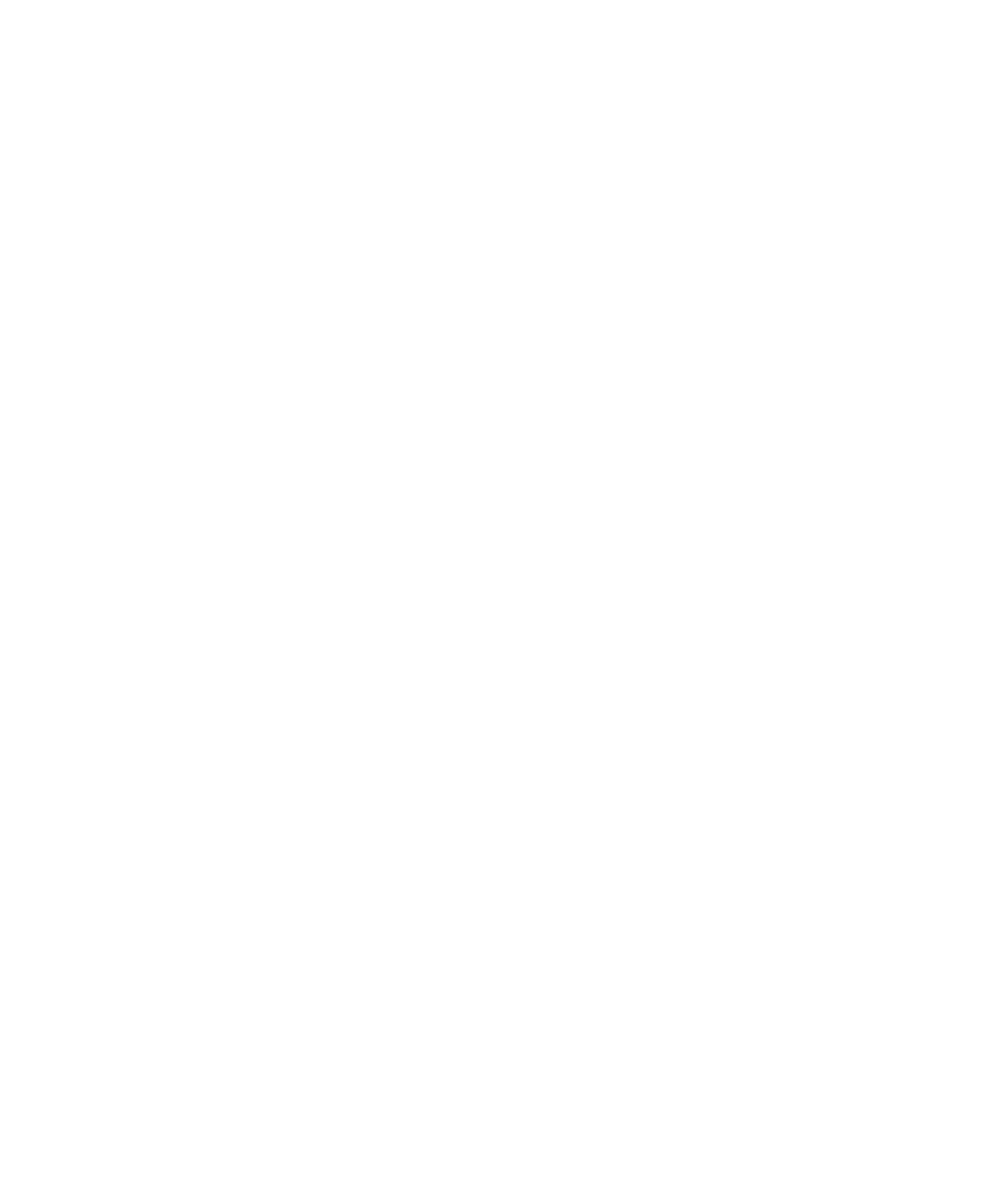




Search WWH ::

Custom Search