Java Reference
In-Depth Information
Why assert something if you are sure it is true? Well, in the world of computer
programming, asserting that something is true and verifying it at runtime are two different
things. During the coding phase, I might be certain that a value is positive, but it would be
nice to verify at runtime that the value actually is positive, and the assertion allows me to
do that.
In the next section, we discuss the details of writing and using assertions in Java and
how they affect the fl ow of control of your application.
The
assert
Statement
An
assert statement
inserts an assertion at a particular point in your code. The syntax for
an
assert
statement has two forms:
assert
boolean_expression
;
assert
boolean_expression
:
error_message
;
The
boolean
expression must evaluate to
true
or
false
. The optional error message
is a
String
used as the message for the
AssertionError
that is thrown. The two possible
outcomes of an
assert
statement are
If the
boolean
expression is
true
, then our assertion has been validated and nothing
happens. The program continues to execute in its normal manner.
If the
boolean
expression is
false
, then our assertion was invalid and a
java.lang
.AssertionError
is thrown, causing our program to terminate at this line of code.
The
AssertionError
is typically not handled by your code, so your program terminates
and the stack trace displays at the standard output. For example, the following assertion fails:
1. public class Asserts {
2. public static void main(String [] args) {
3. int x = 10;
4. assert x < 0;
5. System.out.println(“x = “ + x);
6. }
7. }
Because the
assert
statement on line 4 is false, line 5 does not execute. Assuming
assertions are enabled, the program terminates at line 4 and the following stack trace
displays:
Exception in thread “main” java.lang.AssertionError
at Asserts.main(Asserts.java:4)
The next section discusses how to enable assertions in your Java programs.
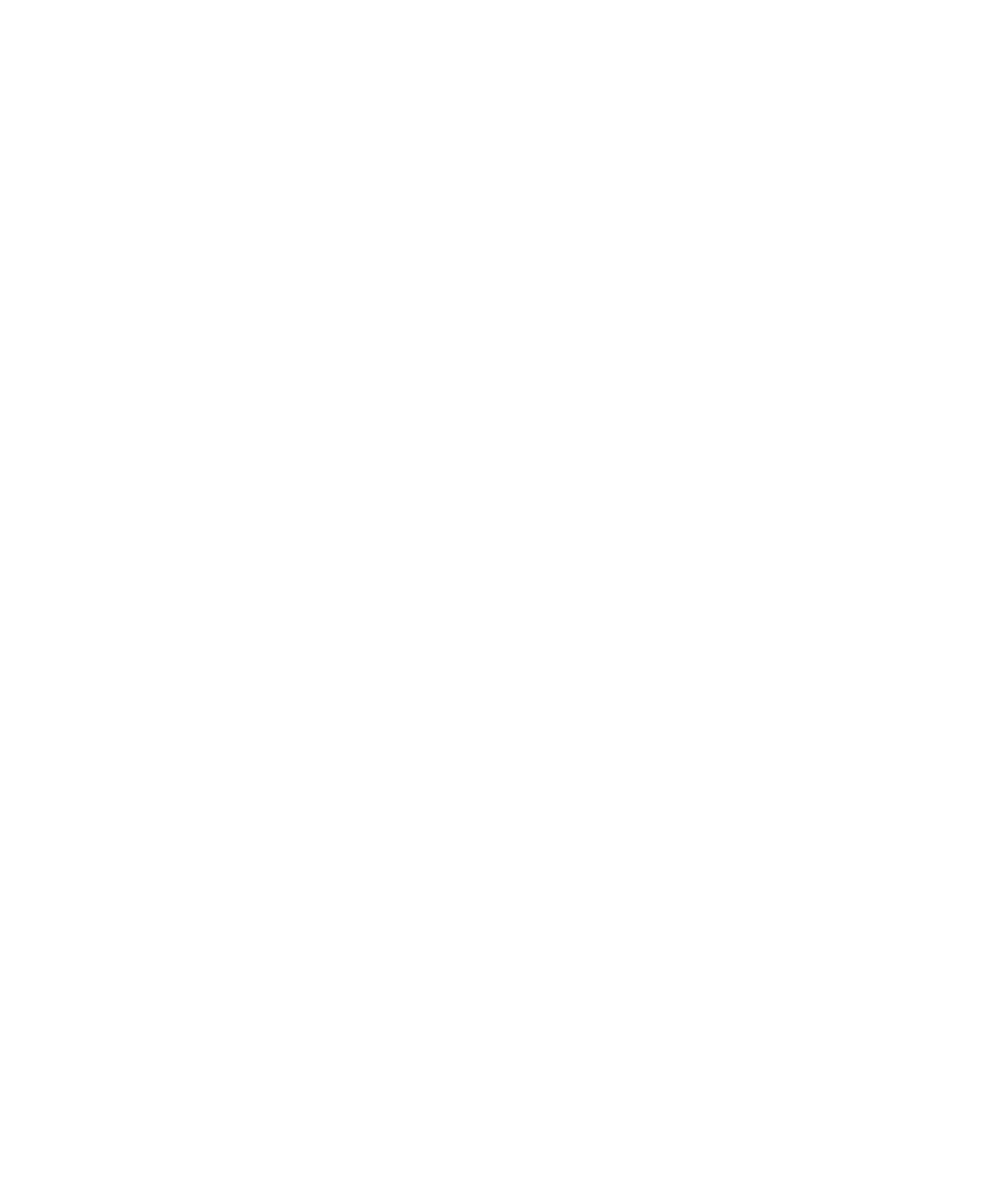




Search WWH ::

Custom Search